Created
September 7, 2017 10:04
-
-
Save undeadcat/7df6e0bcfd0f9efb4d9562a37db06a29 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
package com.intellij.StyledComponents | |
import com.intellij.lang.javascript.psi.JSFile | |
import com.intellij.psi.PsiElement | |
import com.intellij.psi.css.CssElementDescriptorProvider | |
import com.intellij.psi.css.CssPropertyDescriptor | |
import com.intellij.psi.css.CssSimpleSelector | |
import com.intellij.psi.impl.source.tree.injected.InjectedLanguageUtil | |
class MyCssElementDescriptorProvider : CssElementDescriptorProvider() { | |
override fun findPropertyDescriptors(propertyName: String, context: PsiElement?): MutableCollection<out CssPropertyDescriptor> { | |
val normalizedName = toCssName(propertyName) | |
return EP_NAME.extensions.last() | |
.findPropertyDescriptors(normalizedName, context) | |
.filter { it.browsers.isEmpty() } | |
.toMutableList() | |
} | |
override fun getDeclarationsForSimpleSelector(p0: CssSimpleSelector): Array<PsiElement> { | |
return emptyArray() | |
} | |
override fun shouldAskOtherProviders(context: PsiElement?): Boolean { | |
return false | |
} | |
override fun isMyContext(p0: PsiElement?): Boolean { | |
return p0 != null && InjectedLanguageUtil.getTopLevelFile(p0) is JSFile | |
} | |
override fun getAllPropertyDescriptors(context: PsiElement?): MutableCollection<out CssPropertyDescriptor> { | |
return EP_NAME.extensions.last() | |
.getAllPropertyDescriptors(context) | |
.filter { it.browsers.isEmpty() } | |
.map { MyPropertyDescriptor(it, toReactNativeName(it.propertyName)) } | |
.toMutableList() | |
} | |
private fun toReactNativeName(propertyName: String): String { | |
return propertyName.foldIndexed(StringBuilder(), { index, acc, c -> | |
if (c == '-') { | |
} else if (index > 0 && propertyName[index - 1] == '-') { | |
acc.append(c.toUpperCase()) | |
} else { | |
acc.append(c) | |
} | |
acc | |
}).toString() | |
} | |
private fun toCssName(propertyName: String): String { | |
return propertyName.fold(StringBuilder(), { acc, c -> | |
if (!acc.isEmpty() && c.isUpperCase()) { | |
acc.append("-") | |
acc.append(c.toLowerCase()) | |
} else { | |
acc.append(c) | |
} | |
acc | |
}).toString() | |
} | |
class MyPropertyDescriptor(b: CssPropertyDescriptor, private val reactName: String) | |
: CssPropertyDescriptor by b { | |
override fun getPropertyName(): String { | |
return reactName | |
} | |
override fun getPresentableName(): String { | |
return reactName | |
} | |
override fun getDescription(): String { | |
return reactName | |
} | |
override fun getId(): String { | |
return reactName | |
} | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This is a CssElementDescriptorProvider that naively transforms css properties to 'react-native-specific props' in CSS files.
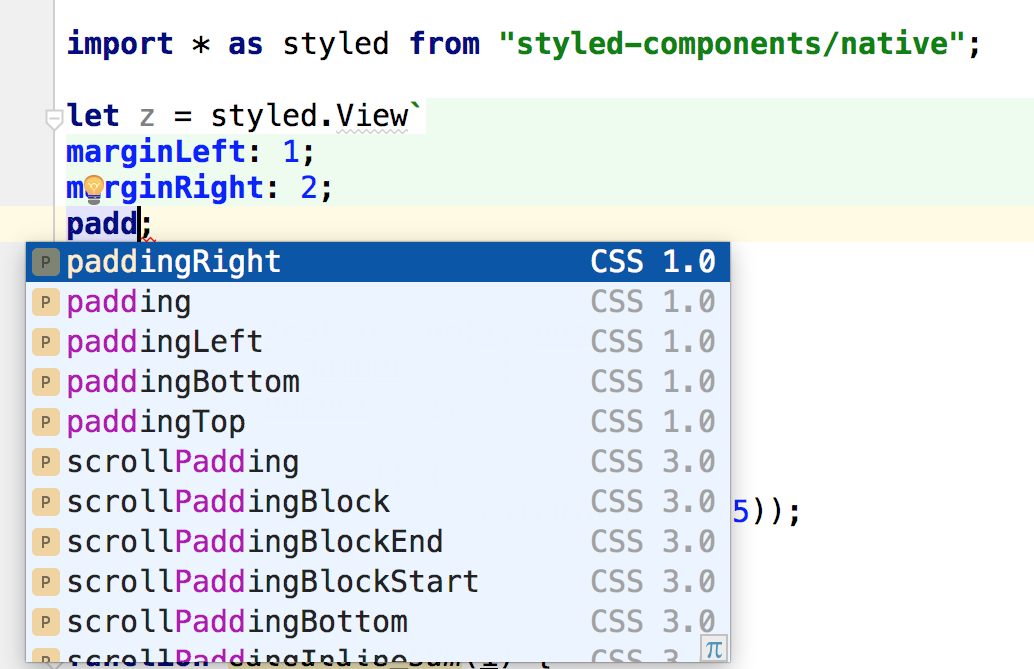
It does not add platform specific props or remove browser-specific properties or take into account difference in schema of some (like top)