Last active
September 25, 2021 17:28
-
-
Save unitycoder/972545b6395503bbd90528585588b5c1 to your computer and use it in GitHub Desktop.
Unity Shader Properties
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Shader "Name" | |
{ | |
Properties | |
{ | |
_MyFloat("Float", Float) = 0 | |
_MyRange ("Range", Range (0.0,1.0)) = 0.5 | |
_Mycolor ("Color", Color) = (0.5, 0.0, 0.0, 1.0) | |
_MyInt ("Int", Int) = 1 | |
_MyColor ("Some Color", Color) = (1,1,1,1) | |
_MyVector ("Some Vector", Vector) = (0,0,0,0) | |
_MyRange ("My Range", Range (0, 1)) = 1 | |
_MyFloat ("My float", Float) = 0.5 | |
_MyInt ("My Int", int) = 1 | |
_MyTexture2D ("Texture2D", 2D) = "white" {} | |
_MyTexture3D ("Texture3D", 3D) = "white" {} | |
_MyCubemap ("Cubemap", CUBE) = "" {} | |
name ("display name", Vector) = (number,number,number,number) | |
_Tex ("Tex", 2D) = "white" {} | |
_Tex ("Tex", 2D) = "defaulttexture" {} | |
name ("display name", Cube) = "defaulttexture" {} | |
name ("display name", 3D) = "defaulttexture" {} | |
} | |
} | |
// get texture tiling and offset value from material: | |
float4 {TextureName}_ST | |
Materials often have Tiling and Offset fields for their texture properties. This information is passed into shaders in a float4 {TextureName}_ST property: | |
x contains X tiling value | |
y contains Y tiling value | |
z contains X offset value | |
w contains Y offset value | |
// get texture size in shader | |
{TextureName}_TexelSize - a float4 property contains texture size information: | |
x contains 1.0/width | |
y contains 1.0/height | |
z contains width | |
w contains height | |
[HideInInspector] - does not show the property value in the material inspector. | |
[NoScaleOffset] - material inspector will not show texture tiling/offset fields for texture properties with this attribute. | |
[Normal] - indicates that a texture property expects a normal-map. | |
[HDR] - indicates that a texture property expects a high-dynamic range (HDR) texture. | |
[Gamma] - indicates that a float/vector property is specified as sRGB value in the UI | |
(just like colors are), and possibly needs conversion according to color space used. See Properties in Shader Programs. | |
[PerRendererData] - indicates that a texture property will be coming from per-renderer data in the form of a MaterialPropertyBlock. Material inspector changes the texture slot UI for these properties. | |
https://docs.unity3d.com/Manual/SL-Properties.html | |
-------------------------------- | |
Rendering Order - Queue tag | |
You can determine in which order your objects are drawn using the Queue tag. A Shader | |
decides which render queue its objects belong to, this way any Transparent shaders make sure they are drawn after all opaque objects and so on. | |
There are four pre-defined render queues, but there can be more queues in between the predefined ones. The predefined queues are: | |
- Background - this render queue is rendered before any others. You’d typically use this for things that really need to be in the background. | |
- Geometry (default) - this is used for most objects. Opaque geometry uses this queue. | |
- AlphaTest - alpha tested geometry uses this queue. It’s a separate queue from Geometry one since it’s more efficient to render alpha-tested objects after all solid ones are drawn. | |
- Transparent - this render queue is rendered after Geometry and AlphaTest, in back-to-front order. Anything alpha-blended (i.e. shaders that don’t write to depth buffer) should go here (glass, particle effects). | |
- Overlay - this render queue is meant for overlay effects. Anything rendered last should go here (e.g. lens flares). | |
Tags { "Queue" = "Transparent" } | |
The 7 passes a Surface Shader can generate are: | |
ForwardBase | |
- Used for rendering the main directional light and ambient lighting and/or lightmaps for forward rendering. | |
ForwardAdd | |
- Used for rendering a point, spot light, or any shadow casting directional light beyond the "main" one for forward rendering. | |
Deferred | |
- Used for rendering to the gbuffers used by the deferred rendering path. Only used by opaque objects when rendering with the deferred rendering path. | |
PrepassBase | |
- Used for rendering gbuffers for the legacy deferred "prepass" rendering path. | |
PrepassFinal | |
- Used for final rendering for the legacy deferred "prepass" rendering path. | |
ShadowCaster | |
- Used for rendering shadow maps and the camera depth texture when using forward rendering. | |
Meta | |
- Used for rendering of lightmaps. |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
matrix values
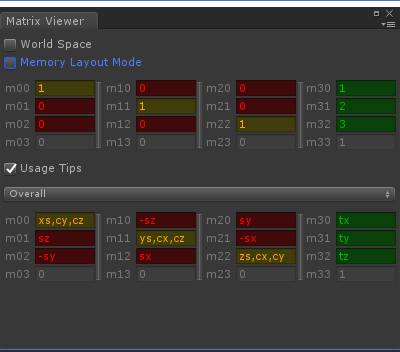
https://github.com/lujian101/UnityToolDist