Last active
October 25, 2022 08:53
-
-
Save v3rm0n/3922facb0e4267d284e7bf5d217420aa to your computer and use it in GitHub Desktop.
Bassdrive VLC Extension
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
local json = nil | |
local dayOrder = {'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday'} | |
function descriptor() | |
return { title = "Bassdrive Radio", capabilities = {} } | |
end | |
function indexOf(array, value) | |
for i, v in ipairs(array) do | |
if v == value then | |
return i | |
end | |
end | |
return nil | |
end | |
function daysort(a, b) | |
return indexOf(dayOrder, a) < indexOf(dayOrder, b) | |
end | |
function pairsByKeys (t, f) | |
local a = {} | |
for n in pairs(t) do table.insert(a, n) end | |
table.sort(a, f) | |
local i = 0 -- iterator variable | |
local iter = function () -- iterator function | |
i = i + 1 | |
if a[i] == nil then return nil | |
else return a[i], t[a[i]] | |
end | |
end | |
return iter | |
end | |
function main() | |
lazy_load() | |
local live = "https://bassdrive.radioca.st/stream"; --parsed['live']; Live URL doesn't change so hardcode it to make it load faster | |
vlc.sd.add_item({path=live, title='Live'}) | |
local parsed, _, err = parse_json('http://bd.maido.io/api.json') | |
if err ~= nil then | |
vlc.msg.err("Error to parse JSON response: " .. err) | |
return | |
end | |
for dayName, day in pairsByKeys(parsed['archive'], daysort) do | |
local dayNode = vlc.sd.add_node( {title=dayName} ) | |
for _, show in ipairs(day['shows']) do | |
local showNode = dayNode:add_subnode({title=show['name']}) | |
for _, episode in ipairs(show['episodes']) do | |
showNode:add_subitem({path=episode['encodedUrl'], title=episode['name']}) | |
end | |
end | |
end | |
end | |
function lazy_load() | |
if json ~= nil then return nil end | |
json = require("dkjson") | |
end | |
function parse_json(url) | |
local stream = vlc.stream(url) | |
local string = "" | |
local line = "" | |
if not stream then | |
return nil, nil, "Failed creating VLC stream" | |
end | |
while true do | |
line = stream:readline() | |
if not line then | |
break | |
end | |
string = string .. line | |
end | |
if string == "" then | |
return nil, nil, "Got empty response from server." | |
end | |
return json.decode(string) | |
end | |
function log(msg) | |
vlc.msg.dbg( "[BASSDRIVE] " .. msg ) | |
end |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Install it by creating a file in the VLC config directory subdirectory lua/sd called bassdrive.lua. Replace the content with this file and relaunch VLC, you should see the following:
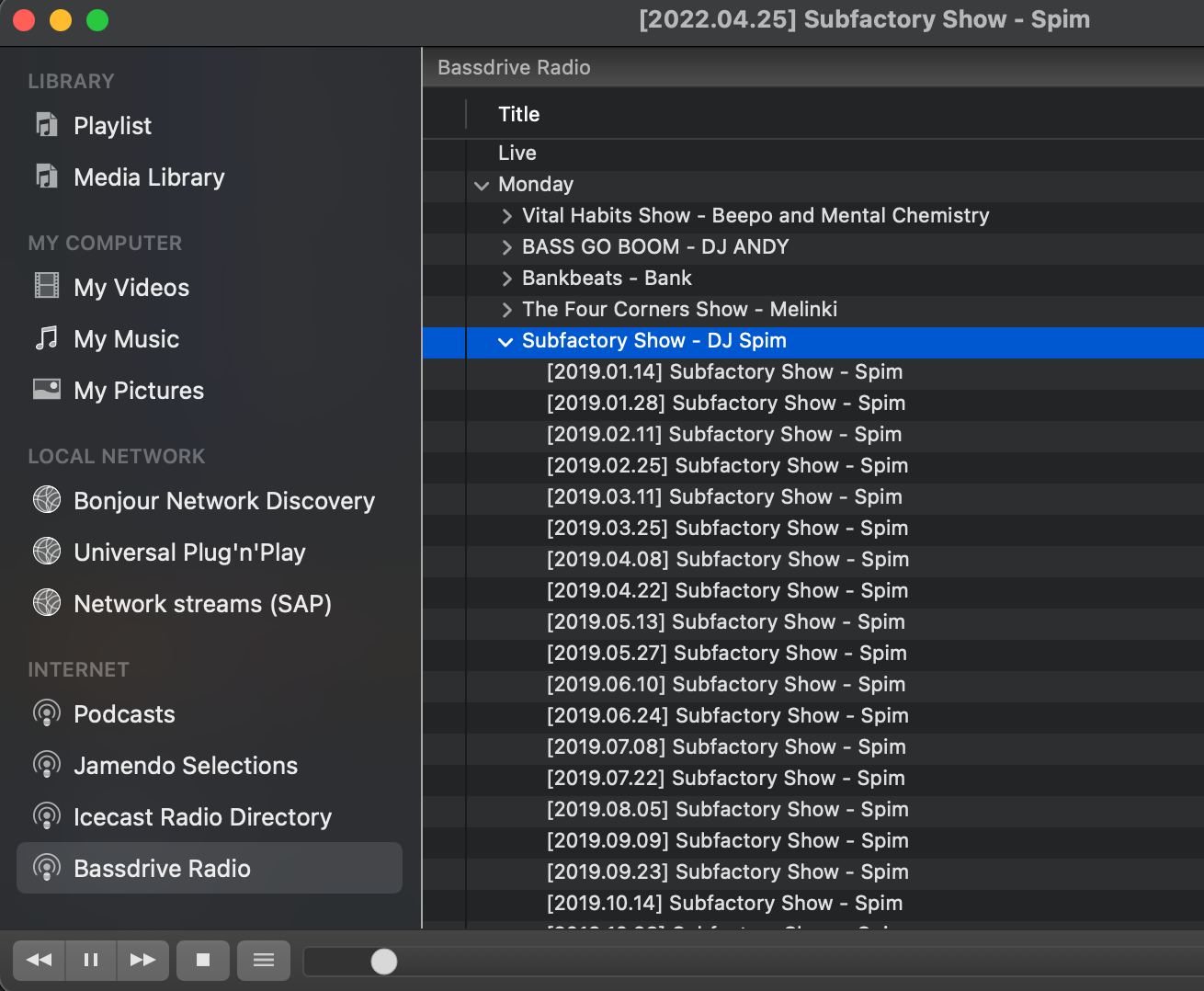