Last active
May 9, 2022 14:09
-
-
Save vdelacou/f5080c76f34a89e3ea8954ae03d64d27 to your computer and use it in GitHub Desktop.
React / Typescript / Material UI / Upload file / Custom Button / Async /
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// import ..... | |
const inputUploadFile: CSSProperties = { | |
display: 'none', | |
}; | |
const buttonUploadFile: CSSProperties = { | |
margin: 8, | |
}; | |
// component own props | |
interface UploadFileOwnProps { } | |
// component props | |
interface UploadFileProps extends UploadFileOwnProps { } | |
// component State | |
interface UploadFileStateProps { } | |
class UploadFileComponent extends Component<UploadFileProps, UploadFileStateProps> { | |
// function to read file as binary and return | |
private getFileFromInput(file: File): Promise<any> { | |
return new Promise(function (resolve, reject) { | |
const reader = new FileReader(); | |
reader.onerror = reject; | |
reader.onload = function () { resolve(reader.result); }; | |
reader.readAsBinaryString(file); // here the file can be read in different way Text, DataUrl, ArrayBuffer | |
}); | |
} | |
private manageUploadedFile(binary: String, file: File) { | |
// do what you need with your file (fetch POST, ect ....) | |
console.log(`The file size is ${binary.length}`); | |
console.log(`The file name is ${file.name}`); | |
} | |
private handleFileChange(event: ChangeEvent<HTMLInputElement>) { | |
event.persist(); | |
Array.from(event.target.files).forEach(file => { | |
this.getFileFromInput(file) | |
.then((binary) => { | |
this.manageUploadedFile(binary, file); | |
}).catch(function (reason) { | |
console.log(`Error during upload ${reason}`); | |
event.target.value = ''; // to allow upload of same file if error occurs | |
}); | |
}); | |
} | |
public render(): JSX.Element { | |
return ( | |
<Grid container style={grid}> | |
<Grid item xs={12}> | |
<input accept="image/*,.pdf,.doc,.docx,.xls,.xlsx" style={inputUploadFile} id="file" multiple={true} type="file" | |
onChange={this.handleFileChange} /> | |
<label htmlFor="file"> | |
<Button raised component="span" style={buttonUploadFile} onClick={e => e.stopPropagation()}> | |
Upload | |
</Button> | |
</label> | |
</Grid> | |
</Grid> | |
); | |
} | |
} |
test
- 1
- 2
- 3
- 4
_`[
- @
](url)`_
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
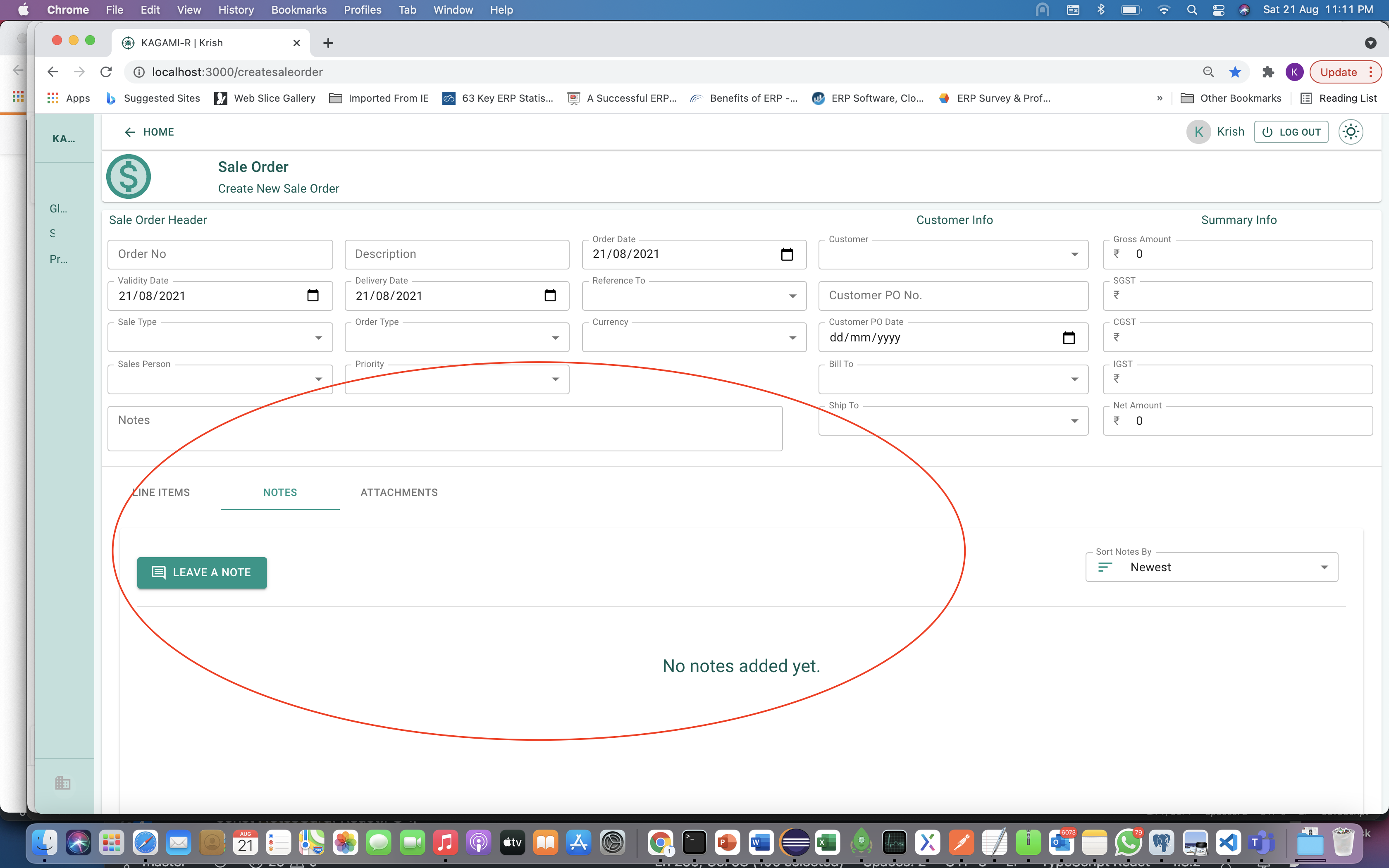
`[](url)`