Created
December 6, 2018 18:45
-
-
Save victorBaro/ff514a16d8db4b52857bdc400c6462c1 to your computer and use it in GitHub Desktop.
PaddingLabel is a subclass of UILabel that allows you to specify internal padding. Specially useful for labels with background color or/and border.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class PaddingLabel: UILabel { | |
var padding: UIEdgeInsets { | |
didSet { | |
invalidateIntrinsicContentSize() | |
} | |
} | |
public required init(padding: UIEdgeInsets = .zero) { | |
self.padding = padding | |
super.init(frame: CGRect.zero) | |
} | |
public override init(frame: CGRect) { | |
padding = .zero | |
super.init(frame: frame) | |
} | |
public required init?(coder aDecoder: NSCoder) { | |
padding = .zero | |
super.init(coder: aDecoder) | |
} | |
public override func drawText(in rect: CGRect) { | |
super.drawText(in: rect.inset(by: padding)) | |
} | |
public override var intrinsicContentSize: CGSize { | |
let superContentSize = super.intrinsicContentSize | |
let width = superContentSize.width + padding.left + padding.right | |
let heigth = superContentSize.height + padding.top + padding.bottom | |
return CGSize(width: width, height: heigth) | |
} | |
public override func sizeThatFits(_ size: CGSize) -> CGSize { | |
let superSizeThatFits = super.sizeThatFits(size) | |
let width = superSizeThatFits.width + padding.left + padding.right | |
let heigth = superSizeThatFits.height + padding.top + padding.bottom | |
return CGSize(width: width, height: heigth) | |
} | |
public override func textRect(forBounds bounds: CGRect, limitedToNumberOfLines numberOfLines: Int) -> CGRect { | |
let insetRect = bounds.inset(by: padding) | |
let textRect = super.textRect(forBounds: insetRect, limitedToNumberOfLines: numberOfLines) | |
let invertedInsets = UIEdgeInsets( | |
top: -padding.top, | |
left: -padding.left, | |
bottom: -padding.bottom, | |
right: -padding.right | |
) | |
return textRect.inset(by: invertedInsets) | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example:
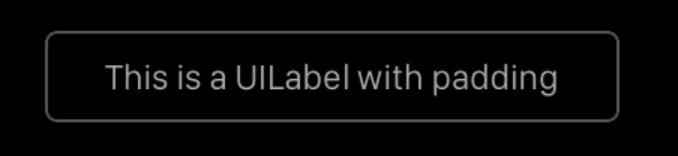