Created
October 5, 2019 16:40
-
-
Save wdevore/69029fde32d610e24968bdbbe5f6173b to your computer and use it in GitHub Desktop.
Simple counter used for learning test bench features and workflow
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// A simple comparator used for learning simulation workflows | |
// comparator - comparator_tb.v | |
// | |
// Description: | |
// Testbench for comparator module. | |
// | |
`timescale 1ns/10ps | |
module comparator #( | |
parameter WIDTH = 8 // data width | |
) ( | |
input [WIDTH-1:0] a, b, // values to compare | |
output lt, // high when a < b | |
output eq, // high when a = b | |
output gt // high when a > b | |
); | |
assign lt = (a < b) ? 1'b1 : 1'b0; | |
assign eq = (a == b) ? 1'b1 : 1'b0; | |
assign gt = (a > b) ? 1'b1 : 1'b0; | |
endmodule // comparator | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// -------------------------------------------------------------------------- | |
// Test bench | |
// -------------------------------------------------------------------------- | |
module comparator_tb; | |
parameter WIDTH = 2; // data width | |
integer i, j; // for-loop variables | |
reg [WIDTH-1:0] a, b; // input values to compare | |
wire lt, eq, gt; // output comparison status | |
initial begin | |
$dumpfile("comparator_tb.vcd"); // waveforms file | |
$dumpvars; // save waveforms | |
$display("%d %m: Starting testbench simulation...", $stime); | |
$monitor("%d %m: MONITOR - a = %d, b = %d, lt = %d, eq = %d, gt = %d.", $stime, a, b, lt, eq, gt); | |
#1; | |
for (i = 0; i < 2 ** WIDTH; i = i + 1) begin | |
for (j = 0; j < 2 ** WIDTH; j = j + 1) begin | |
#1 a = i; b = j; | |
#1; | |
if (a < b && (!lt || eq || gt)) begin | |
$display("%d %m: ERROR - Status flags lt (%d) eq (%d) gt (%d) are not correct for a (%d) less than b (%d).", $stime, lt, eq, gt, a, b); | |
$finish; | |
end | |
if (a == b && (lt || !eq || gt)) begin | |
$display("%d %m: ERROR - Status flags lt (%d) eq (%d) gt (%d) are not correct for a (%d) equal to b (%d).", $stime, lt, eq, gt, a, b); | |
$finish; | |
end | |
if (a > b && (lt || eq || !gt)) begin | |
$display("%d %m: ERROR - Status flags lt (%d) eq (%d) gt (%d) are not correct for a (%d) greater than b (%d). ", $stime, lt, eq, gt, a, b); | |
$finish; | |
end | |
end | |
end | |
#1 $display("%d %m: Testbench simulation PASSED.", $stime); | |
$finish; // end simulation | |
end | |
// Instances | |
comparator #(.WIDTH(WIDTH)) comparator_1(.a(a), .b(b), .lt(lt), .eq(eq), .gt(gt)); | |
endmodule // comparator_tb | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Make sure you have both files in the same folder otherwise you will need to add an include directive:
If you are using APIO then you can use the following command to simulate the counter:
You should get an output in your console:
APIO will also attempt to launch gtkwave if you have it installed:
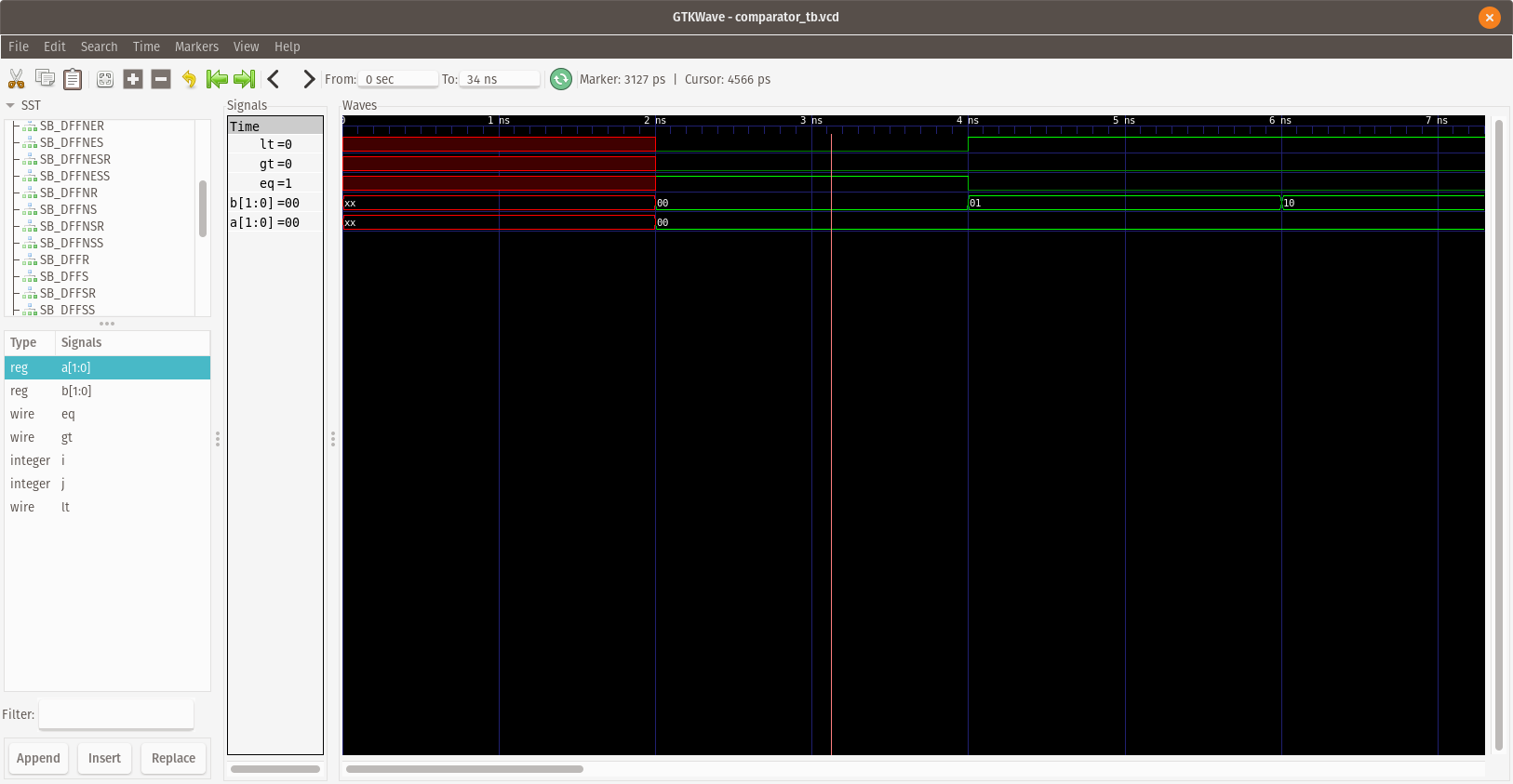