Created
September 7, 2019 23:41
-
-
Save wdevore/e0c57d99b508fd0e5eec4d15405649d6 to your computer and use it in GitHub Desktop.
Verilog cylon using Icestorm framework and targeting the tinyFPGA-B2 board. Properly uses a register and the shift operator.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// cylon effect - top.v | |
// | |
// pins are relative to the tinyFPGA-B2 | |
module top ( | |
output pin1_usb_dp,// USB pull-up enable, set low to disable | |
output pin2_usb_dn, | |
input pin3_clk_16mhz, // 16 MHz on-board clock | |
output pin13, | |
output pin12, | |
output pin11, | |
output pin10, | |
output pin9, | |
output pin8, | |
output pin7, | |
output pin6, | |
output pin5, | |
output pin4 | |
); | |
reg [22:0] clk_1hz_counter = 23'b0; // Hz clock generation counter | |
reg clk_1hz = 1'b0; // Hz clock | |
reg[9:0] shift; | |
reg direction = 0; // Initial direction is from pin4 to pin13 | |
reg[3:0] counter; | |
// 2 Hz clock | |
always @(posedge pin3_clk_16mhz) begin | |
if (clk_1hz_counter < 23'd7_999_999) | |
clk_1hz_counter <= clk_1hz_counter + 23'd16; | |
else begin | |
clk_1hz_counter <= 23'b0; | |
clk_1hz <= ~clk_1hz; | |
end | |
end | |
initial begin | |
shift[0] = 1; | |
counter = 0; | |
end | |
always @(posedge clk_1hz) begin | |
if (counter == 9) begin | |
direction = ~direction; | |
counter = 0; | |
end | |
if (direction == 0) begin | |
shift <= shift << 1; | |
end | |
else begin | |
shift <= shift >> 1; | |
end | |
counter <= #1 counter + 1; | |
end | |
assign pin13 = shift[9]; | |
assign pin12 = shift[8]; | |
assign pin11 = shift[7]; | |
assign pin10 = shift[6]; | |
assign pin9 = shift[5]; | |
assign pin8 = shift[4]; | |
assign pin7 = shift[3]; | |
assign pin6 = shift[2]; | |
assign pin5 = shift[1]; | |
assign pin4 = shift[0]; | |
assign pin1_usb_dp = 1'b0; | |
assign pin2_usb_dn = 1'b0; | |
endmodule // top |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
In action.
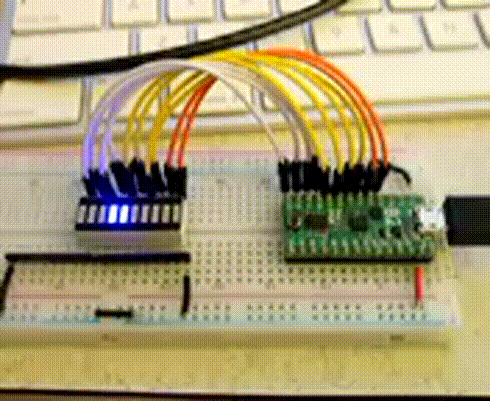