-
-
Save whoisryosuke/d034d3eaa0556e86349fb2634788a7a1 to your computer and use it in GitHub Desktop.
I couldn't use the
getServerSideProps
method in a HOC, because it is only available on pages. Neither usegetInitialProps
inside agetServerSideProps
, I got a warning in my console.
In my case. I needed a generic function to validate the user's access to a private page with an async call. I solved this by creating a function that receives the context and a callback.//util.js const validateRoute = (context) => async (callback) => { const { id, pathname } = context.query; const paths = await getAllowedPaths(id); if (!paths.includes(pathname)) { return { redirect: "/home", permanente: false, }; } return callback(); };In all my private pages.
export const getServerSideProps = (context) => { return validateRoute(context)(async () => { // my custom callback for each page. const { id } = context.query; const props = await getUserData(id); return { props, }; }); };would you help port this to typescript?
I was able to cast it like this:import { GetServerSidePropsContext, GetServerSidePropsResult } from 'next' import Cookies from 'cookies' type EmptyProps = { props: Record<string, unknown> } // HOC to only be executed when user is not logged in, otherwise redirect to portal const withoutAuth = (context: GetServerSidePropsContext) => async ( callback: () => any ): Promise<GetServerSidePropsResult<EmptyProps>> => { const cookies = new Cookies(context.req, context.res) if (cookies.get('user')) { return { redirect: { destination: '/protected-source', permanent: false, }, } } return callback() } export default withoutAuthI still can figure the typescript definition for the callback... I've ended up doing
callback: () => any
@mtoranzoskw
callback
has no parameter and might return the same values ofwithoutAuth
. Try this out, let me know if it works.const withoutAuth = (context: GetServerSidePropsContext) => async ( callback: () => GetServerSidePropsResult<EmptyProps> ): Promise<GetServerSidePropsResult<EmptyProps>> => {Not working... I've tried that but I have an error when implementing it
Got an error using Promise as well (
callback: () => Promise<GetServerSidePropsResult<EmptyProps>>
)
@mtoranzoskw try to make your props
optional.
type EmptyProps = {
props?: Record<string, unknown>
}
// or
type EmptyProps = {
props: Partial<Record<string, unknown>>
}
Thanks for the help @Sergioamjr
I've tried both, but making props optional was the only one that worked! I guess Partial
would go 1 level up so that props become optional.
type EmptyProps = {
props?: Record<string, unknown>
}
💪
anyone done this without getInialProps ? currently im using next 11 and i want to add auth to my app but so far i couldnt understand the logic around protected routes in next js
Thank a lot
Not working... I've tried that but I have an error when implementing it
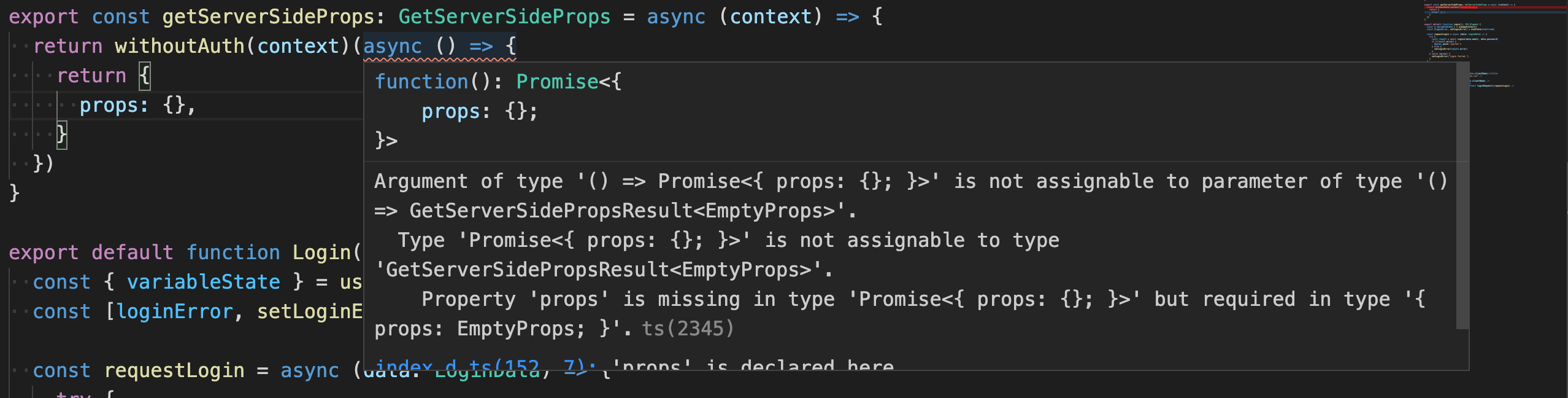
Got an error using Promise as well (
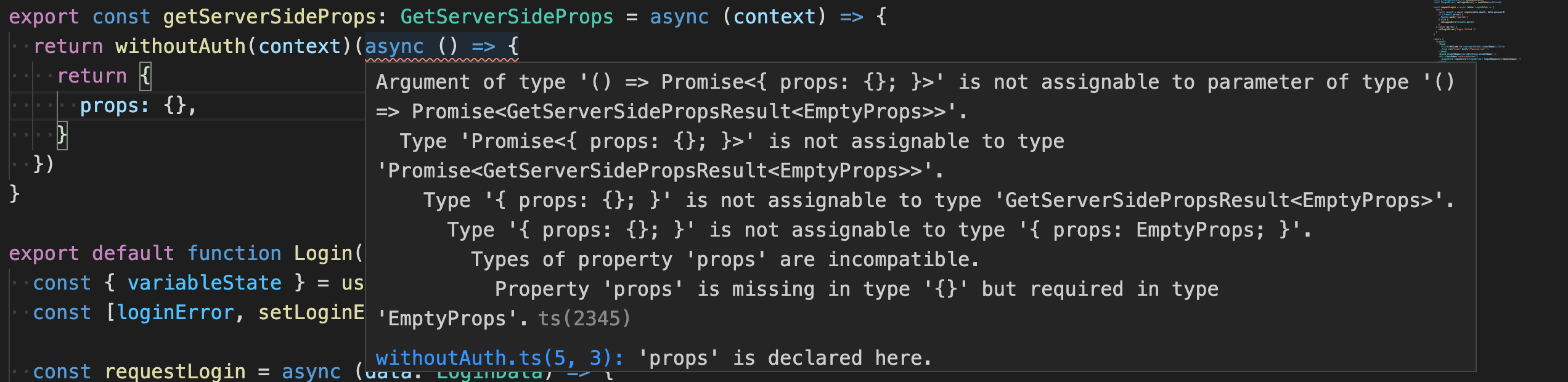
callback: () => Promise<GetServerSidePropsResult<EmptyProps>>
)