Last active
September 17, 2017 18:51
-
-
Save wpmudev-sls/ca31356e3bfca349529e1bdbdb0a6f21 to your computer and use it in GitHub Desktop.
[Membership 2 Pro] - Notifications Preview
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<?php | |
/* | |
Plugin Name: [Membership 2 Pro] - Notifications Preview | |
Plugin URI: https://premium.wpmudev.org/ | |
Description: Adds an option in notifications settings page to send a test email | |
Author: Panos Lyrakis @ WPMUDEV | |
Author URI: https://premium.wpmudev.org/ | |
License: GPLv2 or later | |
*/ | |
if ( ! defined( 'ABSPATH' ) ) { | |
exit; | |
} | |
if ( ! class_exists( 'WPMUDEV_MS_Notification_Preview' ) ) { | |
class WPMUDEV_MS_Notification_Preview { | |
private static $_instance = null; | |
public static function get_instance() { | |
if( is_null( self::$_instance ) ){ | |
self::$_instance = new WPMUDEV_MS_Notification_Preview(); | |
} | |
return self::$_instance; | |
} | |
private function __construct() { | |
add_action( 'admin_footer', array( $this, 'insert_preview_field' ), 10 ); | |
add_action( 'wp_ajax_ms_preview_notification', array( $this, 'ajax_send_preview_notification' ) ); | |
add_filter( 'ms_model_communication_get_comm_vars', array( $this, 'filter_comm_vars' ), 10, 2 ); | |
} | |
public function filter_comm_vars( $comm_vars, $member ){ | |
if( ! wp_doing_ajax() || ! isset( $_POST[ 'action' ] ) || $_POST[ 'action' ] != 'ms_preview_notification' ){ | |
return $comm_vars; | |
} | |
if( isset( $_POST[ 'membership_id' ] ) ){ | |
$membership_id = intval( $_POST[ 'membership_id' ] ); | |
$membership = MS_Factory::load( 'MS_Model_Membership', $membership_id ); | |
$days = 10; // We like number 10 :) | |
$comm_vars[ '%ms-name%' ] = $membership->name; | |
$comm_vars[ '%ms-description%' ] = $membership->description; | |
$comm_vars[ '%ms-remaining-days%' ] = sprintf( | |
__( '%s day%s', 'membership2' ), | |
$days, | |
abs( $days ) > 1 ? 's': '' | |
); | |
$comm_vars[ '%ms-remaining-trial-daysn%' ] = sprintf( | |
__( '%s day%s', 'membership2' ), | |
$days, | |
abs( $days ) > 1 ? 's': '' | |
); | |
$comm_vars[ '%ms-expiry-date%' ] = date( 'Y-m-d', strtotime("+10 days") ); //date( 'D, d F Y', strtotime("+10 days") ); | |
//$comm_vars[ '%ms-invoice%' ] : We need a subscription | |
} | |
return $comm_vars; | |
} | |
public function ajax_send_preview_notification(){ | |
check_ajax_referer( 'ms-preview-notification', 'security' ); | |
$recipient = sanitize_text_field( $_POST['destination_email'] ); | |
$return = array(); | |
if( ! is_email( $recipient ) ){ | |
$return = array( | |
'success' => 0, | |
'message' => __( 'Invalid recipient email address', 'membership2' ) | |
); | |
wp_send_json( $return ); | |
} | |
$comm_type = sanitize_text_field( $_POST['comm_type'] ); | |
$subject = '[PREVIEW] - ' . sanitize_text_field( $_POST[ 'subject' ] ); | |
$member = MS_Factory::load( 'MS_Model_Member', get_current_user_id() ); | |
$MS_Model_Communication = new MS_Model_Communication; | |
$comm_vars = $MS_Model_Communication->get_comm_vars( null, $member ); | |
$args = array( | |
'post_type' => MS_Model_Communication::get_post_type(), | |
'post_status' => 'any', | |
'fields' => 'ids', | |
'posts_per_page' => 1, | |
'meta_query' => array( | |
array( | |
'key' => 'type', | |
'value' => $comm_type, | |
'compare' => '=', | |
), | |
), | |
); | |
MS_Factory::select_blog(); | |
$query = new WP_Query( $args ); | |
$items = $query->posts; | |
MS_Factory::revert_blog(); | |
if ( 1 == count( $items ) ) { | |
$comm_id = $items[0]; | |
} | |
$communication_post = get_post( $comm_id ); | |
$message = str_replace( | |
array_keys( $comm_vars ), | |
array_values( $comm_vars ), | |
stripslashes( $communication_post->post_content ) | |
); | |
$subject = str_replace( | |
array_keys( $comm_vars ), | |
array_values( $comm_vars ), | |
stripslashes( $subject ) | |
); | |
$subject = strip_tags( $subject ); | |
$content_type = $MS_Model_Communication->get_mail_content_type(); | |
$headers[] = sprintf( | |
'Content-Type: %s; charset="UTF-8"', | |
$content_type | |
); | |
$admin_emails = MS_Model_Member::get_admin_user_emails(); | |
if ( ! empty( $admin_emails[0] ) ) { | |
$headers[] = sprintf( | |
'From: %s <%s> ', | |
get_option( 'blogname' ), | |
$admin_emails[0] | |
); | |
} | |
// Final step: Allow customization of all email parts. | |
$recipient = apply_filters( | |
'ms_model_communication_send_preview_message_recipients', | |
$recipient | |
); | |
$message = apply_filters( | |
'ms_model_communication_send_preview_message_contents', | |
$message | |
); | |
$subject = apply_filters( | |
'ms_model_communication_send_preview_message_subject', | |
$subject | |
); | |
$headers = apply_filters( | |
'ms_model_communication_send_preview_message_headers', | |
$headers | |
); | |
/* | |
* Send the mail! | |
* wp_mail will not throw an error, so no error-suppression/handling | |
* is required here. On error the function response is FALSE. | |
*/ | |
$sent = wp_mail( $recipient, $subject, $message, $headers ); | |
if( ! $sent ){ | |
$return = array( | |
'success' => 0, | |
'message' => __( 'Something went wrong and could not send test email', 'membership2' ) | |
); | |
} | |
else{ | |
$return = array( | |
'success' => 1, | |
'message' => __( 'Preview email was sent!', 'membership2' ) | |
); | |
} | |
wp_send_json( $return ); | |
} | |
public function insert_preview_field(){ | |
$screen = get_current_screen(); | |
if( 'membership-2_page_membership2-settings' != $screen->id ){ | |
return; | |
} | |
$all_memberships = MS_Model_Membership::get_memberships(); | |
$memberships_options = ''; | |
foreach ( $all_memberships as $membership ) { | |
$memberships_options .= '<option value="' . $membership->id . '">' . $membership->name . '</option>'; | |
} | |
?> | |
<script type="text/javascript"> | |
(function($){ | |
var MS_Preview_Notification = { | |
title_text: 'Send Preview email', | |
label_text_email: 'Insert destination email', | |
label_text_membership: 'Select a membership', | |
button_text: 'Send', | |
warning_email_text: 'Please check your destination email', | |
place: $('.ms-editor-form #cc_email'), | |
nonce: '<?php echo wp_create_nonce( "ms-preview-notification" ); ?>', | |
comm_type: '<?php echo isset( $_GET['comm_type'] ) ? $_GET['comm_type'] : ''; ?>', | |
all_memberships : '<?php echo $memberships_options; ?>', | |
create_preview_field: function(){ | |
var cc_email = $( '#cc_email' ).val(); | |
var field = $( '<div/>',{ | |
"class": 'ms-email-preview', | |
'style': 'border: 2px solid #f3f3f3; padding: 10px;' | |
} ); | |
var title = $( '<span/>', { | |
"class": 'ms-email-preview-title', | |
text: this.title_text, | |
"style": 'font-weight: bold' | |
}); | |
var email_label = $( '<label/>', { | |
"class": 'ms-email-preview-emaillabel', | |
text: this.label_text_email, | |
"style": 'display: inline-block;' | |
}); | |
var membership_label = $( '<label/>', { | |
"class": 'ms-email-preview-membershiplabel', | |
text: this.label_text_membership, | |
"style": 'display: inline-block;' | |
}); | |
var input = $( '<input/>', { | |
"class": 'ms-email-preview-input', | |
type: 'text', | |
value: cc_email, | |
"style": 'margin-left: 10px' | |
}); | |
var select_membership = $( '<select/>',{ | |
"class": 'ms-email-preview-membership' | |
}).append( this.all_memberships ); | |
var button = $( '<a/>', { | |
"class": 'ms-email-preview-button button-primary', | |
text: this.button_text, | |
"style": 'margin-left: 10px; text-align: center;' | |
}); | |
field.append( title ); | |
email_label.append( input );//.append( button ); | |
membership_label.append( select_membership ) | |
field.append( email_label ).append( membership_label ).append( button ); | |
//field.append( email_label ).append( button ); | |
return field; | |
}, | |
insert_preview_field: function(){ | |
this.place.after( this.create_preview_field() ); | |
}, | |
send_preview: function(){ | |
var destination_email = $( '.ms-email-preview .ms-email-preview-input' ).val(), | |
subject = $( '#subject' ).val(), | |
comm_type = this.comm_type, //$( '#comm_type' ).val(), | |
membership_id = $( '.ms-email-preview-membership' ).val(); | |
if( destination_email == '' || ! this.is_email(destination_email) ){ | |
alert( this.warning_email_text ); | |
return; | |
} | |
var data = { | |
action: 'ms_preview_notification', | |
security: this.nonce, | |
destination_email: destination_email, | |
subject: subject, | |
comm_type: comm_type, | |
membership_id: membership_id | |
}; | |
$.post(ajaxurl, data, function(response) { | |
if( ! response.success ){ | |
alert( response.message ); | |
return; | |
} | |
var success_msg = $( '<div/>',{ | |
"class": 'notice notice-success is-dismissible ms-email-preview-successmsg', | |
text: response.message | |
}); | |
$( '.ms-email-preview' ).append( success_msg ); | |
success_msg.show( 300 ).delay(5000).fadeOut( 300, function(){ success_msg.remove(); } ); | |
}); | |
}, | |
is_email: function(email) { | |
var regex = /^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,4})+$/; | |
return regex.test(email); | |
} | |
}; | |
$(document).ready(function(){ | |
MS_Preview_Notification.insert_preview_field(); | |
$(document).on( 'click', '.ms-email-preview-button', function(){ | |
MS_Preview_Notification.send_preview(); | |
}); | |
}); | |
})(jQuery) | |
</script> | |
<?php | |
} | |
} | |
add_action( 'plugins_loaded', function(){ | |
$GLOBALS['WPMUDEV_MS_Notification_Preview'] = WPMUDEV_MS_Notification_Preview::get_instance(); | |
}, 10 ); | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
It should be adding a new section in
admin > Membership 2 > Settings > Automated Email Responses tab
right under the form.
Fill in the email and select a destination email address so it sends a test email to that address.
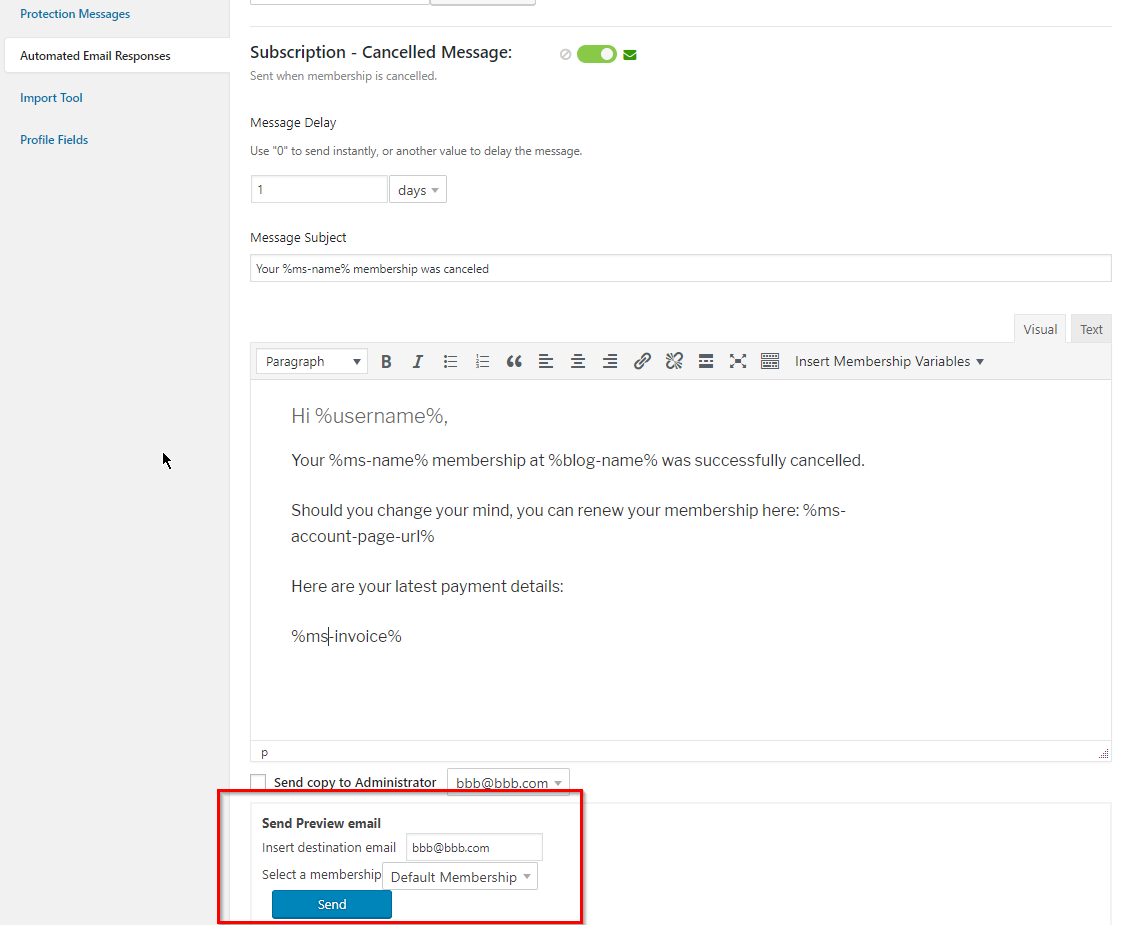