Created
August 3, 2014 13:34
-
-
Save yemrekeskin/16cb2eb71fb337dd98f3 to your computer and use it in GitHub Desktop.
Sequential Guid Generator C#
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
public interface IIdentifierGenerator | |
{ | |
Guid SequentialGuid(); | |
} | |
public class GuidCombGenerator | |
: IIdentifierGenerator | |
{ | |
public Guid SequentialGuid() | |
{ | |
return GenerateComb(); | |
} | |
/// <summary> | |
/// Generate a new <see cref="Guid"/> using the comb algorithm. | |
/// </summary> | |
private Guid GenerateComb() | |
{ | |
byte[] guidArray = Guid.NewGuid().ToByteArray(); | |
DateTime baseDate = new DateTime(1900, 1, 1); | |
DateTime now = DateTime.Now; | |
// Get the days and milliseconds which will be used to build the byte string | |
TimeSpan days = new TimeSpan(now.Ticks - baseDate.Ticks); | |
TimeSpan msecs = now.TimeOfDay; | |
// Convert to a byte array | |
// Note that SQL Server is accurate to 1/300th of a millisecond so we divide by 3.333333 | |
byte[] daysArray = BitConverter.GetBytes(days.Days); | |
byte[] msecsArray = BitConverter.GetBytes((long)(msecs.TotalMilliseconds / 3.333333)); | |
// Reverse the bytes to match SQL Servers ordering | |
Array.Reverse(daysArray); | |
Array.Reverse(msecsArray); | |
// Copy the bytes into the guid | |
Array.Copy(daysArray, daysArray.Length - 2, guidArray, guidArray.Length - 6, 2); | |
Array.Copy(msecsArray, msecsArray.Length - 4, guidArray, guidArray.Length - 4, 4); | |
return new Guid(guidArray); | |
} | |
} |
I'm not in front of a computer right now but I think you are missing the single quotes around the guid in your sql code.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hi, I'm trying to work with your GUID, however MSSQL does not want to accept them and write to the database. What could be wrong?
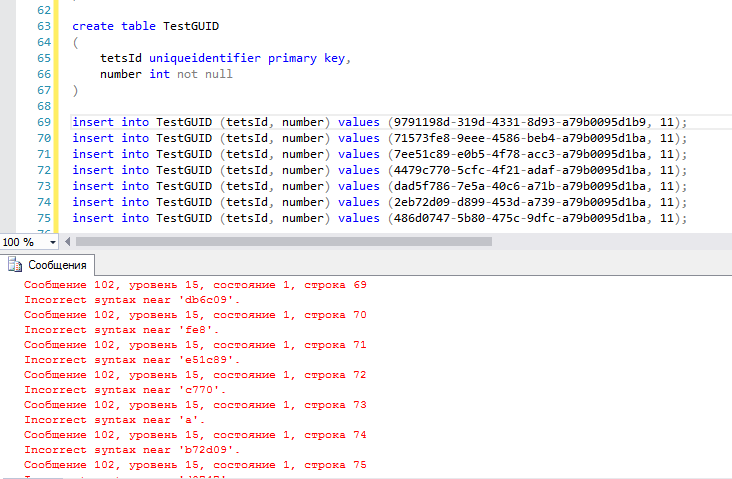
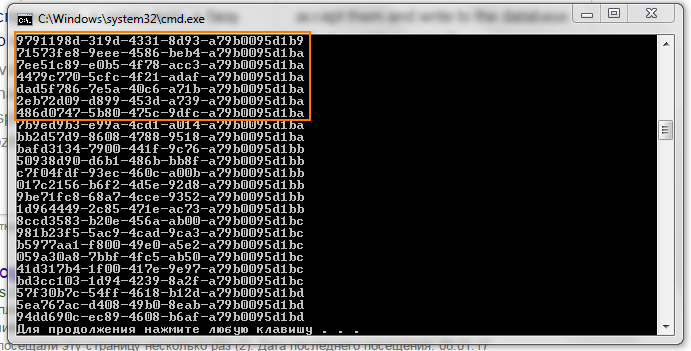