Last active
May 25, 2018 08:03
-
-
Save yuchiki/e779b07b12b9993e4d9a26345e161961 to your computer and use it in GitHub Desktop.
sample for understanding the behavior of 'await' in C#. inspired by Jake Archibald's interesting quiz: https://twitter.com/jaffathecake/status/999269332889763840
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using System; | |
using System.Threading.Tasks; | |
class Program { | |
const bool UseCompletedTask = true; // You can change this. | |
//If you use the completed task, "2 3" must be printed, because the program is run synchronously. | |
//If you use the incompleted task, "1 2", "2 1", "2 3", ... may be printed, because the program is run asynchronously. | |
static void Main() { | |
var x = 0; | |
async Task Test() { | |
var task = UseCompletedTask ? Task.FromResult(2) : Task.Run(() => 2); | |
x += await task; | |
Console.WriteLine(x); | |
} | |
Test(); | |
x += 1; | |
Console.WriteLine(x); | |
Task.Delay(100).Wait(); | |
} | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This is the result when I use the completed task.
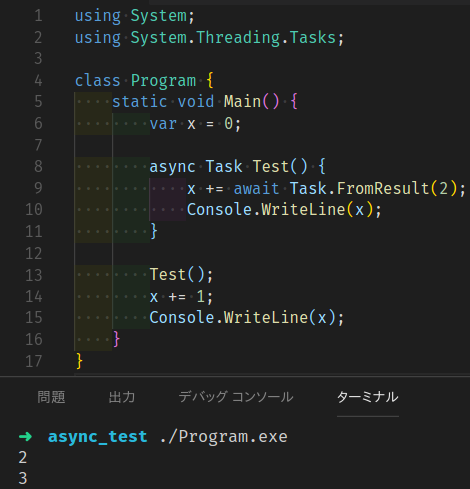
This is the result when I use the incompleted task.
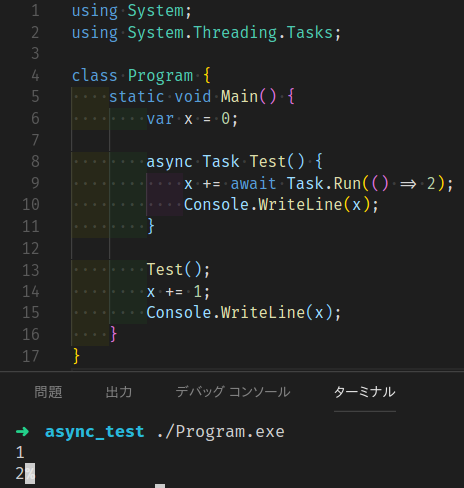