Created
January 5, 2018 20:13
-
-
Save zkamvar/3c74225731ee414b420ddd59773e66b8 to your computer and use it in GitHub Desktop.
Generate guides for making a poster in inkscape
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#' Get the size of a single panel | |
#' | |
#' @param n the number of panels | |
#' @param len the overall length of the side | |
#' @param margin the outer margin width (will be multiplied by 2) | |
#' @param gutter the space between panels | |
#' | |
#' @return a numeric value specifying the panel width/length | |
#' | |
#' @examples | |
#' get_panel_size() | |
get_panel_size <- function(n = 4, len = 48, margin = 1, gutter = 0.5){ | |
all_gutters <- (n - 1) * gutter | |
panel_size <- (len - margin - margin - all_gutters)/n | |
return(panel_size) | |
} | |
#' Get the size of a single panel | |
#' | |
#' @param n the number of panels | |
#' @param len the overall length of the side | |
#' @param margin the outer margin width (will be multiplied by 2) | |
#' @param gutter the space between panels | |
#' | |
#' @return a numeric vector delimiting the panels. | |
#' | |
#' @examples | |
#' get_guides() | |
#' diff(get_guides()) # note the differences are either the gutter or panel size | |
#' get_panel_size() | |
get_guides <- function(n = 4, len = 48, margin = 1, gutter = 0.5){ | |
panel_size <- get_panel_size(n = n, len = len, margin = margin, gutter = gutter) | |
inner <- seq(from = 1, to = n - 1) | |
mat <- matrix(0.0, ncol = n - 1, nrow = 2) | |
gutters <- cumsum(rep(gutter, n - 1)) - gutter # the cumulative gutters, starting at 0 | |
panels <- (panel_size * inner) + gutters # panels and left gutters | |
mat[1, ] <- panels + margin # shifting by left margin | |
mat[2, ] <- mat[1, ] + gutter # adding the right gutters | |
c(margin, mat, len - margin) # putting it all together | |
} | |
# | |
# An example 36x48 poster with 1.5 inch margins and a half an inch gutter and | |
# six panels. | |
if (interactive()){ | |
(columns <- get_guides(n = 3, len = 48, margin = 1.5, gutter = 0.5)) | |
(rows <- get_guides(n = 2, len = 36, margin = 1.5, gutter = 0.5)) | |
plot(0, 0, xlim = c(0, 48), ylim = c(0, 36), asp = 1, | |
ann = F, bty = 'n', type = 'n', xaxt = 'n', yaxt = 'n') | |
rect(0, 0, 48, 36, col = "grey98") | |
abline(v = columns, col = "blue", lty = 2) | |
abline(h = rows, col = "blue", lty = 2) | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Here's the output of the example:
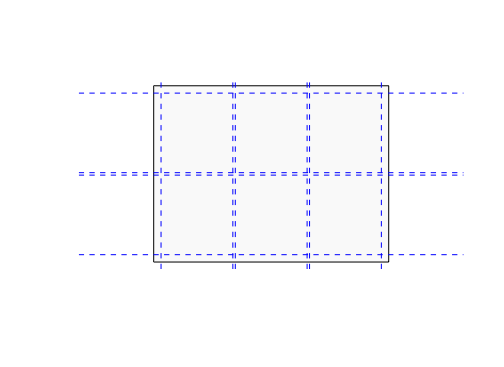