Since GitHub READEME doesn't seems to sopport math mode like $\int_0^1 cos(\theta) d\theta = 1$
We can workaround this using codecogs which is an online latex equation editor which provvides it's outpus as gifs.
The base gif url is https://latex.codecogs.com/gif.latex?
which is followed by the percentage encoded query.
So i made this function to convert an equation to a Markdown image:
def convert_eq(text):
encoded = "".join([encoding.get(c,c) for c in text])
url = base_url + encoded
md = ""%url
return md
That when called:
convert_eq("""\int_0^1 cos(\theta) d\theta = 1""")
gives:
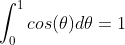
which in markdown is:
This can be generalized to convert all the occurance of the double dollars in a file:
#!/usr/bin/env python
import re
import sys
base_url = """https://latex.codecogs.com/gif.latex?"""
encoding = {
"!": "%21",
"#": "%23",
"$": "%24",
"&": "%26",
"''": "%27",
"(": "%28",
")": "%29",
"*": "%2A",
"+": "%2B",
",": "%2C",
"/": "%2F",
":": "%3A",
";": "%3B",
"=": "%3D",
"?": "%3F",
"@": "%40",
"[": "%5B",
"]": "%5D",
" ": "%20",
'""': "%22",
"%": "%25",
"-": "%2D",
".": "%2E",
"<": "%3C",
"?": "%3E",
"\\": "%5C",
"^": "%5E",
"_": "%5F",
"`": "%60",
"{": "%7B",
"|": "%7C",
"}": "%7D",
"~": "%7E"
}
pattern = re.compile(r"""[^\\]\$([^\$]+[^\\])\$""")
def latex_to_md(text):
for value in re.finditer(pattern, text):
encoded = "".join([encoding.get(c,c) for c in value.group(1)])
url = base_url + encoded
md = ""%url
text = text.replace(value.group(0), md)
text = text.replace("\\$","$")
return text
if len(sys.argv) not in [2,3]:
print("To few arguments, you need to pass the filepath and optionally the name of the new file.")
sys.exit()
file = sys.argv[1]
# If passed set the new file to the args else overwritte the source file
if len(sys.argv) == 2:
new_file = file
else:
new_file = sys.argv[2]
# Read the file
with open(file,"r") as f:
text = f.read()
# Convert it
text = latex_to_md(text)
# Write the new_file
with open(new_file,"w") as f:
f.write(text)