I borrowed crt.hlsl from Hammster although I've tweaked it a little.
You need a terminal profile with something like this in it:
{
// This is the shader and a background with the monitor in it, because that's easy
"experimental.pixelShaderPath": "C:\\Users\\Jaykul\\crt.hlsl",
"backgroundImage": "C:\\Users\\Jaykul\\crt.png",
"backgroundImageAlignment": "center",
"backgroundImageOpacity": 1.0,
"backgroundImageStretchMode": "fill",
// Ideally, you need to use a color scheme with a green or yellow default foreground color:
"colorScheme": "Vintage",
"cursorShape": "vintage",
// But you can use it with any shell you like:
"useAcrylic": true,
"name": "PowerShell 7 Preview",
"source": "Windows.Terminal.PowershellCore",
"guid": "{a3a2e83a-884a-5379-baa8-16f193a13b21}"
}
You want a color scheme that has that green or yellow font. You might want to use all shades of green, but I can't stand that, so I'm just not authentic. Oh well:
{
"background": "#212021",
"black": "#212021",
"blue": "#01A0E4",
"brightBlack": "#493F3F",
"brightBlue": "#6ECEFF",
"brightCyan": "#95F2FF",
"brightGreen": "#6CD18E",
"brightPurple": "#D29BC6",
"brightRed": "#FF6E6D",
"brightWhite": "#FFFCFF",
"brightYellow": "#FFFF85",
"cursorColor": "#FFFFFF",
"cyan": "#55C4CF",
"foreground": "#397339",
"green": "#01A252",
"name": "Vintage",
"purple": "#A16A94",
"red": "#D92D20",
"selectionBackground": "#FFFFFF",
"white": "#A5A2A2",
"yellow": "#FBED02"
}
Then, of course, you need the background image something like this:
Here's a screenshot, which doesn't do it justice. Frankly, you have to see it in action because there's actually a moving faux refresh line and more...
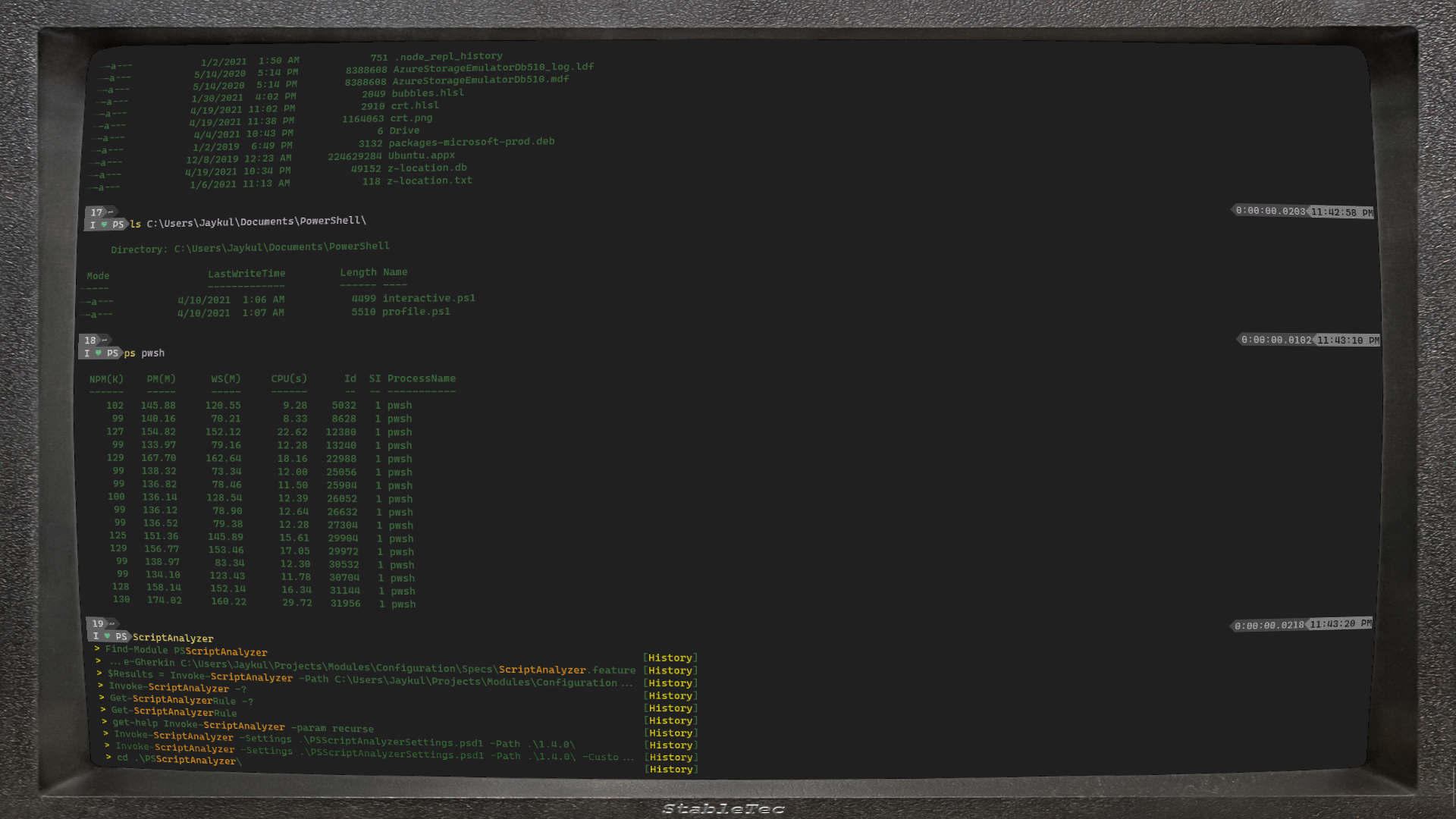