-
Did I run into any issues? yes, I had some issues with getting XCode and Homebrew setup correctly. I needed to update OS X from 10.9 to 10.12. Lots of negative comments in app store about these updates.. Also, my bash commands somehow got messed up. Somehow my PATH seems to have gotten really screwed up. I think it was when I was trying to modify the path to get Homebrew to get going. Found the correct PATHs on stackoverflow: export PATH="/usr/bin:/bin:/usr/sbin:/sbin" export PATH="/usr/local/bin:/usr/local/sbin:$PATH" brew install ruby
-
Type atom into the terminal
-
.rb (for ruby)
-
cmd+/ or cmd+k+b, also cmd+shift+p is awesome
-
cmd+p+t
-
pwd writes the full path name of the current working directory, super useful for debugging or making sure we're in the correct location in the terminal
-
ls lists the files in the current working directory, cd is 'change directory', mkdir is 'make directory' (Essetially creates a new folder)
-
hostname is the server we're currently communicating with, kinda like a domain name online. Mine currently is 'local'
-
All setup below:
- typing irb into the terminal will open an interactive (i) ruby (rb) session
- **2 is the expression to square a number with ** indicates an exponent 1strings) puts outputs to terminal
- make sure pwd is in the correct directory, then call ruby with the file name ex: ruby ex1.rb, If you're calling from a directory above, you can type the folder name ex: ruby folder/ex1.rb
- /# indicates a comment, this disables the line of text when interpreting. Useful to explaining what you're doing and also debugging
- 6
A Pomodoro break is a is either a short 5 minute break after a 25 minute 'work' session, or a longer 30 minute break after 4 25 minute 'work' sessions
- create a new variable like so: variable_name = 9 or 'string' or boolean(true / false)
- it should only contain latin letters, numbers and underscores, also it should never start with a number
- the same way it was set originally variable_name = new_value
- to find out the datatype of a variable use: variable_name.class
- .slice, .partition, .chomp (can be found using .methods)
- changing an integer to string use: integer.to_s
- double quotes allow other non-string code to exist inside of it, such as inserting #{variable} also double quotes allow interpolation. Seems like single quotes are for strings that need to stay permanent.
- It is used for inserting variables and/or executable code within a string. similar to the %d, %s, etc in python.
- cool function, use 'string'.delete('aeiou')
- print will send output to console without a newline, while puts will send output to console with a newline
- gets asks for user input in the console while the script is running.
- Integers are whole numbers without decimals (or decimals truncated). Floats include the decimals.
- 2**2
-
== checks if values are equal, >= checks if a value on left is greaterthan or equal to the right, <= checks if a value is lessthan or equal, != checks if values are not equal, && replaces AND to check if multiple conditions are true, || replaces OR to check if atleast one of multiple conditions are true.
-
end_with?, include?, empty? --- most methods that end with a ? will return a boolean
- Flow control is using if/elsif/else statements to have the program make decisions for us.
- 'Not many apples...', since apple_count is less than 5 we skip the if condition and use the else statement.
- An infinite loop is when a loop such as 'while' or 'for' will never reach a condition that ends it. CTRL+C will stop a script in the terminal.
- nil indicates nothing. Can be used in a variable or a dataset. nil != 0
- Symbols allow ruby to point to an object from multiple places instead of creating a new copy. It saves memory. a symbol will have both a string represenation and a numerical representation.
- Similarly, never start them with a number. However, looks like you can use anthing that you'd put in a string.
- .length will give the number of elements
- 0
- .push - adds an element to the end of an array, .pop - removes and returns the last element of an array, can change the element by using pop(index)
- Hashes are a keyed list, where a 'key' can contain multiple underline values that are immutable. Arrays can be modified easily and items are indexed to their specific numerical slots. Hashses do not have a specific order.
- Arrays are good if you need to modify the specifc order, such as shuffling a deck. Hashes are good when you're trying to setup a group of values that cannot be changed, like a dictionary that cannot be changed.
- "Turing"[0]
- "Turing".length
- "Turing".upcase
- "Turing".delete("n")
- my_school = "Turing"
- gets asks the user to input something with the keyboard into the terminal
- the .chomp will remove the return at the end of the line of inputed text
- it converts the input, which is a string, into an integer
- 4
- "dog"
- false
- animals << 'string' , animals.push('string')
- .pop()
Game code below, also in my github profile:
puts "I have generated a random number for you to guess, what is your guess?"
mystery_number = rand(1..100) is_correct = false num_of_guesses = 0 integral_limit = (mystery_number**2 - 1) puts integral_limit puts mystery_number #puts mystery_number #I put this in there to help debug the program while is_correct != true #this section checks if the user input is either a number or cheat. If it is c #then it will make the users guess the mystery number. If the user inputs anything #but an integer or cheat it will ask for a new integer begin user_guess = gets.chomp num_of_guesses += 1 if user_guess[0].downcase == "c" puts "The mystery number is #{mystery_number}" user_guess = mystery_number else user_guess = Integer(user_guess) end rescue print "Please enter a number:" retry end
if user_guess == mystery_number puts "You guessed the right number!" is_correct = true elsif user_guess > mystery_number puts "Lower..." elsif user_guess < mystery_number puts "Higher..." else puts "Guess again" end
if num_of_guesses > 2 puts "You need a hint, I'll give you one but it won't be easy." puts "The mystery number can be found through this equation:" puts "1 plus the integral of 1/(2*sqrt(x+1)) over the range zero to #{x}" puts "use integral-calculator.com if you don't want to do this by hand!" end end
Typing Level 1:
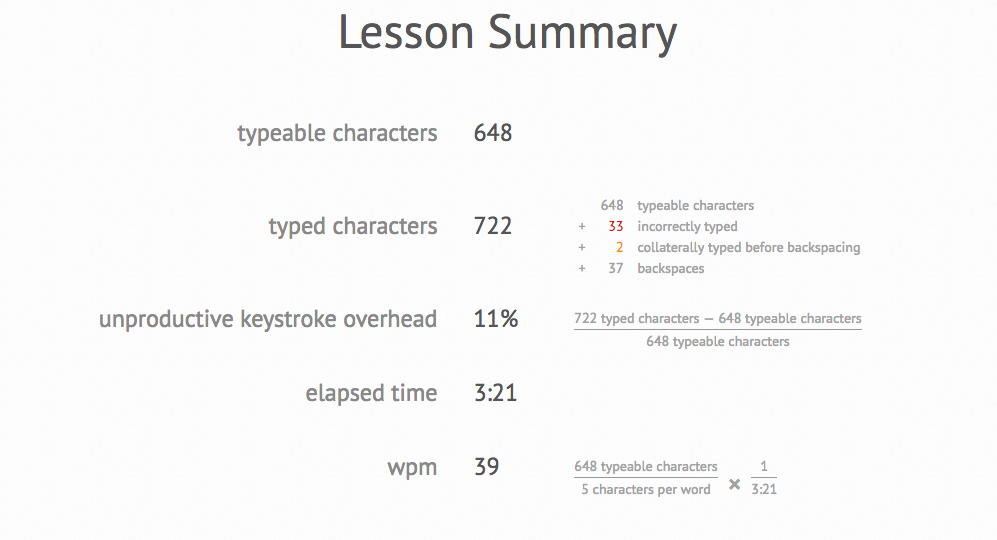