-
-
Save Seneral/2c8e7dfe712b9f53c60f80722fbce5bd to your computer and use it in GitHub Desktop.
using UnityEngine; | |
using System.Collections; | |
using System.Collections.Generic; | |
using System; | |
using System.Reflection; | |
namespace NodeEditorFramework.Utilities | |
{ | |
public static class GUIScaleUtility | |
{ | |
// General | |
private static bool compabilityMode; | |
private static bool initiated; | |
// cache the reflected methods | |
private static FieldInfo currentGUILayoutCache; | |
private static FieldInfo currentTopLevelGroup; | |
// Delegates to the reflected methods | |
private static Func<Rect> GetTopRectDelegate; | |
private static Func<Rect> topmostRectDelegate; | |
// Delegate accessors | |
public static Rect getTopRect { get { return (Rect)GetTopRectDelegate.Invoke (); } } | |
public static Rect getTopRectScreenSpace { get { return (Rect)topmostRectDelegate.Invoke (); } } | |
// Rect stack for manipulating groups | |
public static List<Rect> currentRectStack { get; private set; } | |
private static List<List<Rect>> rectStackGroups; | |
// Matrices stack | |
private static List<Matrix4x4> GUIMatrices; | |
private static List<bool> adjustedGUILayout; | |
private static bool isEditorWindow; | |
#region Init | |
public static void CheckInit () | |
{ | |
if (!initiated) | |
Init (); | |
} | |
public static void Init () | |
{ | |
// Fetch rect acessors using Reflection | |
Assembly UnityEngine = Assembly.GetAssembly (typeof (UnityEngine.GUI)); | |
Type GUIClipType = UnityEngine.GetType ("UnityEngine.GUIClip", true); | |
#if UNITY_2018_2_OR_NEWER | |
MethodInfo topmostRect = GUIClipType.GetMethod("get_topmostRect", BindingFlags.Static | BindingFlags.NonPublic); | |
#else | |
PropertyInfo topmostRect = GUIClipType.GetProperty ("topmostRect", BindingFlags.Static | BindingFlags.Public); | |
#endif | |
MethodInfo GetTopRect = GUIClipType.GetMethod ("GetTopRect", BindingFlags.Static | BindingFlags.NonPublic); | |
MethodInfo ClipRect = GUIClipType.GetMethod ("Clip", BindingFlags.Static | BindingFlags.Public, Type.DefaultBinder, new Type[] { typeof(Rect) }, new ParameterModifier[] {}); | |
if (GUIClipType == null || topmostRect == null || GetTopRect == null || ClipRect == null) | |
{ | |
Debug.LogWarning ("GUIScaleUtility cannot run on this system! Compability mode enabled. For you that means you're not able to use the Node Editor inside more than one group:( Please PM me (Seneral @UnityForums) so I can figure out what causes this! Thanks!"); | |
Debug.LogWarning ((GUIClipType == null? "GUIClipType is Null, " : "") + (topmostRect == null? "topmostRect is Null, " : "") + (GetTopRect == null? "GetTopRect is Null, " : "") + (ClipRect == null? "ClipRect is Null, " : "")); | |
compabilityMode = true; | |
initiated = true; | |
return; | |
} | |
// Create simple acessor delegates | |
GetTopRectDelegate = (Func<Rect>)Delegate.CreateDelegate (typeof(Func<Rect>), GetTopRect); | |
#if UNITY_2018_2_OR_NEWER | |
topmostRectDelegate = (Func<Rect>)Delegate.CreateDelegate(typeof(Func<Rect>), topmostRect); | |
#else | |
topmostRectDelegate = (Func<Rect>)Delegate.CreateDelegate (typeof(Func<Rect>), topmostRect.GetGetMethod ()); | |
#endif | |
if (GetTopRectDelegate == null || topmostRectDelegate == null) | |
{ | |
Debug.LogWarning ("GUIScaleUtility cannot run on this system! Compability mode enabled. For you that means you're not able to use the Node Editor inside more than one group:( Please PM me (Seneral @UnityForums) so I can figure out what causes this! Thanks!"); | |
Debug.LogWarning ((GUIClipType == null? "GUIClipType is Null, " : "") + (topmostRect == null? "topmostRect is Null, " : "") + (GetTopRect == null? "GetTopRect is Null, " : "") + (ClipRect == null? "ClipRect is Null, " : "")); | |
compabilityMode = true; | |
initiated = true; | |
return; | |
} | |
// As we can call Begin/Ends inside another, we need to save their states hierarchial in Lists (not Stack, as we need to iterate over them!): | |
currentRectStack = new List<Rect> (); | |
rectStackGroups = new List<List<Rect>> (); | |
GUIMatrices = new List<Matrix4x4> (); | |
adjustedGUILayout = new List<bool> (); | |
// Sometimes, strange errors pop up (related to Mac?), which we try to catch and enable a compability Mode no supporting zooming in groups | |
/*try | |
{ | |
topmostRectDelegate.Invoke (); | |
} | |
catch (Exception e) | |
{ | |
Debug.LogWarning ("GUIScaleUtility cannot run on this system! Compability mode enabled. For you that means you're not able to use the Node Editor inside more than one group:( Please PM me (Seneral @UnityForums) so I can figure out what causes this! Thanks!"); | |
Debug.Log (e.Message); | |
compabilityMode = true; | |
}*/ | |
initiated = true; | |
} | |
#endregion | |
#region Scale Area | |
public static Vector2 getCurrentScale { get { return new Vector2 (1/GUI.matrix.GetColumn (0).magnitude, 1/GUI.matrix.GetColumn (1).magnitude); } } | |
/// <summary> | |
/// Begins a scaled local area. | |
/// Returns vector to offset GUI controls with to account for zooming to the pivot. | |
/// Using adjustGUILayout does that automatically for GUILayout rects. Theoretically can be nested! | |
/// </summary> | |
public static Vector2 BeginScale (ref Rect rect, Vector2 zoomPivot, float zoom, bool IsEditorWindow, bool adjustGUILayout) | |
{ | |
isEditorWindow = IsEditorWindow; | |
Rect screenRect; | |
if (compabilityMode) | |
{ // In compability mode, we will assume only one top group and do everything manually, not using reflected calls (-> practically blind) | |
GUI.EndGroup (); | |
screenRect = rect; | |
#if UNITY_EDITOR | |
if (isEditorWindow) | |
screenRect.y += 23; | |
#endif | |
} | |
else | |
{ // If it's supported, we take the completely generic way using reflected calls | |
GUIScaleUtility.BeginNoClip (); | |
screenRect = GUIScaleUtility.GUIToScaledSpace (rect); | |
} | |
rect = Scale (screenRect, screenRect.position + zoomPivot, new Vector2 (zoom, zoom)); | |
// Now continue drawing using the new clipping group | |
GUI.BeginGroup (rect); | |
rect.position = Vector2.zero; // Adjust because we entered the new group | |
// Because I currently found no way to actually scale to a custom pivot rather than (0, 0), | |
// we'll make use of a cheat and just offset it accordingly to let it appear as if it would scroll to the center | |
// Note, due to that, controls not adjusted are still scaled to (0, 0) | |
Vector2 zoomPosAdjust = rect.center - screenRect.size/2 + zoomPivot; | |
// For GUILayout, we can make this adjustment here if desired | |
adjustedGUILayout.Add (adjustGUILayout); | |
if (adjustGUILayout) | |
{ | |
GUILayout.BeginHorizontal (); | |
GUILayout.Space (rect.center.x - screenRect.size.x + zoomPivot.x); | |
GUILayout.BeginVertical (); | |
GUILayout.Space (rect.center.y - screenRect.size.y + zoomPivot.y); | |
} | |
// Take a matrix backup to restore back later on | |
GUIMatrices.Add (GUI.matrix); | |
// Scale GUI.matrix. After that we have the correct clipping group again. | |
GUIUtility.ScaleAroundPivot (new Vector2 (1/zoom, 1/zoom), zoomPosAdjust); | |
return zoomPosAdjust; | |
} | |
/// <summary> | |
/// Ends a scale region previously opened with BeginScale | |
/// </summary> | |
public static void EndScale () | |
{ | |
// Set last matrix and clipping group | |
if (GUIMatrices.Count == 0 || adjustedGUILayout.Count == 0) | |
throw new UnityException ("GUIScaleUtility: You are ending more scale regions than you are beginning!"); | |
GUI.matrix = GUIMatrices[GUIMatrices.Count-1]; | |
GUIMatrices.RemoveAt (GUIMatrices.Count-1); | |
// End GUILayout zoomPosAdjustment | |
if (adjustedGUILayout[adjustedGUILayout.Count-1]) | |
{ | |
GUILayout.EndVertical (); | |
GUILayout.EndHorizontal (); | |
} | |
adjustedGUILayout.RemoveAt (adjustedGUILayout.Count-1); | |
// End the scaled group | |
GUI.EndGroup (); | |
if (compabilityMode) | |
{ // In compability mode, we don't know the previous group rect, but as we cannot use top groups there either way, we restore the screen group | |
if (isEditorWindow) // We're in an editor window | |
GUI.BeginClip (new Rect (0, 23, Screen.width, Screen.height-23)); | |
else | |
GUI.BeginClip (new Rect (0, 0, Screen.width, Screen.height)); | |
} | |
else | |
{ // Else, restore the clips (groups) | |
GUIScaleUtility.RestoreClips (); | |
} | |
} | |
#endregion | |
#region Clips Hierarchy | |
/// <summary> | |
/// Begins a field without groups. They should be restored using RestoreClips. Can be nested! | |
/// </summary> | |
public static void BeginNoClip () | |
{ | |
// Record and close all clips one by one, from bottom to top, until we hit the 'origin' | |
List<Rect> rectStackGroup = new List<Rect> (); | |
Rect topMostClip = getTopRect; | |
while (topMostClip != new Rect (-10000, -10000, 40000, 40000)) | |
{ | |
rectStackGroup.Add (topMostClip); | |
GUI.EndClip (); | |
topMostClip = getTopRect; | |
} | |
// Store the clips appropriately | |
rectStackGroup.Reverse (); | |
rectStackGroups.Add (rectStackGroup); | |
currentRectStack.AddRange (rectStackGroup); | |
} | |
/// <summary> | |
/// Begins a field without the last count groups. They should be restored using RestoreClips. Can be nested! | |
/// </summary> | |
public static void MoveClipsUp (int count) | |
{ | |
// Record and close all clips one by one, from bottom to top, until reached the count or hit the 'origin' | |
List<Rect> rectStackGroup = new List<Rect> (); | |
Rect topMostClip = getTopRect; | |
while (topMostClip != new Rect (-10000, -10000, 40000, 40000) && count > 0) | |
{ | |
rectStackGroup.Add (topMostClip); | |
GUI.EndClip (); | |
topMostClip = getTopRect; | |
count--; | |
} | |
// Store the clips appropriately | |
rectStackGroup.Reverse (); | |
rectStackGroups.Add (rectStackGroup); | |
currentRectStack.AddRange (rectStackGroup); | |
} | |
/// <summary> | |
/// Restores the clips removed in BeginNoClip or MoveClipsUp | |
/// </summary> | |
public static void RestoreClips () | |
{ | |
if (rectStackGroups.Count == 0) | |
{ | |
Debug.LogError ("GUIClipHierarchy: BeginNoClip/MoveClipsUp - RestoreClips count not balanced!"); | |
return; | |
} | |
// Read and restore clips one by one, from top to bottom | |
List<Rect> rectStackGroup = rectStackGroups[rectStackGroups.Count-1]; | |
for (int clipCnt = 0; clipCnt < rectStackGroup.Count; clipCnt++) | |
{ | |
GUI.BeginClip (rectStackGroup[clipCnt]); | |
currentRectStack.RemoveAt (currentRectStack.Count-1); | |
} | |
rectStackGroups.RemoveAt (rectStackGroups.Count-1); | |
} | |
#endregion | |
#region Layout & Matrix Ignores | |
/// <summary> | |
/// Ignores the current GUILayout cache and begins a new one. Cannot be nested! | |
/// </summary> | |
public static void BeginNewLayout () | |
{ | |
if (compabilityMode) | |
return; | |
// Will mimic a new layout by creating a new group at (0, 0). Will be restored though after ending the new Layout | |
Rect topMostClip = getTopRect; | |
if (topMostClip != new Rect (-10000, -10000, 40000, 40000)) | |
GUILayout.BeginArea (new Rect (0, 0, topMostClip.width, topMostClip.height)); | |
else | |
GUILayout.BeginArea (new Rect (0, 0, Screen.width, Screen.height)); | |
} | |
/// <summary> | |
/// Ends the last GUILayout cache | |
/// </summary> | |
public static void EndNewLayout () | |
{ | |
if (!compabilityMode) | |
GUILayout.EndArea (); | |
} | |
/// <summary> | |
/// Begins an area without GUIMatrix transformations. Can be nested! | |
/// </summary> | |
public static void BeginIgnoreMatrix () | |
{ | |
// Store and clean current matrix | |
GUIMatrices.Add (GUI.matrix); | |
GUI.matrix = Matrix4x4.identity; | |
} | |
/// <summary> | |
/// Restores last matrix ignored with BeginIgnoreMatrix | |
/// </summary> | |
public static void EndIgnoreMatrix () | |
{ | |
if (GUIMatrices.Count == 0) | |
throw new UnityException ("GUIScaleutility: You are ending more ignoreMatrices than you are beginning!"); | |
// Read and assign previous matrix | |
GUI.matrix = GUIMatrices[GUIMatrices.Count-1]; | |
GUIMatrices.RemoveAt (GUIMatrices.Count-1); | |
} | |
#endregion | |
#region Space Transformations | |
/// <summary> | |
/// Scales the position around the pivot with scale | |
/// </summary> | |
public static Vector2 Scale (Vector2 pos, Vector2 pivot, Vector2 scale) | |
{ | |
return Vector2.Scale (pos - pivot, scale) + pivot; | |
} | |
/// <summary> | |
/// Scales the rect around the pivot with scale | |
/// </summary> | |
public static Rect Scale (Rect rect, Vector2 pivot, Vector2 scale) | |
{ | |
rect.position = Vector2.Scale (rect.position - pivot, scale) + pivot; | |
rect.size = Vector2.Scale (rect.size, scale); | |
return rect; | |
} | |
/// <summary> | |
/// Transforms the position from the space aquired with BeginNoClip or MoveClipsUp to it's previous space | |
/// </summary> | |
public static Vector2 ScaledToGUISpace (Vector2 scaledPosition) | |
{ | |
if (rectStackGroups == null || rectStackGroups.Count == 0) | |
return scaledPosition; | |
// Iterate through the clips and substract positions | |
List<Rect> rectStackGroup = rectStackGroups[rectStackGroups.Count-1]; | |
for (int clipCnt = 0; clipCnt < rectStackGroup.Count; clipCnt++) | |
scaledPosition -= rectStackGroup[clipCnt].position; | |
return scaledPosition; | |
} | |
/// <summary> | |
/// Transforms the rect from the space aquired with BeginNoClip or MoveClipsUp to it's previous space. | |
/// DOES NOT scale the rect, only offsets it! | |
/// </summary> | |
public static Rect ScaledToGUISpace (Rect scaledRect) | |
{ | |
if (rectStackGroups == null || rectStackGroups.Count == 0) | |
return scaledRect; | |
scaledRect.position = ScaledToGUISpace (scaledRect.position); | |
return scaledRect; | |
} | |
/// <summary> | |
/// Transforms the position to the new space aquired with BeginNoClip or MoveClipsUp | |
/// It's way faster to call GUIToScreenSpace before modifying the space though! | |
/// </summary> | |
public static Vector2 GUIToScaledSpace (Vector2 guiPosition) | |
{ | |
if (rectStackGroups == null || rectStackGroups.Count == 0) | |
return guiPosition; | |
// Iterate through the clips and add positions ontop | |
List<Rect> rectStackGroup = rectStackGroups[rectStackGroups.Count-1]; | |
for (int clipCnt = 0; clipCnt < rectStackGroup.Count; clipCnt++) | |
guiPosition += rectStackGroup[clipCnt].position; | |
return guiPosition; | |
} | |
/// <summary> | |
/// Transforms the rect to the new space aquired with BeginNoClip or MoveClipsUp. | |
/// DOES NOT scale the rect, only offsets it! | |
/// It's way faster to call GUIToScreenSpace before modifying the space though! | |
/// </summary> | |
public static Rect GUIToScaledSpace (Rect guiRect) | |
{ | |
if (rectStackGroups == null || rectStackGroups.Count == 0) | |
return guiRect; | |
guiRect.position = GUIToScaledSpace (guiRect.position); | |
return guiRect; | |
} | |
/// <summary> | |
/// Transforms the position to screen space. | |
/// You can use GUIToScaledSpace when you want to transform an old rect to the new space aquired with BeginNoClip or MoveClipsUp (slower, try to call this function before any of these two)! | |
/// ATTENTION: This does not work well when any of the top groups is negative, means extends to the top or left of the screen. You may consider to use GUIToScaledSpace then, if possible! | |
/// </summary> | |
public static Vector2 GUIToScreenSpace (Vector2 guiPosition) | |
{ | |
#if UNITY_EDITOR | |
if (isEditorWindow) | |
return guiPosition + getTopRectScreenSpace.position - new Vector2 (0, 22); | |
#endif | |
return guiPosition + getTopRectScreenSpace.position; | |
} | |
/// <summary> | |
/// Transforms the rect to screen space. | |
/// You can use GUIToScaledSpace when you want to transform an old rect to the new space aquired with BeginNoClip or MoveClipsUp (slower, try to call this function before any of these two)! | |
/// ATTENTION: This does not work well when any of the top groups is negative, means extends to the top or left of the screen. You may consider to use GUIToScaledSpace then, if possible! | |
/// </summary> | |
public static Rect GUIToScreenSpace (Rect guiRect) | |
{ | |
guiRect.position += getTopRectScreenSpace.position; | |
#if UNITY_EDITOR | |
if (isEditorWindow) | |
guiRect.y -= 22; | |
#endif | |
return guiRect; | |
} | |
#endregion | |
} | |
} |
@brnkhy Sorry didn't get a notification.
Hm can you show me parts of your code? Maybe you didn't apply the panZoomAdjust offset value? Does this happen when you zoom out or in?
I can assure you it works for my node editor, would notice if there's something off :)
Seneral
@Seneral Hey! Yes I checked your version as well and it works fine as you said. This happens in both zoom in&out for me. I think "panZoomAdjust" might be the case as I don't even remember that. You can find the editor window here; https://github.com/mapbox/mapbox-unity-sdk/blob/nodeEditor/sdkproject/Assets/Editor/NodeEditor/NodeBasedEditor.cs
Yes, you missed it. In line 126, GUIScaleUtility.BeginScale returns a Vector2 you need to apply to your scaled content (nodes in your case). It changes every frame depending on zoom so you'd need to save it and apply during drawing along with _panDelta :) This is requried due to the way the Clipping rects are scaled and moved aswell as the custom origin...
Seneral
@Seneral
Ah no idea how I missed it. It's much better now. Still having issues, I think with very low/high values but at least it's much more subtle now and functionality in general is quite usable.
Again would love to hear if you can think of anything else on top of your head. 🙏
@brnkhy Hey, sorry again got no notification... Just randomly remembered and checked.
Really, everything should work just fine if correctly applied. You looked into my node editor to see how it works there?
If nothing helps, can you send me your project (code)? Might be a bug or simply edge case I don't know of...
Seneral
@Seneral , no worries I'm checking randomly as well.
You can find the code here; https://github.com/mapbox/mapbox-unity-sdk/tree/nodeEditorFolderRestructure
It would be great if you can have a look as I really would love to be able to use your class instead of learning and writing my own (I'm just learning editor coding so trying to reuse as much as I can). Structure is different (but simpler) than your own so I totally understand if you don't want to or not have time etc.
You'll probably notice right away but I'm drawing all nodes in recursive and applying a base pan and scale delta you suggested.
I think my main problem is that I don't understand what blocks drawing node in that left edge in those cases for example. I mean considering that the problem is in my Draw function, it's not like I'm calculating/using a window area rect in there. So I feel like maybe I'm passing wrong parameters to BeginScale function?
Hey @Seneral,
I came across this snippet when toying around with a node editor myself, and finding that GUI.matrix can draw over tabs. Anyway, I ended up creating a method that was effectively equivalent to your BeginNoClip()
, but then added GUI.BeginClip(Rect position, Vector2 scrollOffset, Vector2 renderOffset, bool resetOffset)
, call at the bottom. This made it clip again, but in ways that we want it to (i.e. limits it to a given rect in EditorWindow space, and prevents the contents from being drawn over the editor's tabs).
The nitty-gritty important code being:
// Top of BeginNoClip
Rect inverseRect = new Rect(GetTopRect().position + clippingRect.position, clippingRect.width)
inverseRect.position = matrix.inverse * new Vector4(inverseRect.position.x, inverseRect.position.y, 0, 1);
inverseRect.width /= matrix.m00;
inverseRect.height /= matrix.m11;
// ...
// End of BeginNoClip
GUI.BeginClip(inverseRect, -inverseRect.position, Vector2.zero, false);
Where clippingRect
is the Rect in EditorWindow space you want to limit your region to, and matrix
is the GUI.matrix
within that region.
This not only removes the excess clipping when scaling and translating GUI.matrix, it also uses the first GetTopRect()
call to keep the tab room clear of any draws, and allows the pan/translate to be respected (caused by setting -inverseRect.position
). By doing this, I haven't needed to use any methods similar to those in you Space Transformations code region.
I haven't tested it for nested calls, so there might be a few matrix multiplications to sprinkle in there. Anyway, thought you might be interested. If so, feel free to message back and I can upload a full copy of what I'm using.
Damn, hate that I get no notifications whatsoever for Gist...
Looks really interesting, may be the final step to a truly unintrusive approach - thanks very much!
Btw, had to fix an error for 2018, changed stuff, so need to update this snippet accordingly. Next time I have time I will add both.
Thanks again!
^^ Update on this: Implemented a similar thing to @mderu's suggestion here, using the GUI.BeginClip scrollOffset instead of a custom zoomPosAdjust, but this makes the problem with popups even worse - since they seem to not only ignore scale, but also that scrollOffset. Which means even in scale 1 popups (EnumPopup) will be offset from the actual control - with zoomPosAdjust (since that is a stanard offset) it at least works on scale 1.
Currently, there's no way to fix these popups, since GUI.matrix is essentially the only way to implement proper scaling and any scaled popups simply won't work.
Hey man do you have any examples on how to use this? currently adjustedGUILayout is always null even though it is initialized. Would really love to see this working as its hard to find a solution on this
Sure, I'm using it in my Node Editor Framework.
Initial call here.
The zoomPosAdjust is later used on all normally placed components here.
AdjustedGUILayout is not used in the framework though, but setting that flag basically does GUILayout.Space to offset all layout components in a similar fashion as I manually do with zoomPosAdjust in the framework.
What do you mean by adjustedGUILayout being null though? Do you mean the list in the script? Generally you shouldn't need to access it from outside
I'm not calling it from the outside, it seems to be null when I call BeginScale.
I'm trying to only scale a part of my window ( an GUILayout Scrollview with a GUI scrollview inside)
Here's the code I used to test your script out, which pops the error
private void DrawNodeView() { GUIScaleUtility.CheckInit(); var x = HorizontalSplitView.View2Rect; var adjust = GUIScaleUtility.BeginScale(ref x, HorizontalSplitView.View2Rect.center, 1f, true, false); var rect = HorizontalSplitView.View2Rect; rect.position = adjust; NodeViewScrollPos = GUI.BeginScrollView(rect, NodeViewScrollPos, NodeViewRect, NodeViewScrollbarStyle, NodeViewScrollbarStyle); DrawConnections(); DrawNodes(); GUIScaleUtility.EndScale(); }
Bump!!
Great script @Seneral , it was unusable on 2021.2.7 due to internal changes.
Using the method info of get_topmostRect
with BindingFlags.Static | BindingFlags.NonPublic
instead of using PropertyInfo did the trick.
Ln52:
from PropertyInfo topmostRect = GUIClipType.GetProperty ("topmostRect", BindingFlags.Static | BindingFlags.Public);
to MethodInfo topmostRect = GUIClipType.GetMethod("get_topmostRect", BindingFlags.Static | BindingFlags.NonPublic);
Ln67:
from topmostRectDelegate = (Func<Rect>)Delegate.CreateDelegate (typeof(Func<Rect>), topmostRect.GetGetMethod ());
to topmostRectDelegate = (Func<Rect>)Delegate.CreateDelegate(typeof(Func<Rect>), topmostRect);
I hope you update it since it was a life saver for me, and surely for others!
Thanks! Yeah I haven't used unity in years, so I have not been a good maintainer for a while now. Sorry about that.
I assume that change came with 2021 or just 2021.2?
That would influence which preprocessor check we'd need to not break compability, so if you already know that'd be great
@MRKDaGods Forgot you need to ping
@Seneral I have spent the past hour looking through the CsReference branches, and it was hell of a time actually since GUIClip has been moving around.
Your old code should work for any version prior to 2018.2 (< 2018.2
).
My fix should work for >= 2018.2
.
Ok, that gave me the hint, it's already fixed (I think?) in my framework, I just forgot about it.
https://github.com/Seneral/Node_Editor_Framework/blob/develop/Node_Editor_Framework/Runtime/Utilities/GUI/GUIScaleUtility.cs#L65
It is a different fix though, so yours seems more explicit, and I don't see how the code above even adapts to the changes you mentioned.
Sadly I can't verify it myself rn, very busy, so I just gotta rely on reports.
Thanks!
Hey @Seneral,
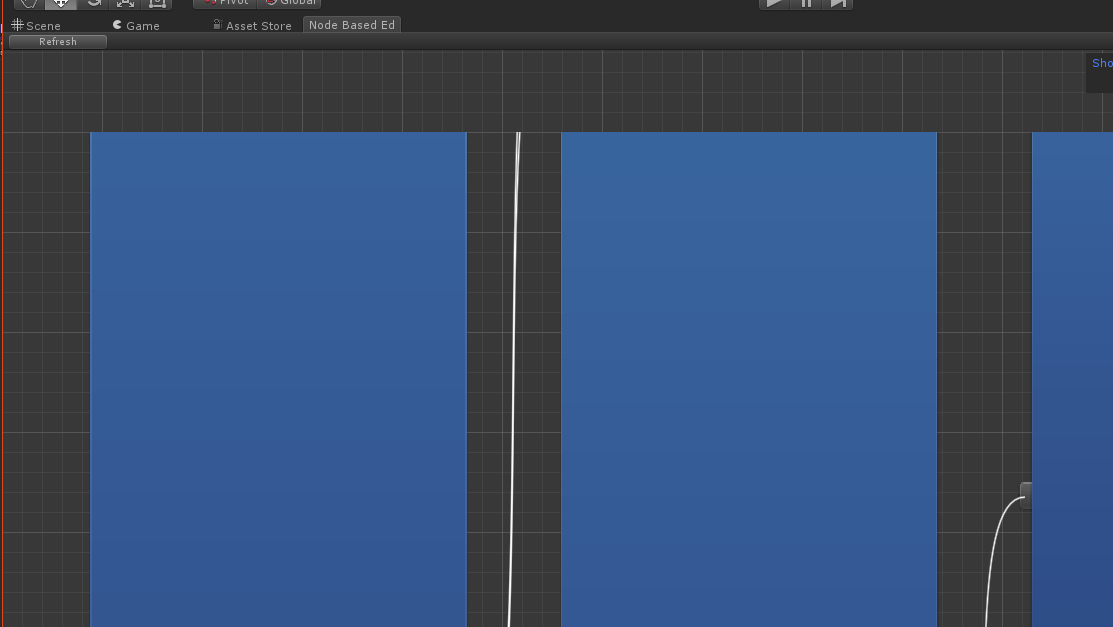
I'm having a clipping issue at top left using this scipt, any quick ideas what might be wrong?