Last active
February 1, 2023 15:41
-
-
Save Sharlottes/b2332b88695d11686dab5b9248c433da to your computer and use it in GitHub Desktop.
Beackjoon Node.js quick-tester script
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
const fs = require("fs"); | |
const HJSON = require("hjson"); | |
const path = require("path"); | |
const childProcess = require("child_process"); | |
const rl = require("readline"); | |
const rlI = rl.createInterface(process.stdin, process.stdout); | |
const options = (() => { | |
const defaults = { | |
codePath: process.argv[2], | |
testCaseMode: "", | |
}; | |
const flagType = [ | |
[ | |
"testCase", | |
"tc", | |
(value) => (defaults.testCaseMode = value || "./testcase.hjson"), | |
], | |
]; | |
for (const param of process.argv.slice(3)) { | |
for (const [long, short, setter] of flagType) { | |
const [key, value] = param.split(":"); | |
if ( | |
key.start == `--${long}` || | |
key.toUpperCase() == `-${short.toUpperCase()}` | |
) { | |
setter(value); | |
break; | |
} | |
} | |
} | |
return defaults; | |
})(); | |
const cachedTestcasePath = options.testCaseMode; | |
const asyncQuestion = async (query) => | |
new Promise((res) => rlI.question(query, res)); | |
async function forkProcess(line) { | |
return new Promise((res, rej) => { | |
const stack = []; | |
const childPros = childProcess | |
.fork(options.codePath, { stdio: "pipe" }) | |
.on("error", rej) | |
.on("exit", res); | |
childPros.stdio[1] | |
.setEncoding("utf-8") | |
.on("data", (data) => stack.push(data)) | |
.on("close", () => { | |
console.log(stack.join("")); | |
childPros.kill(); | |
res(); | |
}); | |
const lineHandler = (line) => { | |
stack.push(line + "\n-----------\n"); | |
childPros.stdio[0].write(line); | |
childPros.stdio[0].end(); | |
}; | |
if (options.testCaseMode) lineHandler(line); | |
else rlI.once("line", lineHandler); | |
}); | |
} | |
async function runCode() { | |
return new Promise(async (res, rej) => { | |
if (!options.testCaseMode) forkProcess().then(res).catch(rej); | |
else { | |
const testCases = await new Promise((res) => | |
fs.readFile(path.join(__dirname, options.testCaseMode), {}, (e, d) => | |
res(d) | |
) | |
).then((buffer) => Array.from(HJSON.parse(buffer.toString()))); | |
console.log( | |
`${testCases.length}개의 테스트 케이스 발견! 모두 동시에 실행됩니다...` | |
); | |
for (const line of testCases) await forkProcess(line).catch(rej); | |
res(); | |
} | |
}); | |
} | |
const start = async () => { | |
console.log( | |
" Node.js 백준 문제 테스터 " + "\n=================================" | |
); | |
while (true) { | |
await runCode().catch((e) => console.log("실패!, ", e)); | |
if (options.testCaseMode) { | |
await asyncQuestion( | |
"테스트 케이스를 계속 진행할까요? [Y,y / N,n] (기본 Y)" | |
).then((res) => { | |
if (res.toUpperCase() == "N") options.testCaseMode = ""; | |
}); | |
} else | |
await asyncQuestion( | |
"아무 키를 누르세요... (테스트 케이스 계속하기: [T])" | |
).then((res) => { | |
if (res.toUpperCase() == "T") options.testCaseMode = cachedTestcasePath; | |
}); | |
} | |
}; | |
start(); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Baekjoon.js quick tester script
very simple non-stop node.js I/O tester
motivation
typing
node [scriptname]
every time testing is very tired and wasteful for me. i thought i need more faster and comfortable test-case tester forwho don't know debuggersome pained developers.How To Start
create package.json
first of all, you need to create
package.json
for better quick testing.init packages
simple. just run
yarn
ornpm i
run test
npm run qt <target script> [-tc | -TC | --testCase[:path = ./testcase.json]]
or
yarn qt <target script> [-tc | -TC | --testCase[:path = ./testcase.json]]
ex)
yarn qt 1000 -tc
at least project directory is this.
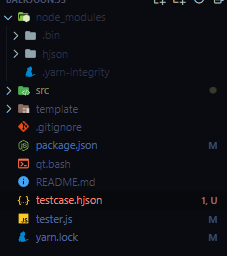
yeah.... project setting is very complex and lazyness sometimes. i will make generator module as possible as soon :3stop test
there isn't special keycode to exit in the script. juse use node.js' exit code -
Ctrl + C
Options - testcase.hjson
i'm also tired at typing same test inputs every time testing.
tester.js will automatically test codes with given testcases.json data.
testcase format is also very simple,
check hjson syntex more in tryout
why HJSON?
because it's very good when typing multiple-line testcases.