|
/* |
|
This is utility to that provides converter to convert open3d::geometry::PointCloud and pcl::PointCloud<T>. |
|
|
|
// Point Type (pcl::PointXYZ, pcl::PointNormal, pcl::PointXYZRGB, pcl::PointXYZRGBA, pcl::PointXYZRGBNormal) |
|
typedef pcl::PointXYZRGB PointType; |
|
|
|
// Convert Point Cloud To Open3D From PCL |
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = convert::to_open3d<PointType>( pcl_cloud ); |
|
|
|
// Convert Point Cloud To PCL From Open3D |
|
pcl::PointCloud<PointType>::Ptr pcl_cloud = convert::to_pcl<PointType>( open3d_cloud ); |
|
|
|
Copyright (c) 2020 Tsukasa Sugiura <t.sugiura0204@gmail.com> |
|
Licensed under the MIT license. |
|
|
|
Permission is hereby granted, free of charge, to any person obtaining a copy |
|
of this software and associated documentation files (the "Software"), to deal |
|
in the Software without restriction, including without limitation the rights |
|
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
|
copies of the Software, and to permit persons to whom the Software is |
|
furnished to do so, subject to the following conditions: |
|
|
|
The above copyright notice and this permission notice shall be included in all |
|
copies or substantial portions of the Software. |
|
|
|
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
|
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
|
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
|
SOFTWARE. |
|
*/ |
|
|
|
#ifndef __CONVERT__ |
|
#define __CONVERT__ |
|
|
|
#include <vector> |
|
#include <memory> |
|
|
|
#define NOMINMAX |
|
#include <Open3D/Open3D.h> |
|
#include <pcl/point_cloud.h> |
|
#include <pcl/point_types.h> |
|
|
|
#include <Eigen/Core> |
|
#include <Eigen/Geometry> |
|
|
|
namespace convert |
|
{ |
|
template<typename T> |
|
std::shared_ptr<pcl::PointCloud<T>> to_pcl( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
pcl::PointCloud<T>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<T>>(); |
|
return pcl_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<pcl::PointCloud<pcl::PointXYZ>> to_pcl<pcl::PointXYZ>( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
const uint32_t size = open3d_cloud->points_.size(); |
|
|
|
pcl::PointCloud<pcl::PointXYZ>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<pcl::PointXYZ>>(); |
|
pcl_cloud->width = size; |
|
pcl_cloud->height = 1; |
|
pcl_cloud->is_dense = false; |
|
pcl_cloud->points.resize( size ); |
|
|
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
} |
|
|
|
return pcl_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<pcl::PointCloud<pcl::PointNormal>> to_pcl<pcl::PointNormal>( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
const uint32_t size = open3d_cloud->points_.size(); |
|
const Eigen::Vector3f zero = Eigen::Vector3f::Zero(); |
|
|
|
pcl::PointCloud<pcl::PointNormal>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<pcl::PointNormal>>(); |
|
pcl_cloud->width = size; |
|
pcl_cloud->height = 1; |
|
pcl_cloud->is_dense = false; |
|
pcl_cloud->points.resize( size ); |
|
|
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
const Eigen::Vector3f normal = ( open3d_cloud->HasNormals() ) ? open3d_cloud->normals_[i].cast<float>() : zero; |
|
std::copy( normal.data(), normal.data() + normal.size(), pcl_cloud->points[i].normal ); |
|
} |
|
|
|
return pcl_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGB>> to_pcl<pcl::PointXYZRGB>( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
const uint32_t size = open3d_cloud->points_.size(); |
|
|
|
pcl::PointCloud<pcl::PointXYZRGB>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<pcl::PointXYZRGB>>(); |
|
pcl_cloud->width = size; |
|
pcl_cloud->height = 1; |
|
pcl_cloud->is_dense = false; |
|
pcl_cloud->points.resize( size ); |
|
|
|
if( open3d_cloud->HasColors() ){ |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
const auto color = ( open3d_cloud->colors_[i] * 255.0 ).cast<uint32_t>(); |
|
uint32_t rgb = color[0] << 16 | color[1] << 8 | color[2]; |
|
pcl_cloud->points[i].rgb = *reinterpret_cast<float*>( &rgb ); |
|
} |
|
} |
|
else{ |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
uint32_t rgb = 0x000000; |
|
pcl_cloud->points[i].rgb = *reinterpret_cast<float*>( &rgb ); |
|
} |
|
} |
|
|
|
return pcl_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGBA>> to_pcl<pcl::PointXYZRGBA>( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
const uint32_t size = open3d_cloud->points_.size(); |
|
|
|
pcl::PointCloud<pcl::PointXYZRGBA>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<pcl::PointXYZRGBA>>(); |
|
pcl_cloud->width = size; |
|
pcl_cloud->height = 1; |
|
pcl_cloud->is_dense = false; |
|
pcl_cloud->points.resize( size ); |
|
|
|
if( open3d_cloud->HasColors() ){ |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
const auto color = ( open3d_cloud->colors_[i] * 255.0 ).cast<uint32_t>(); |
|
pcl_cloud->points[i].rgba = 0xff000000 | color[0] << 16 | color[1] << 8 | color[2]; |
|
} |
|
} |
|
else{ |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
pcl_cloud->points[i].rgba = 0xff000000; |
|
} |
|
} |
|
|
|
return pcl_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGBNormal>> to_pcl<pcl::PointXYZRGBNormal>( const std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud ) |
|
{ |
|
const uint32_t size = open3d_cloud->points_.size(); |
|
const Eigen::Vector3f zero = Eigen::Vector3f::Zero(); |
|
|
|
pcl::PointCloud<pcl::PointXYZRGBNormal>::Ptr pcl_cloud = pcl::make_shared<pcl::PointCloud<pcl::PointXYZRGBNormal>>(); |
|
pcl_cloud->width = size; |
|
pcl_cloud->height = 1; |
|
pcl_cloud->is_dense = false; |
|
pcl_cloud->points.resize( size ); |
|
|
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
pcl_cloud->points[i].getVector3fMap() = open3d_cloud->points_[i].cast<float>(); |
|
const auto color = ( open3d_cloud->colors_[i] * 255.0 ).cast<uint32_t>(); |
|
uint32_t rgb = ( open3d_cloud->HasColors() ) ? color[0] << 16 | color[1] << 8 | color[2] : 0x000000; |
|
pcl_cloud->points[i].rgb = *reinterpret_cast<float*>( &rgb ); |
|
const Eigen::Vector3f normal = ( open3d_cloud->HasNormals() ) ? open3d_cloud->normals_[i].cast<float>() : zero; |
|
std::copy( normal.data(), normal.data() + normal.size(), pcl_cloud->points[i].normal ); |
|
} |
|
|
|
return pcl_cloud; |
|
}; |
|
|
|
template<typename T> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d( const std::shared_ptr<pcl::PointCloud<T>> pcl_cloud ) |
|
{ |
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
return open3d_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d<pcl::PointXYZ>( const std::shared_ptr<pcl::PointCloud<pcl::PointXYZ>> pcl_cloud ) |
|
{ |
|
const uint32_t size = pcl_cloud->size(); |
|
|
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
open3d_cloud->points_.resize( size ); |
|
|
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
open3d_cloud->points_[i] = pcl_cloud->points[i].getVector3fMap().cast<double>(); |
|
} |
|
|
|
return open3d_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d<pcl::PointNormal>( const std::shared_ptr<pcl::PointCloud<pcl::PointNormal>> pcl_cloud ) |
|
{ |
|
const uint32_t size = pcl_cloud->size(); |
|
|
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
open3d_cloud->points_.resize( size ); |
|
open3d_cloud->normals_.resize( size ); |
|
|
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
open3d_cloud->points_[i] = pcl_cloud->points[i].getVector3fMap().cast<double>(); |
|
open3d_cloud->normals_[i] = pcl_cloud->points[i].getNormalVector3fMap().cast<double>(); |
|
} |
|
|
|
return open3d_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d<pcl::PointXYZRGB>( const std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGB>> pcl_cloud ) |
|
{ |
|
const uint32_t size = pcl_cloud->size(); |
|
|
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
open3d_cloud->points_.resize( size ); |
|
open3d_cloud->colors_.resize( size ); |
|
|
|
constexpr double normal = 1.0 / 255.0; |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
open3d_cloud->points_[i] = pcl_cloud->points[i].getVector3fMap().cast<double>(); |
|
const uint32_t color = *reinterpret_cast<uint32_t*>( &pcl_cloud->points[i].rgb ); |
|
open3d_cloud->colors_[i] = Eigen::Vector3d( ( color >> 16 ) & 0x0000ff, ( color >> 8 ) & 0x0000ff, color & 0x0000ff ) * normal; |
|
} |
|
|
|
return open3d_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d<pcl::PointXYZRGBA>( const std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGBA>> pcl_cloud ) |
|
{ |
|
const uint32_t size = pcl_cloud->size(); |
|
|
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
open3d_cloud->points_.resize( size ); |
|
open3d_cloud->colors_.resize( size ); |
|
|
|
constexpr double normal = 1.0 / 255.0; |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
open3d_cloud->points_[i] = pcl_cloud->points[i].getVector3fMap().cast<double>(); |
|
const uint32_t color = pcl_cloud->points[i].rgba; |
|
open3d_cloud->colors_[i] = Eigen::Vector3d( ( color >> 16 ) & 0x000000ff, ( color >> 8 ) & 0x000000ff, color & 0x000000ff ) * normal; |
|
} |
|
|
|
return open3d_cloud; |
|
}; |
|
|
|
template<> |
|
std::shared_ptr<open3d::geometry::PointCloud> to_open3d<pcl::PointXYZRGBNormal>( const std::shared_ptr<pcl::PointCloud<pcl::PointXYZRGBNormal>> pcl_cloud ) |
|
{ |
|
const uint32_t size = pcl_cloud->size(); |
|
|
|
std::shared_ptr<open3d::geometry::PointCloud> open3d_cloud = std::make_shared<open3d::geometry::PointCloud>(); |
|
open3d_cloud->points_.resize( size ); |
|
open3d_cloud->normals_.resize( size ); |
|
open3d_cloud->colors_.resize( size ); |
|
|
|
constexpr double normal = 1.0 / 255.0; |
|
#pragma omp parallel for |
|
for( int32_t i = 0; i < size; i++ ){ |
|
open3d_cloud->points_[i] = pcl_cloud->points[i].getVector3fMap().cast<double>(); |
|
open3d_cloud->normals_[i] = pcl_cloud->points[i].getNormalVector3fMap().cast<double>(); |
|
const uint32_t color = *reinterpret_cast<uint32_t*>( &pcl_cloud->points[i].rgb ); |
|
open3d_cloud->colors_[i] = Eigen::Vector3d( ( color >> 16 ) & 0x0000ff, ( color >> 8 ) & 0x0000ff, color & 0x0000ff ) * normal; |
|
} |
|
|
|
return open3d_cloud; |
|
}; |
|
} |
|
|
|
#endif // __CONVERT__ |
Hello,
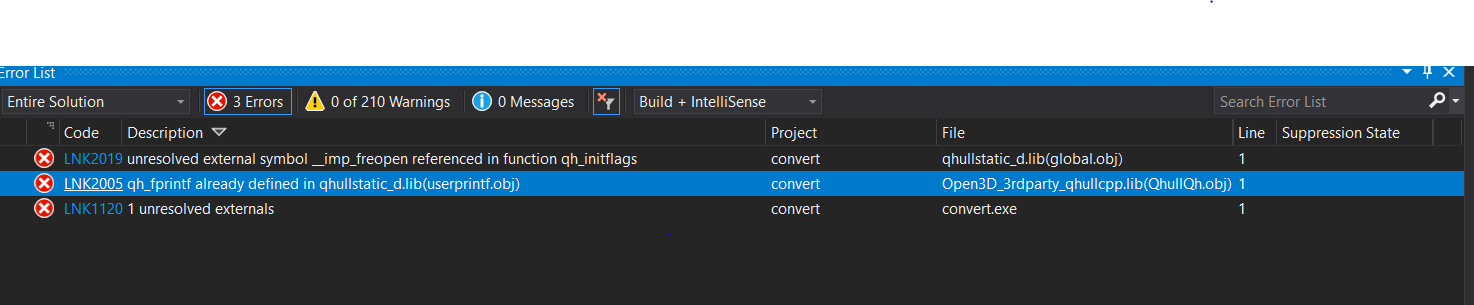
I'm trying to build this project in Visual Studio 2019. I'm having these three errors. Can you please help me solve these? Thank you!