-
-
Save XerxesZorgon/5205facbb7c7ef02999e27ead70a5d59 to your computer and use it in GitHub Desktop.
#= | |
Plot Julia functions | |
Load with: include("JuliaSetPlots.jl") | |
Written by: John Peach 13-Feb-2022 | |
Wild Peaches | |
Julia sets are defined as | |
z_{n+1} = zₙ² + c | |
where zₙ ∈ ℂ and c = x₀ + iy₀ | |
Mandelbrot sets are similar, but z₀ is fixed, and c ∈ ℂ. | |
=# | |
# Required packages | |
using Plots, LinearAlgebra | |
gr() | |
""" | |
plotIterates | |
Plots iteratations of the Julia function from in initial point z₀ ∈ ℂ and parameter c | |
Parameters | |
z₀: Starting point | |
c: Control parameter | |
n: Number of iteratations | |
Returns | |
Plot of n iteratations | |
""" | |
function plotIterates(z₀,c,n) | |
# Initialize trajectory | |
zₙ = length(z₀) | |
traj = zeros(ComplexF64,n,zₙ) | |
traj[1,:] .= z₀ | |
# Generate trajectory | |
for k = 1:zₙ | |
for j = 2:n | |
traj[j,k] = traj[j-1,k]^2 + c | |
end | |
end | |
# Plot trajectory. The display function is needed inside a function. | |
crvLabel = Array{String}(undef,zₙ) | |
for k = 1:zₙ | |
crvLabel[k] = string("z₀ = ", z₀[k]) | |
end | |
# Note permation of label string. | |
# Ref: https://discourse.julialang.org/t/transpose-of-an-array-of-strings/40431/8 | |
crvPlot = plot(traj, | |
linewidth = 3, | |
aspect_ratio = 1, | |
xlabel = "Real axis", | |
ylabel = "Imag axis", | |
title = "Julia Iterates", | |
arrow = :arrow) | |
#label = permutedims(crvLabel) | |
#legend = :outertopright) | |
display(crvPlot) | |
# Return trajectory | |
return traj | |
end | |
""" | |
plotJulia | |
Plots points in a Julia set | |
Parameters | |
c: Constant added to each iterate | |
""" | |
function plotJulia(c,xRng = [-1.6,1.6], yRng = [-1.6, 1.6]) | |
# Array of iteration counts | |
nx = 1281 | |
ny = 1281 | |
iterCounts = zeros(nx,ny) | |
# Locations | |
xLocs = range(minimum(xRng),stop = maximum(xRng),length = nx) | |
yLocs = range(minimum(yRng),stop = maximum(yRng),length = ny) | |
# Loop over x,y locations iterating until maxIter reached, or |z|² > 4 | |
maxIters = 1000 | |
for j = 1:nx | |
for k = 1:ny | |
z = xLocs[j] + yLocs[k]im | |
while iterCounts[j,k] < maxIters && norm(z) < 2 | |
z = z^2 + c | |
iterCounts[j,k] += 1 | |
end | |
end | |
end | |
# Display Julia set as a heatmap | |
display(heatmap(iterCounts')) | |
# Return array of iterations | |
return iterCounts | |
end |
glanzkaiser,
Sorry you're having trouble with the code. I was able to run it from the Julia command window:
julia> c = -0.7269 + 0.1889im
julia> plotJulia(c);
I'm using version 1.7.2, but there probably isn't enough of a change to 1.7.3 to break the code. Maybe there's an issue with Jupyter Notebook?
John
HI @XerxesZorgon ,
it is quite dumb of me that I forget to call the function, too much on my plate
These are forgotten to plot it:
julia> c = -0.7269 + 0.1889im
julia> plotJulia(c);
I want to know why version upgrade can break old codes?
I'm using version 1.7.2, but there probably isn't enough of a change to 1.7.3 to break the code
I know an example linspace
can't be used and have to use range
instead. For science I think the basic does not change much. We learn calculus and it does not change that much for a very long time. Still plot Fractals the same right?
Hi John,
I read your posts the "The Growing Gap" and "Mathics and Nebo", great articles.. I believe that we will still depend on oil and gas till very long, no matter if we try to convert to renewable energy, all still needs fossil fuels. Politics, can be counted on why we still depend on oil and gas.
I am studying master degree "Mathematical Engineering" now in Erasmus Mundus program, in italy. It is very tight schedule, I hope I can manage to learn them all and pass the exams.
About Pluto, why is it slow? Why the creator name it the slowest moving planet? Even if it is not included as planet anymore currently, but next time we learn from this case that we shall name something with similar analogous meaning, name is a prayer I believe.
You are an amazing person, not only smart, but want to share your knowledge in your blog for free, I wish one day I have the chance to write article suitable for your blog and then I will show it to you, probably it can be published on your blog.
I have this imagination that your articles can be printed on leaflet like Rosary' paper that can be folded and back and forth it could contain 2 articles. Your articles is a science based that can be useful for the Economies, people will be more knowledgeable, I am from a poor country thus I know the most effective way to make a country to be rich is by the right education with morale value.
An example is if more people read your blog/articles there will be less uneducated people and therefore better country, less inequality, people be more productive and smarter.
About human kind, I feel pessimist today, I am in Europe now, and I still see a lot of negative impacts of overpopulation, throwing trashes randomly, even in London too. Are electric cars and rockets really going to be breakthroughs or only to showing Pride of some clans/nations. After trashing the earth therefore trashing Mars and Space?
Working at MIT is a great opportunity, I believe you worked hardly before and very intellectual that is why you are there right now, luck is when preparation meets opportunity. All the best.
To the author, I try using Jupyter Notebook with Julia 1.7.3 and
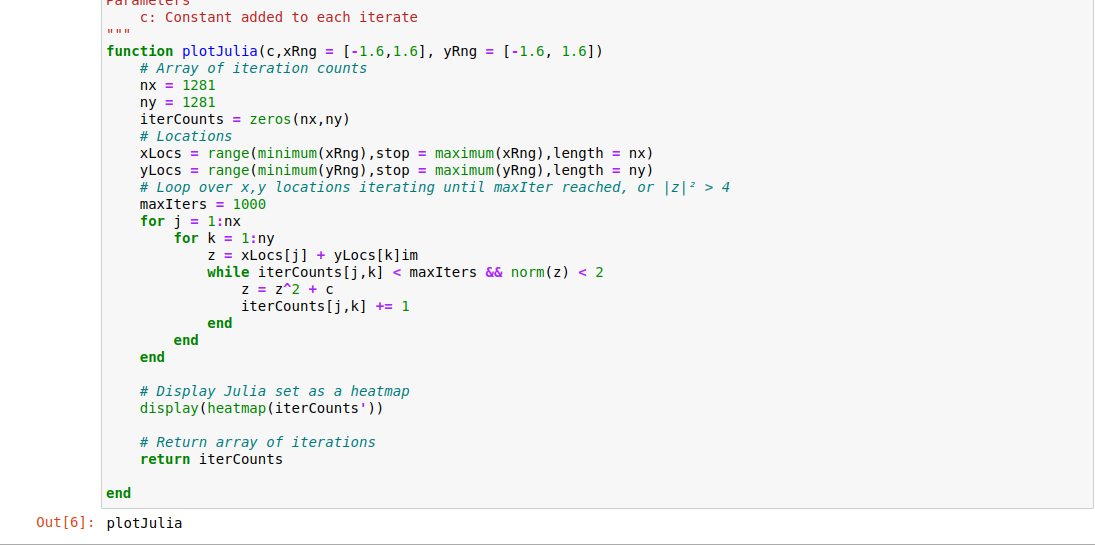
I can't obtain the plot of this, a great learning texts anyway. Thanks