Created
December 24, 2019 05:04
-
-
Save alexanderameye/fe56c473544d2491b6114a3f178020a0 to your computer and use it in GitHub Desktop.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
using UnityEngine; | |
using UnityEngine.Rendering; | |
using UnityEngine.Rendering.Universal; | |
public class OutlineFeature : ScriptableRendererFeature | |
{ | |
class OutlinePass : ScriptableRenderPass | |
{ | |
private RenderTargetIdentifier source { get; set; } | |
private RenderTargetHandle destination { get; set; } | |
public Material outlineMaterial = null; | |
RenderTargetHandle temporaryColorTexture; | |
public void Setup(RenderTargetIdentifier source, RenderTargetHandle destination) | |
{ | |
this.source = source; | |
this.destination = destination; | |
} | |
public OutlinePass(Material outlineMaterial) | |
{ | |
this.outlineMaterial = outlineMaterial; | |
} | |
// This method is called before executing the render pass. | |
// It can be used to configure render targets and their clear state. Also to create temporary render target textures. | |
// When empty this render pass will render to the active camera render target. | |
// You should never call CommandBuffer.SetRenderTarget. Instead call <c>ConfigureTarget</c> and <c>ConfigureClear</c>. | |
// The render pipeline will ensure target setup and clearing happens in an performance manner. | |
public override void Configure(CommandBuffer cmd, RenderTextureDescriptor cameraTextureDescriptor) | |
{ | |
} | |
// Here you can implement the rendering logic. | |
// Use <c>ScriptableRenderContext</c> to issue drawing commands or execute command buffers | |
// https://docs.unity3d.com/ScriptReference/Rendering.ScriptableRenderContext.html | |
// You don't have to call ScriptableRenderContext.submit, the render pipeline will call it at specific points in the pipeline. | |
public override void Execute(ScriptableRenderContext context, ref RenderingData renderingData) | |
{ | |
CommandBuffer cmd = CommandBufferPool.Get("_OutlinePass"); | |
RenderTextureDescriptor opaqueDescriptor = renderingData.cameraData.cameraTargetDescriptor; | |
opaqueDescriptor.depthBufferBits = 0; | |
if (destination == RenderTargetHandle.CameraTarget) | |
{ | |
cmd.GetTemporaryRT(temporaryColorTexture.id, opaqueDescriptor, FilterMode.Point); | |
Blit(cmd, source, temporaryColorTexture.Identifier(), outlineMaterial, 0); | |
Blit(cmd, temporaryColorTexture.Identifier(), source); | |
} | |
else Blit(cmd, source, destination.Identifier(), outlineMaterial, 0); | |
context.ExecuteCommandBuffer(cmd); | |
CommandBufferPool.Release(cmd); | |
} | |
/// Cleanup any allocated resources that were created during the execution of this render pass. | |
public override void FrameCleanup(CommandBuffer cmd) | |
{ | |
if (destination == RenderTargetHandle.CameraTarget) | |
cmd.ReleaseTemporaryRT(temporaryColorTexture.id); | |
} | |
} | |
[System.Serializable] | |
public class OutlineSettings | |
{ | |
public Material outlineMaterial = null; | |
} | |
public OutlineSettings settings = new OutlineSettings(); | |
OutlinePass outlinePass; | |
RenderTargetHandle outlineTexture; | |
public override void Create() | |
{ | |
outlinePass = new OutlinePass(settings.outlineMaterial); | |
outlinePass.renderPassEvent = RenderPassEvent.AfterRenderingTransparents; | |
outlineTexture.Init("_OutlineTexture"); | |
} | |
// Here you can inject one or multiple render passes in the renderer. | |
// This method is called when setting up the renderer once per-camera. | |
public override void AddRenderPasses(ScriptableRenderer renderer, ref RenderingData renderingData) | |
{ | |
if (settings.outlineMaterial == null) | |
{ | |
Debug.LogWarningFormat("Missing Outline Material"); | |
return; | |
} | |
outlinePass.Setup(renderer.cameraColorTarget, RenderTargetHandle.CameraTarget); | |
renderer.EnqueuePass(outlinePass); | |
} | |
} | |
The effect executes in material preview views, which can be annoying when editing materials. To prevent this, just add this block of code right after the AddRenderPasses
method declaration and before the material validation.
if (renderingData.cameraData.isPreviewCamera)
{
return;
}
Hay, thanks for this, however I am struggling to set this up from your post: https://alexanderameye.github.io/notes/edge-detection-outlines/
Can you provide the .shader file? Or even better example Unity project. I think this is useful as I really cannot find a decent URP outline shader.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello. First, I'm sorry to ask you a question on an old post. There is no comment function on the blog, so I post it here. The outline is visible even if the game object with the outline is behind the particle. Is there a way to solve it?
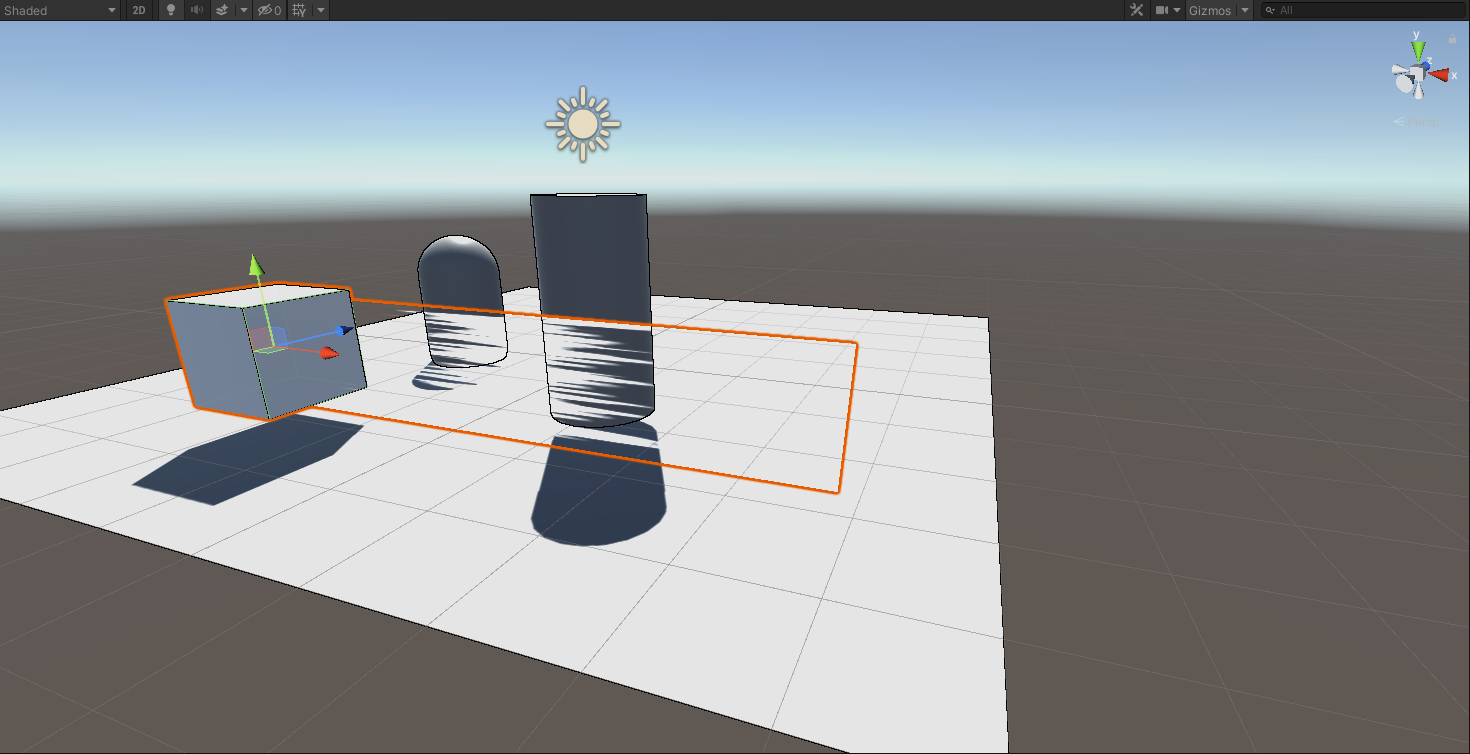
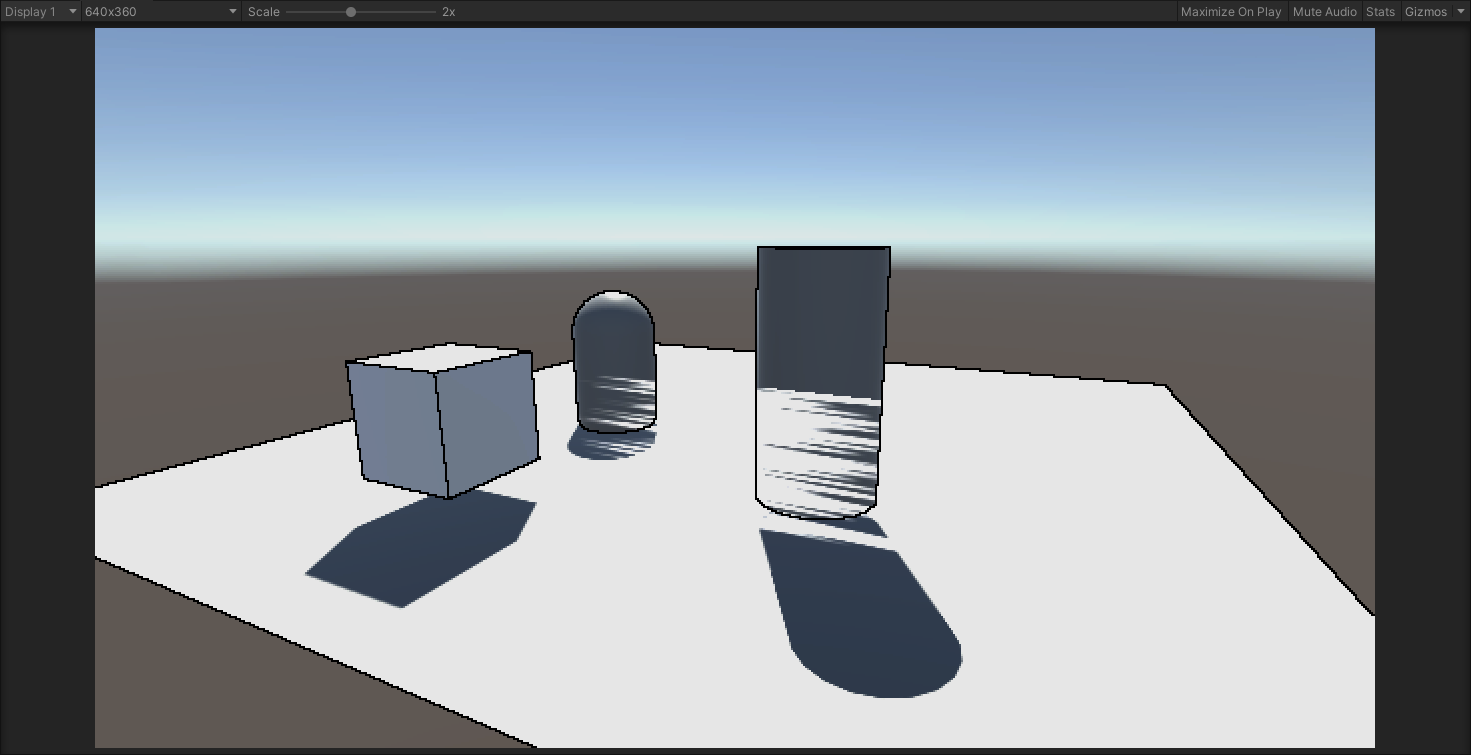