Created
October 28, 2015 05:40
-
-
Save alexanderkoumis/f2d0be69a5a062bc825e to your computer and use it in GitHub Desktop.
Python OpenCV C++ Wrapper (SWIG)
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
all: | |
swig -c++ -python opencv_facedetect.i | |
g++ -Wall -fPIC -c opencv_facedetect.cpp `pkg-config --cflags opencv` | |
g++ -Wall -fPIC -c opencv_facedetect_wrap.cxx `pkg-config --cflags python` | |
g++ -shared opencv_facedetect.o opencv_facedetect_wrap.o `pkg-config --libs opencv` `pkg-config --libs python` -o _opencv_facedetect.so | |
clean: | |
rm -f ./*.o $(PROG) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <iostream> | |
#include <vector> | |
#include <opencv2/core/core.hpp> | |
#include <opencv2/contrib/contrib.hpp> | |
#include <opencv2/objdetect/objdetect.hpp> | |
#include <opencv2/highgui/highgui.hpp> | |
#include <opencv2/imgproc/imgproc.hpp> | |
// #include <opencv2/gpu/gpu.hpp> | |
// Steps from http://docs.opencv.org/modules/gpu/doc/object_detection.html | |
int find_face(char* cascade_file, char* face_image) | |
{ | |
cv::CascadeClassifier cascade; | |
if(cascade.load(std::string(cascade_file))) | |
{ | |
cv::Mat frame = cv::imread(face_image); | |
cv::Mat frame_gray; | |
std::vector<cv::Rect> faces; | |
cv::cvtColor(frame, frame_gray, CV_BGR2GRAY); | |
cv::equalizeHist(frame_gray,frame_gray); | |
cascade.detectMultiScale( frame_gray, faces, 1.1, 2, 0 |CV_HAAR_SCALE_IMAGE, cv::Size(30, 30) ); | |
for(int i = 0; i < (int)faces.size(); ++i) | |
{ | |
cv::Point pt1 = faces[i].tl(); | |
cv::Size sz = faces[i].size(); | |
cv::Point pt2(pt1.x+sz.width, pt1.y+sz.height); | |
cv::rectangle(frame, pt1, pt2, cv::Scalar(255)); | |
} | |
cv::imshow("faces", frame); | |
cv::waitKey(0); | |
} | |
else | |
{ | |
std::cout << "Error: " << cascade_file << " is not a valid haarcascade file" << std::endl; | |
return 1; | |
} | |
return 0; | |
} | |
// // GPU version | |
// int find_face(char* cascade_file, char* face_image) | |
// { | |
// cv::gpu::CascadeClassifier_GPU cascade_gpu; | |
// int gpu_count = cv::gpu::getCudaEnabledDeviceCount(); // gpu_count >0 if CUDA device detected | |
// if(gpu_count == 0) | |
// { | |
// std::cout << "Error: No GPU" << std::endl; | |
// return 1; | |
// } | |
// if(cascade_gpu.load(std::string(cascade_file))) | |
// { | |
// cv::Mat frame = cv::imread(face_image); | |
// cv::gpu::GpuMat faces; | |
// cv::Mat frame_gray; | |
// cv::cvtColor(frame, frame_gray, CV_BGR2GRAY); | |
// cv::gpu::GpuMat gray_gpu(frame_gray); // copy the gray image to GPU memory | |
// cv::equalizeHist(frame_gray,frame_gray); | |
// int detect_num = cascade_gpu.detectMultiScale(gray_gpu, faces,1.2, 4, cv::Size(20, 20) ); | |
// cv::Mat obj_host; | |
// faces.colRange(0, detect_num).download(obj_host); // retrieve results from GPU | |
// cv::Rect* cfaces = obj_host.ptr<cv::Rect>(); // results are now in "obj_host" | |
// for(int i = 0; i < detect_num; ++i) | |
// { | |
// // Draw faces | |
// cv::Point pt1 = cfaces[i].tl(); | |
// cv::Size sz = cfaces[i].size(); | |
// cv::Point pt2(pt1.x+sz.width, pt1.y+sz.height); | |
// cv::rectangle(frame, pt1, pt2, cv::Scalar(255)); | |
// } | |
// cv::imshow("faces", frame); | |
// cv::waitKey(0); | |
// } | |
// } | |
// else | |
// { | |
// std::cout << "Error: " << cascade_file << " is not a valid haarcascade file" << std::endl; | |
// return 1; | |
// } | |
// return 0; | |
// } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
%module opencv_facedetect | |
%{ | |
extern int find_face(char* cascade_file, char* face_image); | |
%} | |
extern int find_face(char* cascade_file, char* face_image); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello I have followed all of your step. However in command
,I got an error which declared that 'cv' has not been declared. How can I fixed this?
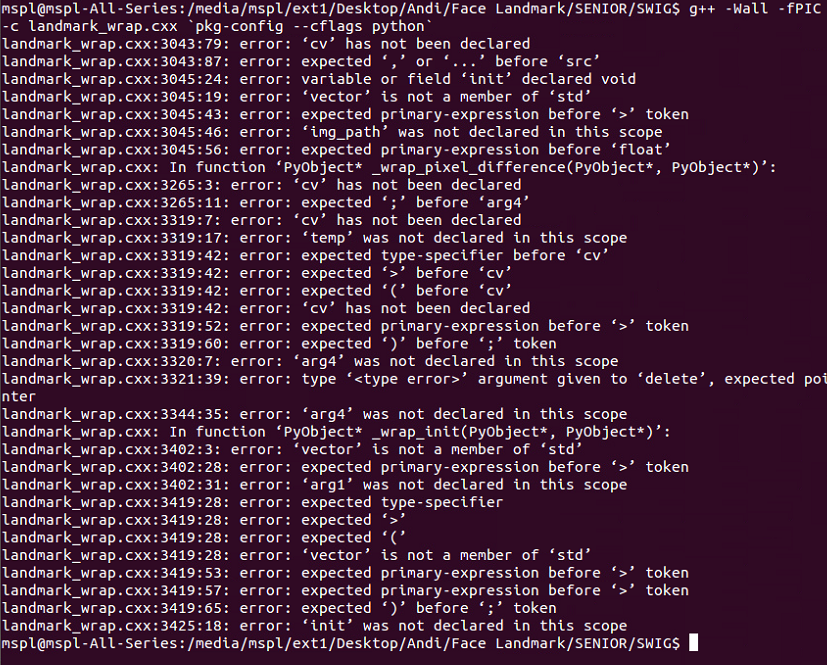
g++ -Wall -fPIC -c opencv_facedetect_wrap.cxx
pkg-config --cflags python