-
-
Save cooldil/0b2c5ee22befbbfcdefd06c9cf2b7a98 to your computer and use it in GitHub Desktop.
import requests, pickle | |
from datetime import datetime | |
import json, pytz | |
import pandas as pd | |
from influxdb import DataFrameClient | |
login_url = "https://monitoring.solaredge.com/solaredge-apigw/api/login" | |
panels_url = "https://monitoring.solaredge.com/solaredge-web/p/playbackData" | |
SOLAREDGE_USER = "" # web username | |
SOLAREDGE_PASS = "" # web password | |
SOLAREDGE_SITE_ID = "" # site id | |
DAILY_DATA = "4" | |
WEEKLY_DATA = "5" | |
INFLUXDB_IP = "" | |
INFLUXDB_PORT = 8086 | |
INFLUXDB_DATABASE = "" | |
INFLUXDB_SERIES = "optimisers" | |
INFLUXDB_RETENTION_POLICY = "autogen" | |
session = requests.session() | |
try: # Make sure the cookie file exists | |
with open('solaredge.cookies', 'rb') as f: | |
f.close() | |
except IOError: # Create the cookie file | |
session.post(login_url, headers = {"Content-Type": "application/x-www-form-urlencoded"}, data={"j_username": SOLAREDGE_USER, "j_password": SOLAREDGE_PASS}) | |
panels = session.post(panels_url, headers = {"Content-Type": "application/x-www-form-urlencoded", "X-CSRF-TOKEN": session.cookies["CSRF-TOKEN"]}, data={"fieldId": SOLAREDGE_SITE_ID, "timeUnit": DAILY_DATA}) | |
with open('solaredge.cookies', 'wb') as f: | |
pickle.dump(session.cookies, f) | |
f.close() | |
with open('solaredge.cookies', 'rb') as f: | |
session.cookies.update(pickle.load(f)) | |
panels = session.post(panels_url, headers = {"Content-Type": "application/x-www-form-urlencoded", "X-CSRF-TOKEN": session.cookies["CSRF-TOKEN"]}, data={"fieldId": SOLAREDGE_SITE_ID, "timeUnit": DAILY_DATA}) | |
if panels.status_code != 200: | |
session.post(login_url, headers = {"Content-Type": "application/x-www-form-urlencoded"}, data={"j_username": SOLAREDGE_USER, "j_password": SOLAREDGE_PASS}) | |
panels = session.post(panels_url, headers = {"Content-Type": "application/x-www-form-urlencoded", "X-CSRF-TOKEN": session.cookies["CSRF-TOKEN"]}, data={"fieldId": SOLAREDGE_SITE_ID, "timeUnit": DAILY_DATA}) | |
if panels.status_code != 200: | |
exit() | |
with open('solaredge.cookies', 'wb') as f: | |
pickle.dump(s.cookies, f) | |
response = panels.content.decode("utf-8").replace('\'', '"').replace('Array', '').replace('key', '"key"').replace('value', '"value"') | |
response = response.replace('timeUnit', '"timeUnit"').replace('fieldData', '"fieldData"').replace('reportersData', '"reportersData"') | |
response = json.loads(response) | |
data = {} | |
for date_str in response["reportersData"].keys(): | |
date = datetime.strptime(date_str, '%a %b %d %H:%M:%S GMT %Y') | |
date = pytz.timezone('Australia/Adelaide').localize(date).astimezone(pytz.utc) | |
for sid in response["reportersData"][date_str].keys(): | |
for entry in response["reportersData"][date_str][sid]: | |
if entry["key"] not in data.keys(): | |
data[entry["key"]] = {} | |
data[entry["key"]][date] = float(entry["value"].replace(",", "")) | |
df = pd.DataFrame(data) | |
conn = DataFrameClient(INFLUXDB_IP, INFLUXDB_PORT, "", "", INFLUXDB_DATABASE) | |
conn.write_points(df, INFLUXDB_SERIES, retention_policy=INFLUXDB_RETENTION_POLICY) |
Hi @cooldil I'm wondering whether you happen to have a list of API parameters that I could use alongside those that you programmed in this GIST. Or, could you confirm that these are the only ones?
Hi
I am wondering how to find this url on dashboard. Thanks!
panels_url = "https://monitoring.solaredge.com/solaredge-web/p/playbackData"
Hi I am wondering how to find this url on dashboard. Thanks! panels_url = "https://monitoring.solaredge.com/solaredge-web/p/playbackData"
The URL you referenced is called when you change over to the Layout area and then click on "Show playback". This brings up a "slider view" where you can see what the output of each of your panels was for any given 15 minute interval.
Thanks for your reply. It works well for me!
Another question, it seems that we are only able to retrive data on current day. Cause the "Show playback" can't display historical daily data. Am I right? And do you have any idea to retrive historical panel data. Thanks.
the ouptput dataframe's column names are be like:
197469474 | 197469507 | 197469573 | 197469606
I am wondering how to match it with the module number on the dashboard. Thanks again!
Hi
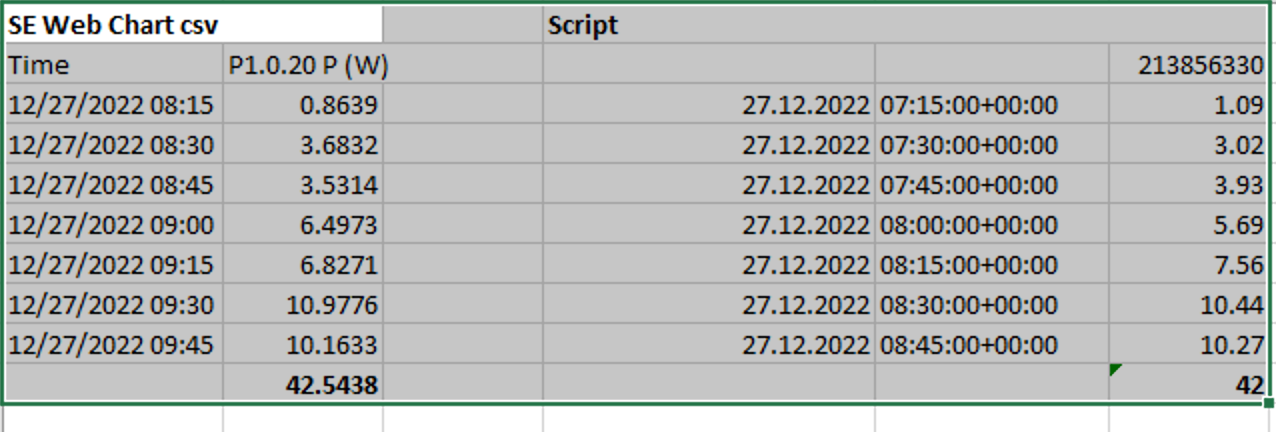
thank you for the advice. Tested the script results (before Influx) against the Solar Edge Web Portal (CSV export). It's quite similar, but not exactly the same. I assume it's some different roundings... See below:
I'm really not familiar with python, could you give me a hint where at the script you choose the data to be extracted and could the other data (Energy, Current, Voltage Panel, Voltage Optimizer) as well be extracted?
Merry christmay