Last active
June 2, 2020 11:40
-
-
Save hddananjaya/5b9d567d00799e69552c53be82ced866 to your computer and use it in GitHub Desktop.
Postgres CITEXT vs TEXT performance
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
But this is what happened when selecting data from the course.
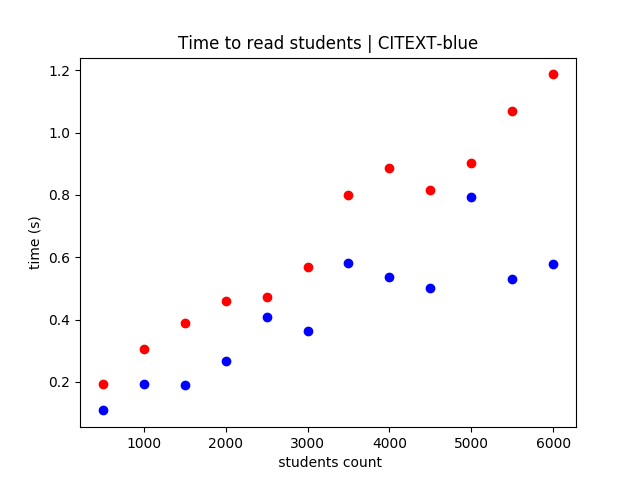
Code that I used for automating requests for selecting.
Code for the plotting