Obligatory disclaimer, this is all opinion and cannot possibly generalize to all problems, workflows, environments, etc. This is meant primarily as a launching point for an open-ended discussion on best practices in debugging code. This is a sketch of what may be a brief book that covers each point below with anecdotes, examples, and techniques.
The ultimate goal of a debugging session is to rule out possibilities until only one remains. All applications of best practices, techniques, and tools should be pointed at this purpose (contextualize all points below against this statement). Debugging is first and foremost a critical thinking problem, and presence of mind is your most critical asset. Establish a mental model but do not be afraid to invalidate it as the investigation unfolds.
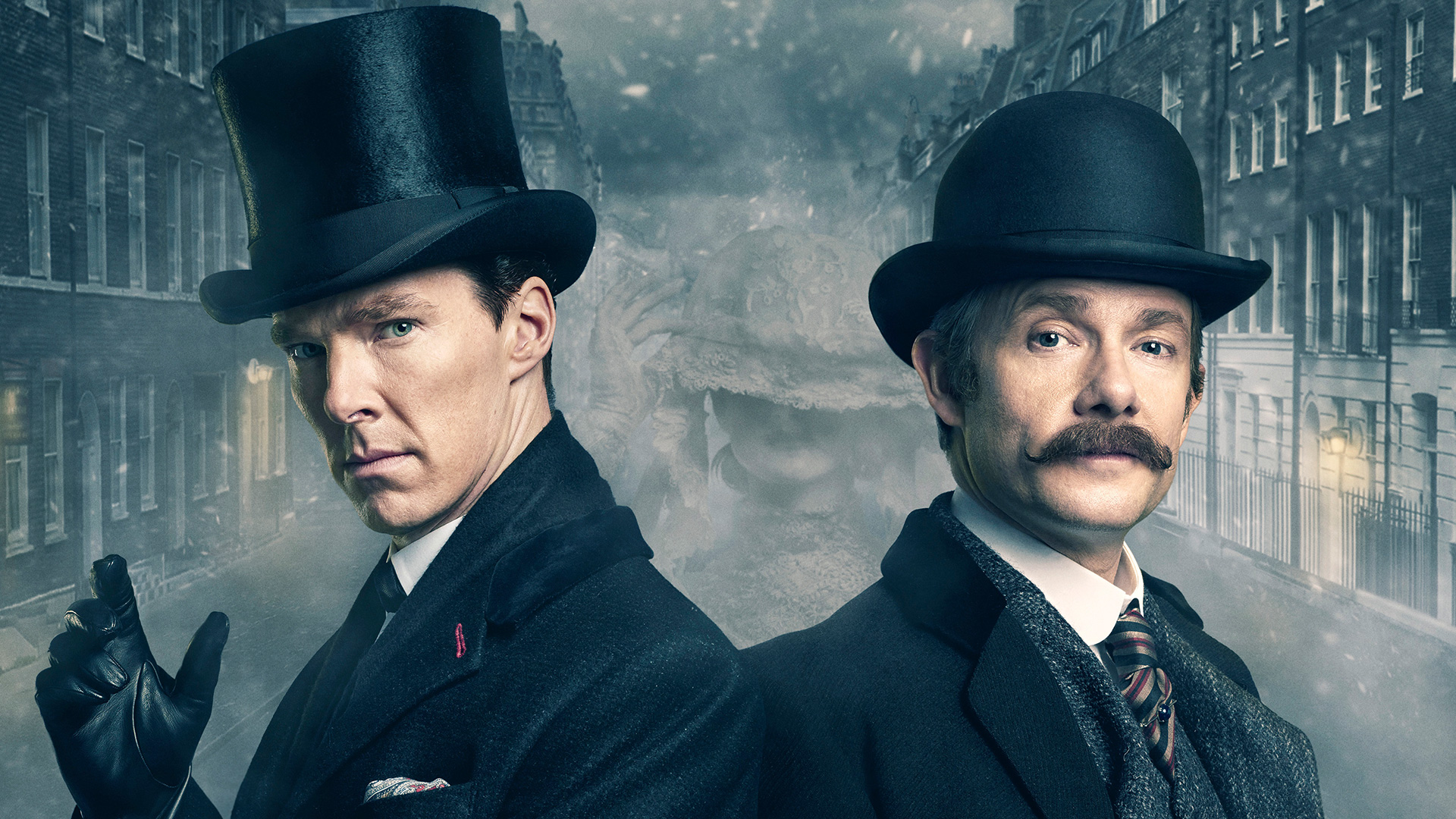