-
-
Save lagerone/1568ea6fbb98fd90a3495f9e51e26c8c to your computer and use it in GitHub Desktop.
#!/usr/bin/python3 | |
import logging | |
import os | |
import subprocess | |
import sys | |
from typing import Literal | |
logging.basicConfig(level=logging.DEBUG) | |
FILE_PATH = os.path.join( | |
os.path.dirname(os.path.realpath(__file__)), ".screen-brightness" | |
) | |
def read_current_level() -> float: | |
if not os.path.isfile(FILE_PATH): | |
return 1 | |
with open( | |
file=FILE_PATH, | |
mode="r", | |
encoding="utf-8", | |
) as file: | |
current_level = file.readline().strip() | |
return float(current_level) | |
def save_level(level: float) -> None: | |
with open( | |
file=FILE_PATH, | |
mode="w", | |
encoding="utf-8", | |
) as file: | |
file.write(str(level)) | |
def adjust_level(method: Literal["up", "down"]) -> None: | |
adjuster = 0.05 if method == "up" else -0.05 | |
current_level = read_current_level() | |
adjusted_level = current_level + adjuster | |
if adjusted_level > 1: | |
adjusted_level = 1 | |
if adjusted_level < 0.2: | |
adjusted_level = 0.2 | |
logging.debug(f"Setting screen brightness to {adjusted_level}.") | |
subprocess.run(["xrandr", "--output", "eDP-1", "--brightness", str(adjusted_level)]) | |
save_level(level=adjusted_level) | |
if __name__ == "__main__": | |
METHOD = sys.argv[1] if len(sys.argv) > 1 else "up" | |
adjust_level(method=METHOD) |
Very good script, from the terminal works fine, nonetheless when I call the script from the Keyboard Shortcut nothing happens, any idea what I might be doing wrong, I have tried different keyboard shortcuts combinations, thank you
it is working now, it was a permissions problem and I was using Wayland, I had to switch to X11, Wayland doesn't support the xrandr command, all good now, thank you
it is working now, it was a permissions problem and I was using Wayland, I had to switch to X11, Wayland doesn't support the xrandr command, all good now, thank you
Thanks for posting the solution to your problem 👍
In one of the latest 5.11 updates fixed this issue, because now I can adjust the OLED brightness with the system keyboard keys
I have a Lenovo X1 Yoga 1st Gen with OLED Screen, Ubuntu 21.10 with Kernel 5.13 installed and changing the screen brightness still doesn't work. Could anybody please help me? I have no idea what to do.
This is awesome. Very simple, much wow. Thanks for the work, man!
FYI, I tried to use the brightness keys on my laptop to be the shortcut to the script (Galaxy Book2 360 with Pop!_OS) and it didn't work. Apparently the brightness keys do something, so they don't call the script. Ended up using CTRL+SHIFT+F2|F3
which match where the brightness keys are.
Is there a reason it stops at 0.2
? Is it not safe or something? Since my display is OLED, depending on the lighting values below 0.2
are acceptable, but now I'm wondering if I might screwing up my display or something.
I made a small change to support basically turning off the display and have smaller intervals below 0.2
. Here's the patch if anyone is interested:
diff --git a/screen-brightness b/screen-brightness
index 84c8eef..7d4bd11 100755
--- a/screen-brightness
+++ b/screen-brightness
@@ -35,13 +35,13 @@ def save_level(level: float) -> None:
def adjust_level(method: Literal["up", "down"]) -> None:
- adjuster = 0.05 if method == "up" else -0.05
current_level = read_current_level()
- adjusted_level = current_level + adjuster
+ adjuster = 0.02 if current_level <= 0.2 else 0.05
+ adjusted_level = current_level + adjuster if method == "up" else current_level - adjuster
if adjusted_level > 1:
adjusted_level = 1
- if adjusted_level < 0.2:
- adjusted_level = 0.2
+ if adjusted_level < 0.0:
+ adjusted_level = 0.0
logging.debug(f"Setting screen brightness to {adjusted_level}.")
subprocess.run(["xrandr", "--output", "eDP-1", "--brightness", str(adjusted_level)])
save_level(level=adjusted_level)
Is there a reason it stops at
0.2
? Is it not safe or something? Since my display is OLED, depending on the lighting values below0.2
are acceptable, but now I'm wondering if I might screwing up my display or something.
No particular reason, it was a limit I added which suited my needs. It shouldn't break anything allowing it to go to 0.0
.
I use this script on Ubuntu 20.04. My laptop screen name was
eDP-1
in xrandr.Usage:
# increase brightness ./screen-brightness up
# lower brightness ./screen-brightness down
You can add custom keyboard shortcuts to invoke the script:
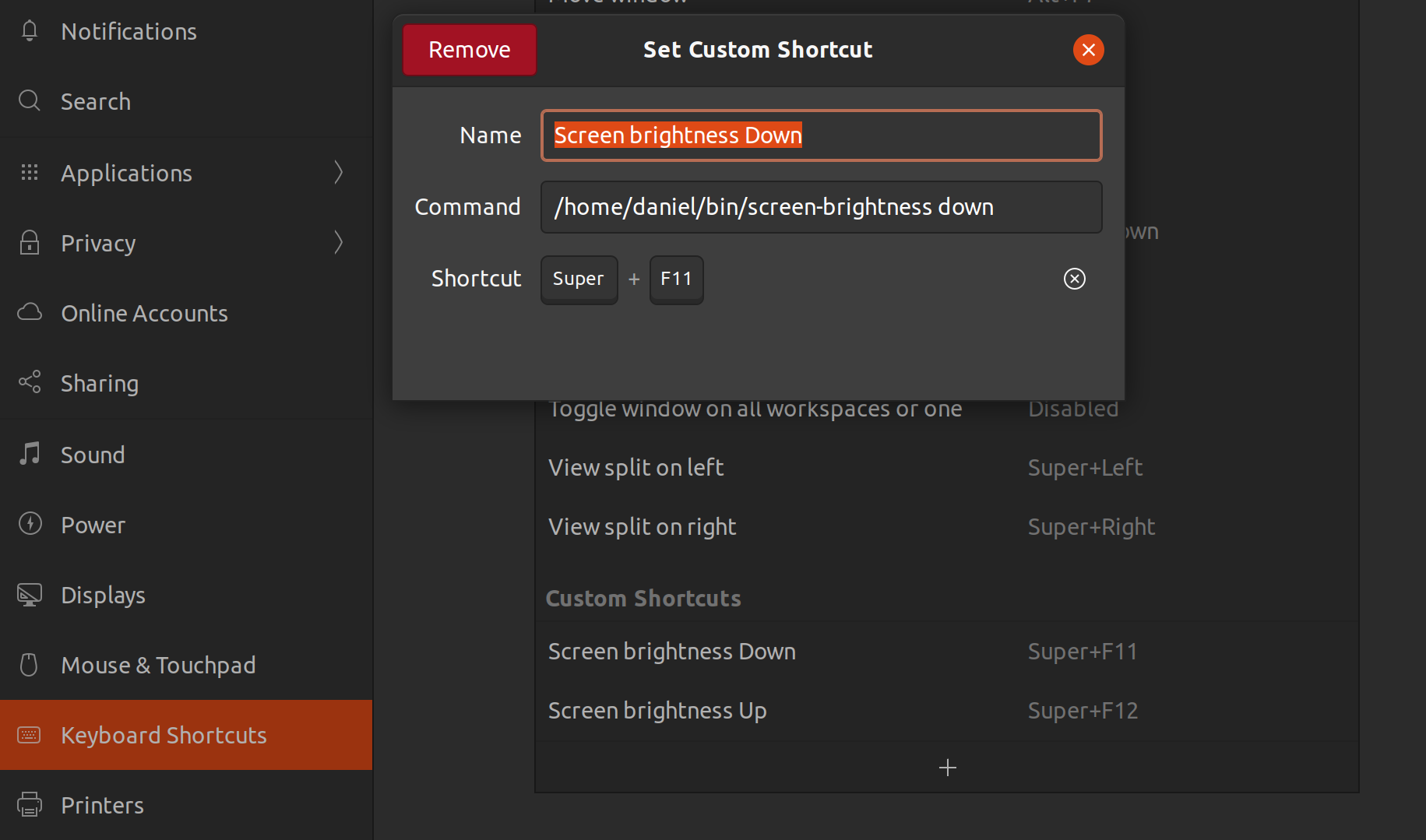