Last active
December 7, 2022 03:55
-
-
Save mikesparr/3a5166c290e3c4139621234b7cacb26c to your computer and use it in GitHub Desktop.
Experiment creating disk snapshots and deleting all but one to verify data restored for Google Cloud Platform (GCP) Compute Engine
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/env bash | |
##################################################################### | |
# REFERENCES | |
# - https://cloud.google.com/compute/docs/instances/create-start-instance | |
# - https://cloud.google.com/compute/docs/disks/create-snapshots | |
# - https://cloud.google.com/compute/docs/disks/restore-snapshot | |
##################################################################### | |
export PROJECT_ID=$(gcloud config get-value project) | |
export PROJECT_USER=$(gcloud config get-value core/account) # set current user | |
export PROJECT_NUMBER=$(gcloud projects describe $PROJECT_ID --format="value(projectNumber)") | |
export IDNS=${PROJECT_ID}.svc.id.goog # workflow identity domain | |
export GCP_REGION="us-west4" # CHANGEME (OPT) | |
export GCP_ZONE="us-west4-a" # CHANGEME (OPT) | |
export NETWORK_NAME="default" | |
# enable apis | |
gcloud services enable compute.googleapis.com \ | |
storage.googleapis.com | |
# configure gcloud sdk | |
gcloud config set compute/region $GCP_REGION | |
gcloud config set compute/zone $GCP_ZONE | |
############################################################# | |
# NETWORKING | |
############################################################# | |
# firewall allow ssh | |
gcloud compute firewall-rules create fw-allow-ssh \ | |
--network=$NETWORK_NAME \ | |
--action=allow \ | |
--direction=ingress \ | |
--target-tags=allow-ssh \ | |
--rules=tcp:22 | |
############################################################# | |
# COMPUTE | |
############################################################# | |
export INSTANCE_NAME="snapshot-test-1" # disk name should be same | |
# create compute instance to test from proxy-only network to ILB | |
gcloud compute instances create $INSTANCE_NAME \ | |
--machine-type e2-micro \ | |
--zone $GCP_ZONE \ | |
--network $NETWORK_NAME \ | |
--tags allow-ssh | |
############################################################# | |
# SNAPSHOTS | |
############################################################# | |
export IMAGE1_NAME="image1.jpg" | |
export IMAGE1_URL="https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/cute-cat-photos-1593441022.jpg" | |
export IMAGE2_NAME="image2.jpg" | |
export IMAGE2_URL="https://media.istockphoto.com/id/1267021092/photo/funny-winking-kitten.jpg" | |
export IMAGE3_NAME="image3.jpg" | |
export IMAGE3_URL="https://thumbs.dreamstime.com/b/portrait-funny-cat-fly-his-nose-portrait-funny-cat-fly-his-nose-isolated-white-background-125606127.jpg" | |
export SNAPSHOT1_NAME="snapshot1" | |
export SNAPSHOT2_NAME="snapshot2" | |
export SNAPSHOT3_NAME="snapshot3" | |
export DIR_NAME="cat-photos" | |
# create an images folder in home directory (via IAP tunnel) | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- mkdir -p $DIR_NAME | |
# ------- 1st image ----------- | |
# download image1 from web using curl (via IAP tunnel) | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- curl -o $DIR_NAME/$IMAGE1_NAME $IMAGE1_URL | |
# verify image1 in directory | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- ls -hl $DIR_NAME | |
# create snapshot of disk with only image1 on disk | |
gcloud compute snapshots create $SNAPSHOT1_NAME \ | |
--source-disk $INSTANCE_NAME \ | |
--source-disk-zone $GCP_ZONE | |
# ------- 2nd image ----------- | |
# download image2 from web using curl (via IAP tunnel) | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- curl -o $DIR_NAME/$IMAGE2_NAME $IMAGE2_URL | |
# verify image2 in directory | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- ls -hl $DIR_NAME | |
# create snapshot of disk with 2 images on disk | |
gcloud compute snapshots create $SNAPSHOT2_NAME \ | |
--source-disk $INSTANCE_NAME \ | |
--source-disk-zone $GCP_ZONE | |
# ------- 3rd image ----------- | |
# download image3 from web using curl (via IAP tunnel) | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- curl -o $DIR_NAME/$IMAGE3_NAME $IMAGE3_URL | |
# verify image3 in directory | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- ls -hl $DIR_NAME | |
# create snapshot of disk with 3 images on disk | |
gcloud compute snapshots create $SNAPSHOT3_NAME \ | |
--source-disk $INSTANCE_NAME \ | |
--source-disk-zone $GCP_ZONE | |
############################################################# | |
# SNAPSHOTS TEST | |
############################################################# | |
export DISK_NAME="new-disk1" | |
# delete snapshots 1,2 | |
gcloud compute snapshots delete $SNAPSHOT1_NAME | |
gcloud compute snapshots delete $SNAPSHOT2_NAME | |
# verify only snapshot3 remains | |
gcloud compute snapshots list | |
# stop instance, detach and delete disk (simulate disaster) | |
gcloud compute instances stop $INSTANCE_NAME --zone $GCP_ZONE | |
gcloud compute instances detach-disk $INSTANCE_NAME \ | |
--disk $INSTANCE_NAME \ | |
--zone $GCP_ZONE | |
gcloud compute disks delete $INSTANCE_NAME | |
# create disk from only remaining snapshot3 | |
gcloud compute disks create $DISK_NAME \ | |
--size="10GB" \ | |
--source-snapshot=$SNAPSHOT3_NAME | |
# attach disk (boot) to instance and start it back up | |
gcloud compute instances attach-disk $INSTANCE_NAME \ | |
--disk $DISK_NAME \ | |
--boot \ | |
--zone $GCP_ZONE | |
gcloud compute instances start $INSTANCE_NAME --zone $GCP_ZONE | |
# verify which images are in test images folder | |
gcloud compute ssh $INSTANCE_NAME --zone $GCP_ZONE -- ls -hl $DIR_NAME | |
# remember to clean up or delete project to avoid unnecessary billing | |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Disk snapshot recovery experiment
Recently a customer asked whether disk snapshots were an effective means to backup their self-managed database in case of need for disaster recovery. They noticed the first snapshot was much larger than the smaller subsequent incremental snapshots, and asked whether deleting some snapshots still rebuilds the entire disk.
Experiment
Result (success)
Note that the 2nd image was 0 bytes in size so restored disk was exact same as deleted.
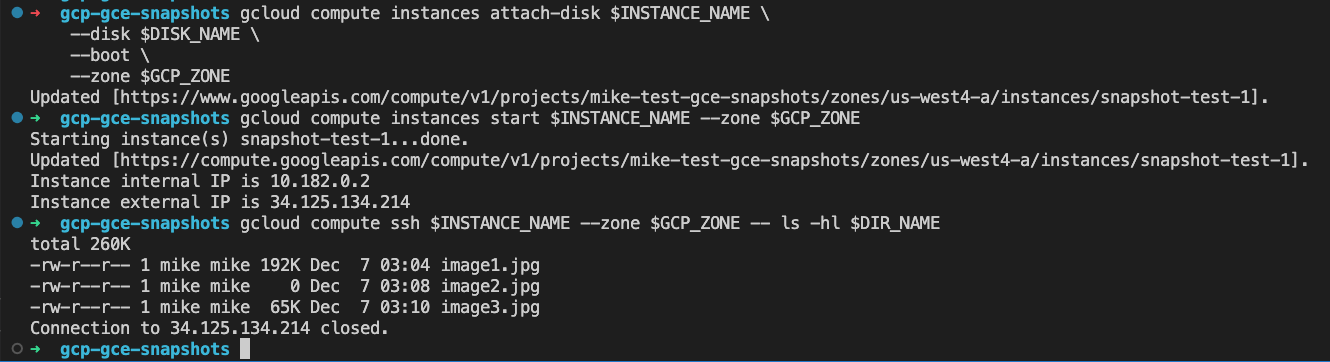