Last active
February 5, 2024 09:25
-
-
Save twilight-sparkle-irl/cb63762000e606e50690911cac1bcead to your computer and use it in GitHub Desktop.
webcrack: mess with webpacked (webpackJsonp) applications
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// webcrack, a script that allows you to break webpack.js's sandbox and randomization easily | |
// made by @yourcompanionAI | |
// licensed under the trust that you will credit me for my work visibly and other than that you can go have fun with this | |
// window.wc is the webcrack object | |
// wc.get gives you the module attached to the id you give it. | |
// literally just what webpack functions use. not much to it | |
// this is the basic part of all this, everything else is just to allow you to updateproof your code | |
// both find functions return modules in this format: | |
// {exports: [can be any type, whatever exports is set to in module], | |
// id: [number, id of module like those passed to wc.get], | |
// loaded: [boolean, if false, either try to load or just ignore this module]} | |
// wc.findFunc allows you to search modules by their constructor source (what you see in the script using webpackJsonp) | |
// pass a string to search for that string | |
// (for example, if you know the module you want has "api.example.com/echo" in it, you can pass that string to it to find it) | |
// pass a function and it will return modules where function(modulefunction) is truthy | |
// wc.findCache allows you to search modules as loaded in memory. | |
// pass a function, it will return modules where function(module) is truthy | |
// pass a string and it will return modules if their exports is an object and has the string as a key | |
// wc.modArr is the list of module constructors | |
// wc.modCache is the list of modules as loaded as an object | |
// wc.modCArr is modCache but as an array | |
webpackJsonp([0],[function(n,b,d){ | |
// d is the all holy require function. | |
mArr = d.m // appears to be an array of all module constructor functions | |
mCac = d.c // appears to be a object with a cache of modules as singletons | |
mCar = [] // no idea why modCache is a object since it only has sequential number keys (correct me if i'm wrong) | |
Object.keys(mCac).forEach(function(x){mCar[x] = mCac[x]}) | |
findFunc = function(s) { | |
results = [] | |
if (typeof s === "string") { | |
mArr.forEach(function(x,y){if(x.toString().indexOf(s) !== -1) { results.push(mCac[y]) }}) | |
} else if (typeof s === "function") { | |
modArray.forEach(function(x,y){if(s(x)) { results.push(d.c[y]) }}) | |
} else { | |
throw new TypeError('findFunc can only find via string and function, '+(typeof s)+' was passed') | |
} | |
return results | |
} | |
findCache = function(s) { | |
results = [] | |
if (typeof s === "function") { | |
mCar.forEach(function(x,y){if(s(x)) { results.push(x) }}) | |
} else if (typeof s === "string") { | |
mCar.forEach(function(x,y){ | |
// webpack is very indecisive about if stuff should go in default or not so we check both | |
if(typeof x.exports === "object") { | |
for (p in x.exports) { | |
if(p == s) { results.push(x) } | |
if(p == "default" && typeof x.exports.default === "object") { | |
for (p in x.exports.default) { | |
if(p == s) { results.push(x) } | |
} | |
} | |
} | |
} | |
}) | |
} else { | |
throw new TypeError('findCache can only find via function or string, '+(typeof s)+' was passed') | |
} | |
return results | |
} | |
window.wc = { get: d, modArr: mArr, modCache: mCac, modCArr: mCar, findFunc: findFunc, findCache: findCache } | |
}]) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// webcrack.min.js | |
// author: @yourcompanionAI | |
// licensed under the trust that you will credit me for my work visibly and other than that you can go have fun with this | |
webpackJsonp([0],[function(n,b,d){mArr=d.m,mCac=d.c,mCar=[],Object.keys(mCac).forEach(function(n){mCar[n]=mCac[n]}),findFunc=function(n){if(results=[],"string"==typeof n)mArr.forEach(function(r,t){-1!==r.toString().indexOf(n)&&results.push(mCac[t])});else{if("function"!=typeof n)throw new TypeError("findFunc can only find via string and function, "+typeof n+" was passed");modArray.forEach(function(r,e){n(r)&&results.push(t.c[e])})}return results},findCache=function(n){if(results=[],"function"==typeof n)mCar.forEach(function(r,t){n(r)&&results.push(r)});else{if("string"!=typeof n)throw new TypeError("findCache can only find via function or string, "+typeof n+" was passed");mCar.forEach(function(r,t){if("object"==typeof r.exports)for(p in r.exports)if(p==n&&results.push(r),"default"==p&&"object"==typeof r.exports["default"])for(p in r.exports["default"])p==n&&results.push(r)})}return results},window.wc={get:d,modArr:mArr,modCache:mCac,modCArr:mCar,findFunc:findFunc,findCache:findCache}}]); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// example for discord, tested on discord-canary | |
// load webcrack before this | |
c = (x => x[0].exports) // simply grabs the first result's exported format from a search | |
randomItem = (arr => arr[Math.floor(Math.random() * arr.length)]) // gets a random item from an array | |
config = c(wc.findCache("API_HOST")) // you can find modules with keys in exports | |
dispatcher = c(wc.findCache("dirtyDispatch")).default // you can find modules with keys in exports.defaults | |
gamelist = c(wc.findFunc("ZSNES")) // you can find modules with strings in them | |
dispatcher.dirtyDispatch({ // tell dispatcher... | |
type: config.ActionTypes.RUNNING_GAMES_CHANGE, // to say the running game's name has changed... | |
games: [{"gameName": randomItem(gamelist).name}] // to a random game that discord recognizes. | |
}) |
Licensing isn't very clear. Also, usage instructions might help.
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
it doesn't seems to be function but an array
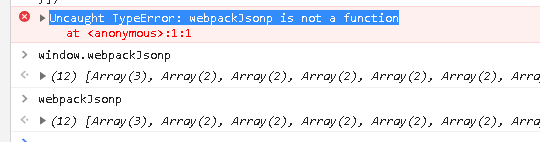