Last active
August 29, 2015 14:27
-
-
Save clamytoe/51343bfafc7eb2580acd to your computer and use it in GitHub Desktop.
HKUSTx: COMP107x Introduction to Mobile Application Development using Android
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Greet Friend (Part 1)\n", | |
"---\n", | |
"## Objectives\n", | |
"\n", | |
"At the end of this exercise, you will be able to:\n", | |
"\n", | |
"* Import a project into Android Studio\n", | |
"* Create a UI layout with various UI widgets (TextView, EditText and Button)\n", | |
"\n", | |
"## Introduction\n", | |
"\n", | |
"In this exercise, we implement a simple Android application. The app should ask the user to type in his/her friend's name and display a greeting message to the friend on the screen when the button is clicked. The fully functional app will function as shown in the next unit.\n", | |
"\n", | |
"Many times as you develop Android applications, you may require to import a pre-configured project into Android Studio. We learn the steps for accomplishing this first. Thereafter, we learn creating a user interface for an application using various UI widgets. While we illustrate with a few widgets, the general procedure is applicable to any UI widget that you might wish to include in a UI.\n", | |
"\n", | |
"## Textual Instructions\n", | |
"---\n", | |
"### Step1: Import the Barebones Greet Friend Project into Android Studio\n", | |
"\n", | |
"We provide you with a barebones Greet Friend app that is pre-configured with some settings to enable you to get started. The app will also carry out some automated tests to ensure that you have completed the exercise correctly. To import the project into Android Studio, follow the steps below. This procedure works for any project that you may wish to import into Android Studio.\n", | |
"\n", | |
"1. Download the [GreetFriend.zip](http://w02.hkvu.hk/edX/COMP107x/w1/GreetFriend.zip) file to the folder where you normally store your Android Studio projects.\n", | |
"2. Unzip the file. This will create a folder named **GreetFriend**. This folder holds all the files corresponding to your Android Studio project.\n", | |
"\n", | |
"3. Start Android Studio. When Android Studio is ready, click on the **Open an existing Android Studio project**.\n", | |
" \n", | |
" 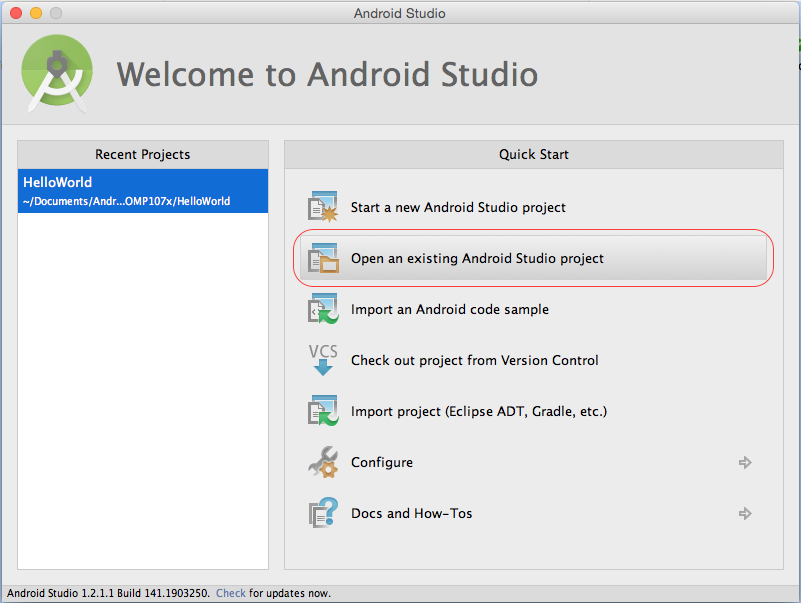<br/>\n", | |
" \n", | |
"4. When the file selection window opens, navigate to the folder where you unzipped the file and select the **GreetFriend** folder.\n", | |
" \n", | |
" 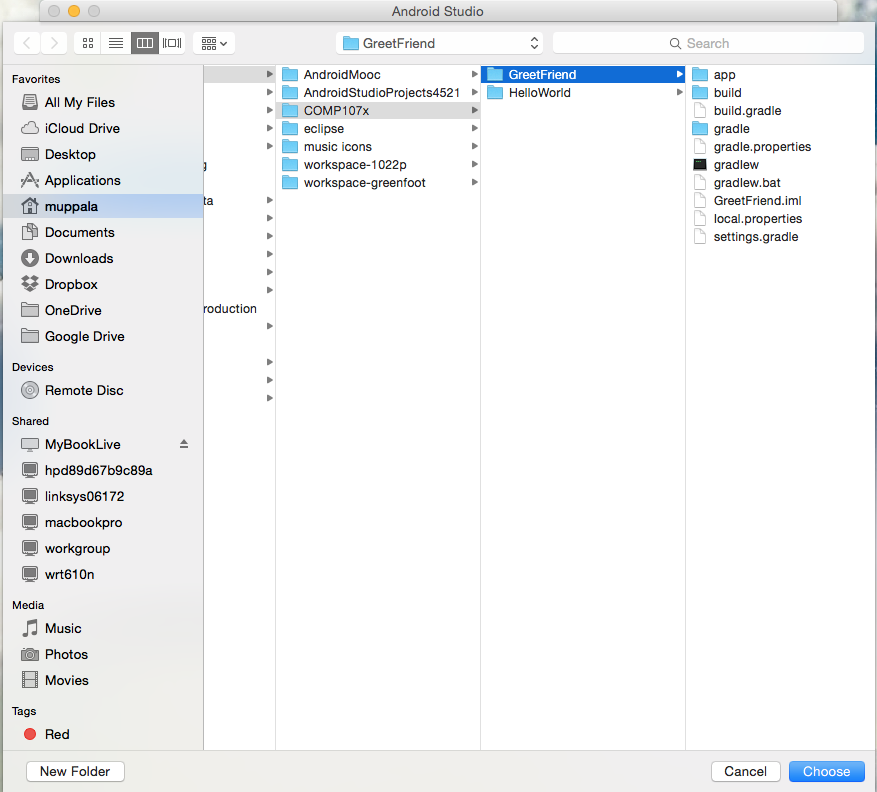<br/>\n", | |
" \n", | |
"5. Android Studio will import the project, do a Gradle build and open the project. If the project files are not shown, click on the project tab in the window.\n", | |
" \n", | |
" 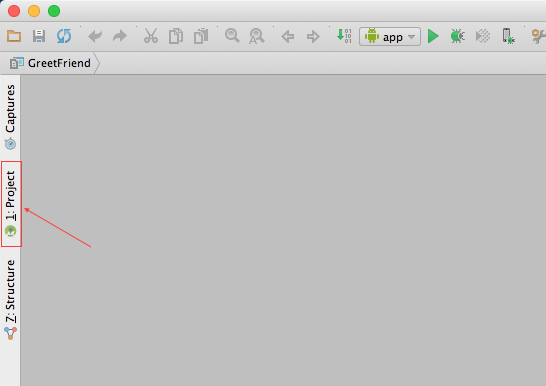<br/>\n", | |
" \n", | |
"6. Once the project files are open, then in the folder tree on the left, click to open **MainActivity.java** and **activity_main.xml** files.\n", | |
" \n", | |
" 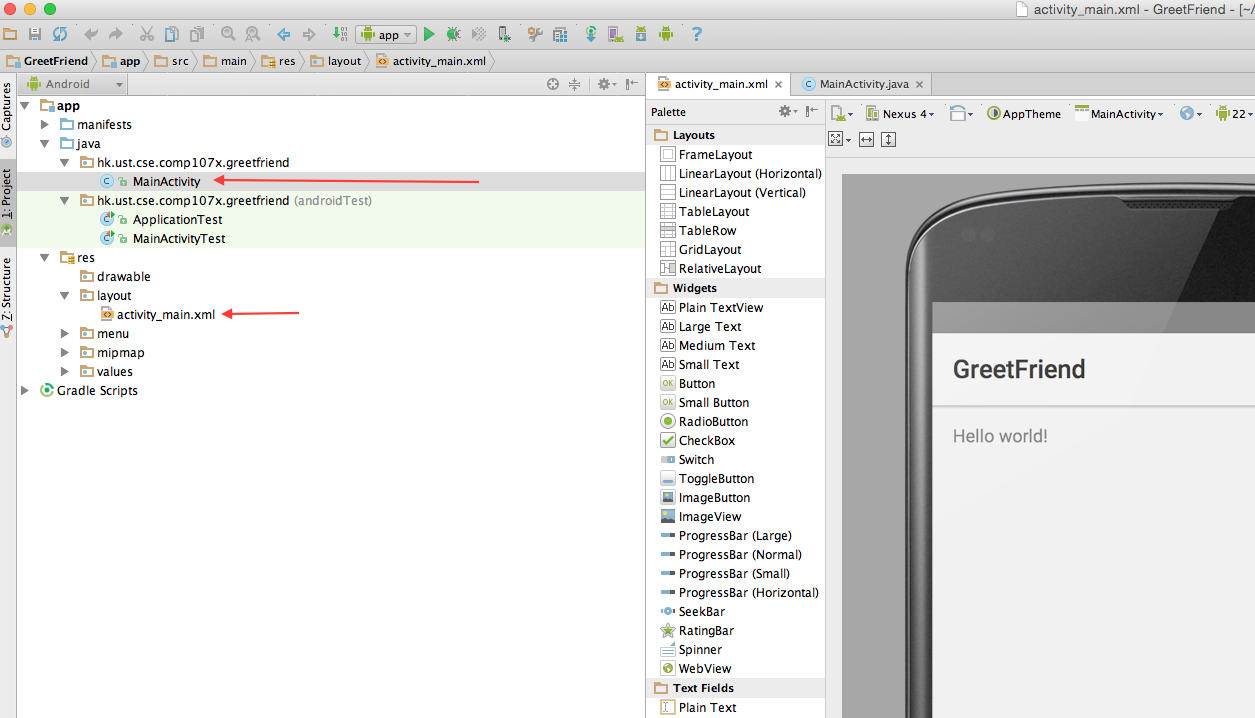<br/>\n", | |
"\n", | |
"---\n", | |
"### Step 2: Creating the Layout for the Greet Friend App User Interface\n", | |
"\n", | |
"Now that the GreetFriend project is imported, let us create the UI layout for the Greet Friend app. Note that in the following steps, we use italics to indicate what you need to type in, and use ***bold*** letters for field names. Also, Android specific terms and concepts will be highlighted in **bold**.\n", | |
"\n", | |
"1. On the design tab of the layout, click on the **Hello world!** text once to select it. Android uses a **TextView** UI widget to display a string of text on the UI. Double click on the string until a small window opens displaying ***text:*** and ***id:***. In the ***id*** field, type in textMessage. When referring to it in code, we use **R.id.textMessage**. The same widget when referred to in an XML file, will be referred to as **@+id/textMessage**.\n", | |
"\n", | |
" 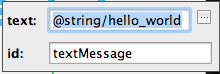<br/>\n", | |
"\n", | |
"2. Next, drag to position the TextView at the center of the screen as you feel appropriate. The layout properties of the TextView will automatically adjust as you make the changes.\n", | |
"\n", | |
"3. You can also make changes to the UI widget properties using the Properties window on the right side. Use the ***textSize*** property to set the text size of the TextView to *24sp*. (Note: sp refers to scale-independent pixel. We specify text sizes in sp units so that the text automatically adapts in size to the screen resolution.)\n", | |
" \n", | |
" 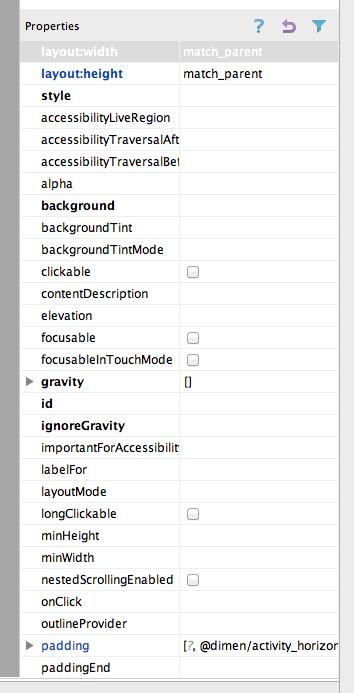<br/>\n", | |
"\n", | |
"4. The panel on the left side of the layout provides many UI widgets that can be dragged onto and positioned on the UI. Drag a Plain Text **Text Field** and position it below the TextView. The text field is referred to as **EditText** widget in Android. Set its ***id*** to be *editFriendName*. Using the Properties window, set its ***layout_width*** to be *match_parent*. Also set the ***hint*** field to be \"*Type in your friend's name*\".\n", | |
"\n", | |
"5. Below the EditText widget, drag in a **button** widget. Set its ***text*** to be greetings, and its id to be *greetButton*. At the end of these steps, your UI should be as shown below.\n", | |
" \n", | |
" 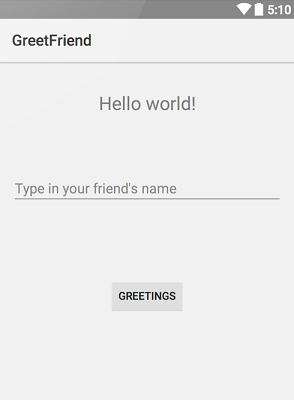<br/>\n", | |
"\n", | |
"6. At this stage, you can run the application on the emulator or a device. However, the button is not yet activated and so nothing will happen when you click on it." | |
] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Python 2", | |
"language": "python", | |
"name": "python2" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 2 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython2", | |
"version": "2.7.6" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 0 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment