Last active
August 29, 2015 14:27
-
-
Save clamytoe/51343bfafc7eb2580acd to your computer and use it in GitHub Desktop.
HKUSTx: COMP107x Introduction to Mobile Application Development using Android
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Exercise: Greet Friend (with Activity)\n", | |
"---\n", | |
"## Objectives\n", | |
"\n", | |
"In this exercise, you will learn how to add a new activity to the application, and how to start a new activity from the current activity. At the end of this exercise you will be able to:\n", | |
"\n", | |
"* Understand the steps to add new activities to an application\n", | |
"* Start a new activity from the current activity using an **Intent**\n", | |
"* Pass data from the current activity to the new activity by adding it to the **Intent** as a payload in the form of a key-value pair.\n", | |
"* Get access to the Intent that resulted in starting this new activity and retrieve the data from the Intent\n", | |
"\n", | |
"\n", | |
"## Introduction\n", | |
"\n", | |
"We will now modify the GreetFriend app that we developed earlier such that the user types his/her friend's name in the UI and when the greetings button is clicked, the app will switch to a new activity named **ShowMessage**. The UI of the ShowMessage activity will display the greeting message.\n", | |
"\n", | |
"## Textual Instructions\n", | |
"---\n", | |
"### Step 1: Adding a new Activity\n", | |
"\n", | |
"1. You can start with the version of the GreetFriend app that you completed at the end of the GreetFriend Exercise Part 2 in the previous section. Alternately, you can download the updated [GreetFriend.zip](http://w02.hkvu.hk/edX/COMP107x/w2/GreetFriend.zip) file, unzip it and import it into Android Studio.\n", | |
"\n", | |
"2. Open the **activity_main.xml** file. Then, delete the TextView with the ID textMessage from the UI layout and save the file.\n", | |
"\n", | |
"3. Go to the left side under the project tree and right click on the location specified in the figure below and add a new **Blank Activity**.\n", | |
" \n", | |
" 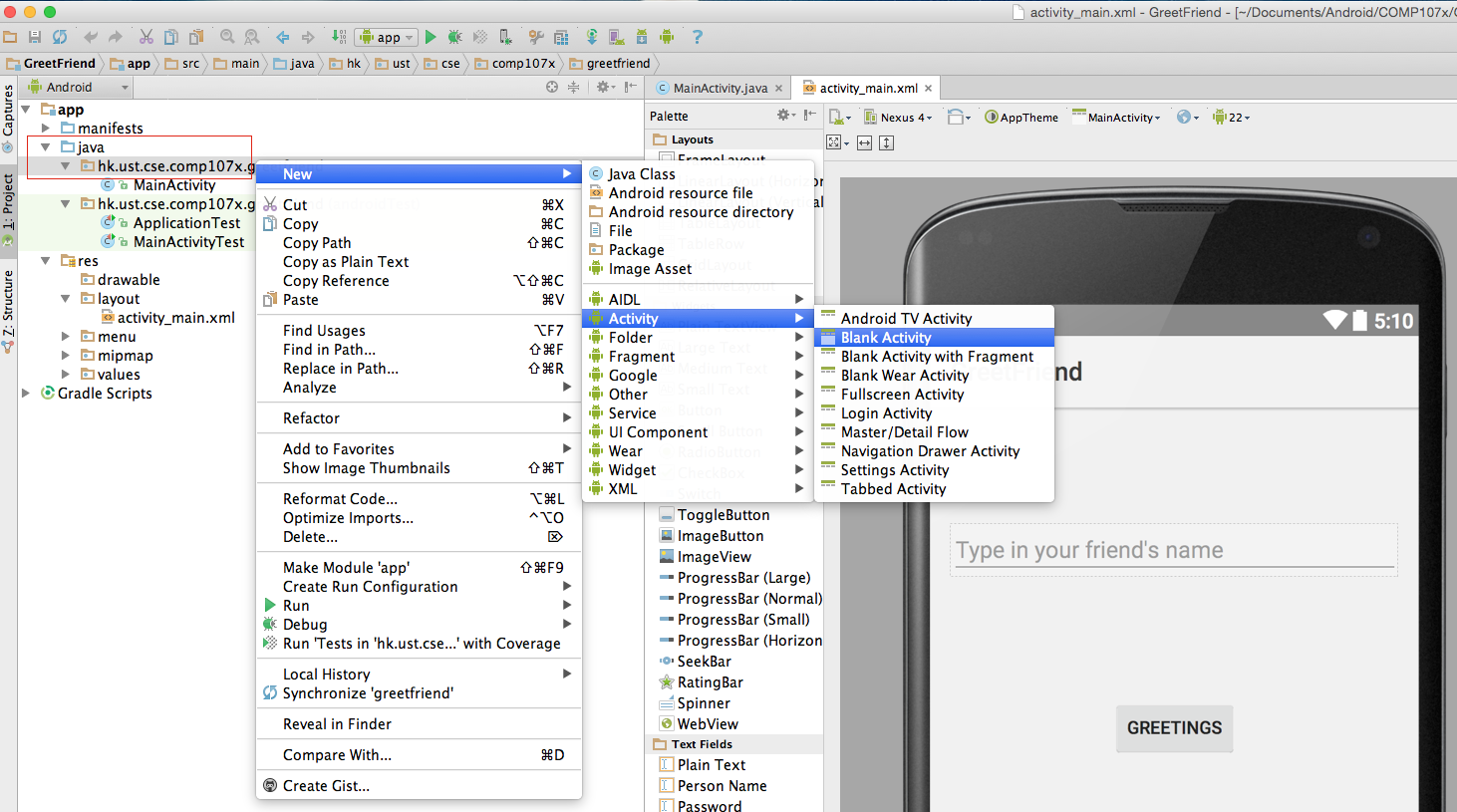<br/>\n", | |
"\n", | |
"4. When the new activity configuration window opens, type in the name of the new activity as *ShowMessage*.\n", | |
"\n", | |
"5. Open **activity_show_message.xml** file. Then, position the \"Hello world!\" string to the center of the screen and change its ***text size*** to *24sp*.\n", | |
"\n", | |
"6. Double click on the \"Hello world!\" string and key in *textMessage* as the ***ID***. We will be using this TextView to display the greeting message.\n", | |
"\n", | |
"---\n", | |
"### Step 2: Modifying the Code\n", | |
"\n", | |
"1. Open **MainActivity.java** file and update the onClick() method code as shown below. You can read the comments in the code to understand the reasons for the modification, or watch the video instructions in the previous unit. Then save the changes to the file.\n", | |
"\n", | |
" @Override\n", | |
" public void onClick(View view) {\n", | |
"\n", | |
" TextView textMessage = (TextView) findViewById(R.id.textMessage);\n", | |
"\n", | |
" EditText editFriendName = (EditText) findViewById(R.id.editFriendName);\n", | |
"\n", | |
" String friendName = editFriendName.getText().toString();\n", | |
"\n", | |
" switch (view.getId()) {\n", | |
"\n", | |
" case R.id.greetButton:\n", | |
"\n", | |
" // create a new intent. The first parameter is the Context which is the current Activity.\n", | |
" // Hence we use \"this\". The second parameter is the Activity class that we wish to start.\n", | |
" // Hence it is specified as ShowMessage.class\n", | |
" Intent in = new Intent(this,ShowMessage.class);\n", | |
"\n", | |
" // Add the message as a payload to the Intent. We add data to be carried by the intern using\n", | |
" // the putExtra() methods. The data is specified as a key-value pair. The first parameter is\n", | |
" // the key, specified as a string, and the second parameter is the value.\n", | |
" in.putExtra(\"message\", getString(R.string.greetstring) + friendName + \"!\");\n", | |
"\n", | |
" // We start the new activity by calling this method to inform the Android framework to start\n", | |
" // the new activity. The parameter is the Intent we just created earlier\n", | |
" startActivity(in);\n", | |
"\n", | |
" break;\n", | |
"\n", | |
" default:\n", | |
" break;\n", | |
" }\n", | |
" }\n", | |
" \n", | |
"2. Open **ShowMessage.java** file and update the onCreate() method code as shown below. You can read the comments in the code to understand the reasons for the modification, or watch the video instructions in the previous unit. Then save the changes to the file.\n", | |
"\n", | |
" @Override\n", | |
" protected void onCreate(Bundle savedInstanceState) {\n", | |
" super.onCreate(savedInstanceState);\n", | |
" setContentView(R.layout.activity_show_message);\n", | |
"\n", | |
" // We first get a reference to the Intent that resulted in this activity\n", | |
" // being started by the Android framework.\n", | |
" Intent in = getIntent();\n", | |
"\n", | |
" // From the Intent we retrieve the message that was sent from MainActivity\n", | |
" // Note the use of the same key, \"message\", to retrieve the message\n", | |
" String message = in.getStringExtra(\"message\");\n", | |
"\n", | |
" // Get the reference to the TextView on the ShowMessage UI\n", | |
" TextView textMessage = (TextView) findViewById(R.id.textMessage);\n", | |
"\n", | |
" // set the text of the TextView to display the incoming greeting message\n", | |
" textMessage.setText(message);\n", | |
" }\n", | |
"\n", | |
"3. Now, run the application and see how the app starts with the GreetFriend activity. See the updated UI. When you type in your friend's name and click the Greetings button, it switches to the ShowMessage activity and displays the greetings message on the screen." | |
] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Python 2", | |
"language": "python", | |
"name": "python2" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 2 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython2", | |
"version": "2.7.6" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 0 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment