Last active
August 29, 2015 14:27
-
-
Save clamytoe/51343bfafc7eb2580acd to your computer and use it in GitHub Desktop.
HKUSTx: COMP107x Introduction to Mobile Application Development using Android
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
{ | |
"cells": [ | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"# Assignment: Time Greet Friend\n", | |
"---\n", | |
"In this assignment you will modify the GreetFriend App to show the greeting based on the time of the day as listed below:\n", | |
"\n", | |
"* At or after 6 am and before 12 noon it should show \"Good Morning <friend's name>!\"\n", | |
"* At or after 12 noon and before 5 pm it should show \"Good Afternoon <friend's name>!\"\n", | |
"* At or after 5 pm and before 9 pm it should show \"Good Evening <friend's name>!\"\n", | |
"* At or after 9 pm and before 6 am it should show \"Good Night <friend's name>!\"\n", | |
"\n", | |
"Note: Your output message should be exactly the same as the greeting message above with exactly the same number of white spaces in exactly the same positions.\n", | |
"\n", | |
"To get you started, please download the following pre-configured Android project [TimeGreetFriend.zip](http://w02.hkvu.hk/edX/COMP107x/w1/TimeGreetFriend.zip) and import it into Android Studio. This project is configured with the complete GreetFriend application at the end of the Greet Friend (Part 2) exercise. You can use this as the starting point for the assignment.\n", | |
"\n", | |
"---\n", | |
"## Hint\n", | |
"\n", | |
"The following code snippet will give you the hour of the day. You can use it to implement your solution." | |
] | |
}, | |
{ | |
"cell_type": "raw", | |
"metadata": { | |
"collapsed": true | |
}, | |
"source": [ | |
"\n", | |
" Date date = new Date();\n", | |
" Calendar cal = Calendar.getInstance();\n", | |
" cal.setTime(date);\n", | |
" int hour = cal.get(Calendar.HOUR_OF_DAY);\n" | |
] | |
}, | |
{ | |
"cell_type": "markdown", | |
"metadata": {}, | |
"source": [ | |
"## Textual Instructions\n", | |
"---\n", | |
"### Testing Procedure\n", | |
"\n", | |
"To show that you have done the implementation of your app, you need to follow the steps below and capture a screenshot - which is to be submitted through the assignment submission part in the next unit.\n", | |
"\n", | |
"Note: You may click on this [link](https://courses.edx.org/courses/course-v1:HKUSTx+COMP107x+2016_T1/jump_to_id/f730b04c646e47208799f7996f34658f) to view the video instructions again on how to carry out the automated test for your assignment.\n", | |
"\n", | |
"1. After you import the pre-configured project into Android Studio and implement your solution to the assignment, please proceed to the next step.\n", | |
" \n", | |
" 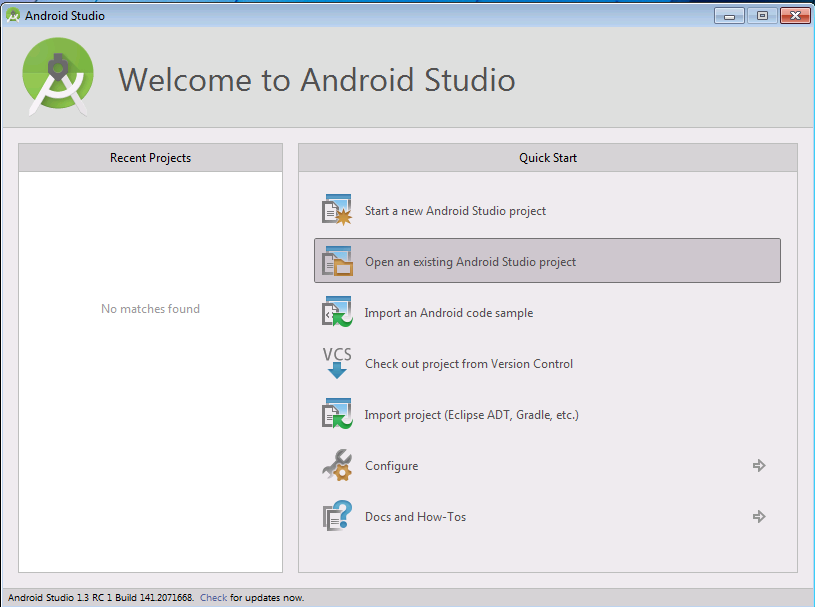<br/>\n", | |
"\n", | |
"2. Conduct an automated test to make sure your code has been implemented correctly. To do that, you need to add a new Run Configuration to the project by clicking on “**app**” and then selecting “**Edit Configurations…**” as shown in the image below.\n", | |
" \n", | |
" 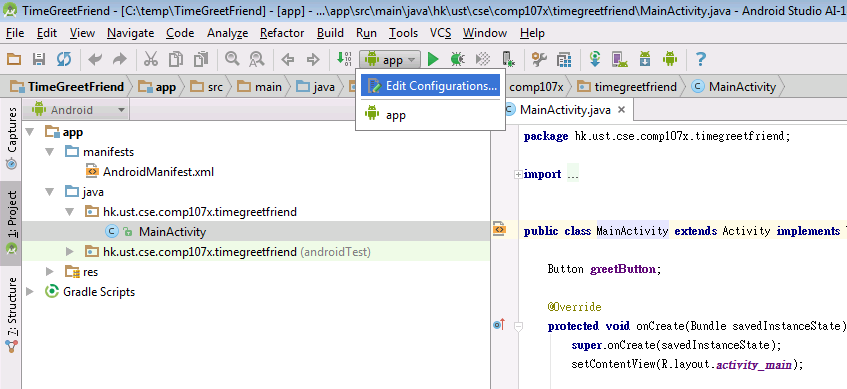<br/>\n", | |
"\n", | |
"3. When the “**Run/Debug Configuration**” window starts, click on the plus sign (“+”) at the upper left corner, as shown below, to add a new configuration. Select “**Android Tests**” from the menu.\n", | |
" \n", | |
" 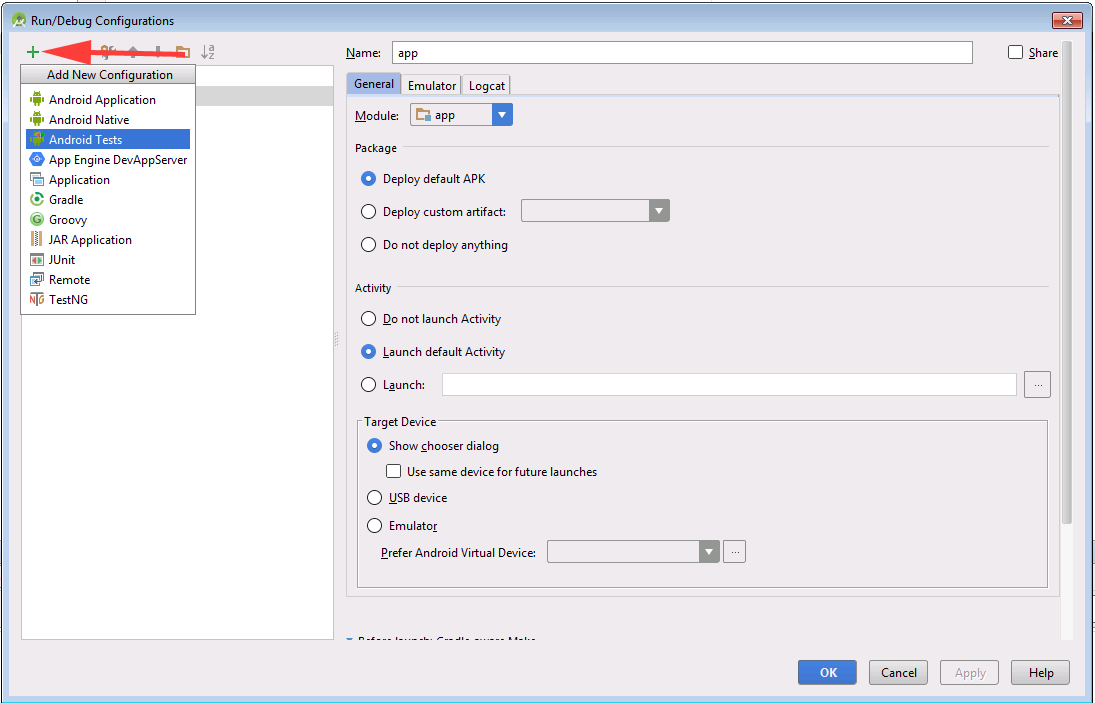<br/>\n", | |
"\n", | |
"4. When the configuration window opens, type the Name as “test” and select the Module as “app”, as shown below.\n", | |
" \n", | |
" 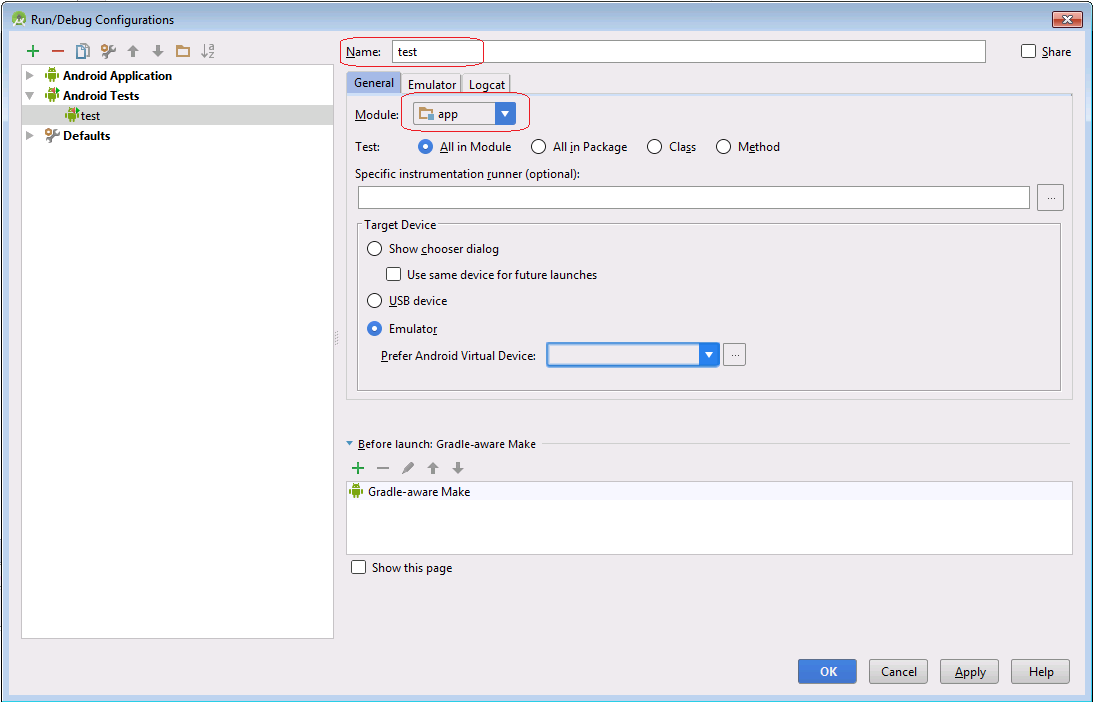<br/>\n", | |
"\n", | |
"5. Click on the **Run ‘test’** icon to run the automated test. If the Android Virtual device’s screen has been locked, make sure you unlock it before running the test. Otherwise, the test could fail with complaints (e.g. unable to perform a click or can not get view focus for a period of time, etc.).\n", | |
" \n", | |
" 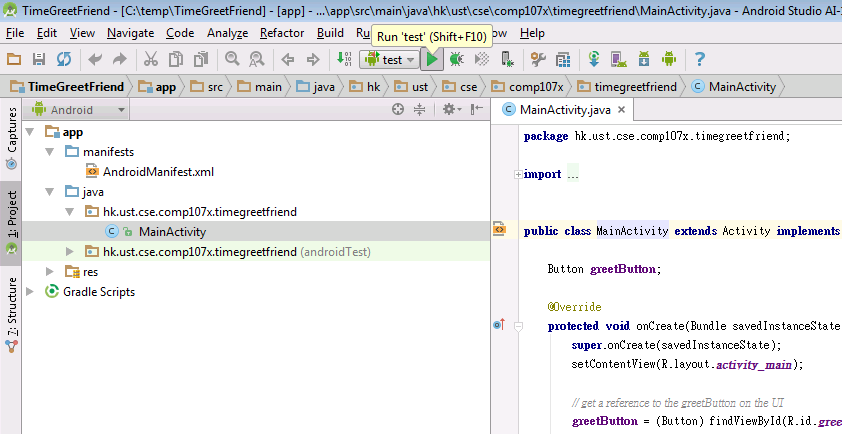<br/>\n", | |
"\n", | |
"6. Upon completion, if all the tests succeeded, then you will see a screen with a *green bar* as shown below.\n", | |
" \n", | |
" 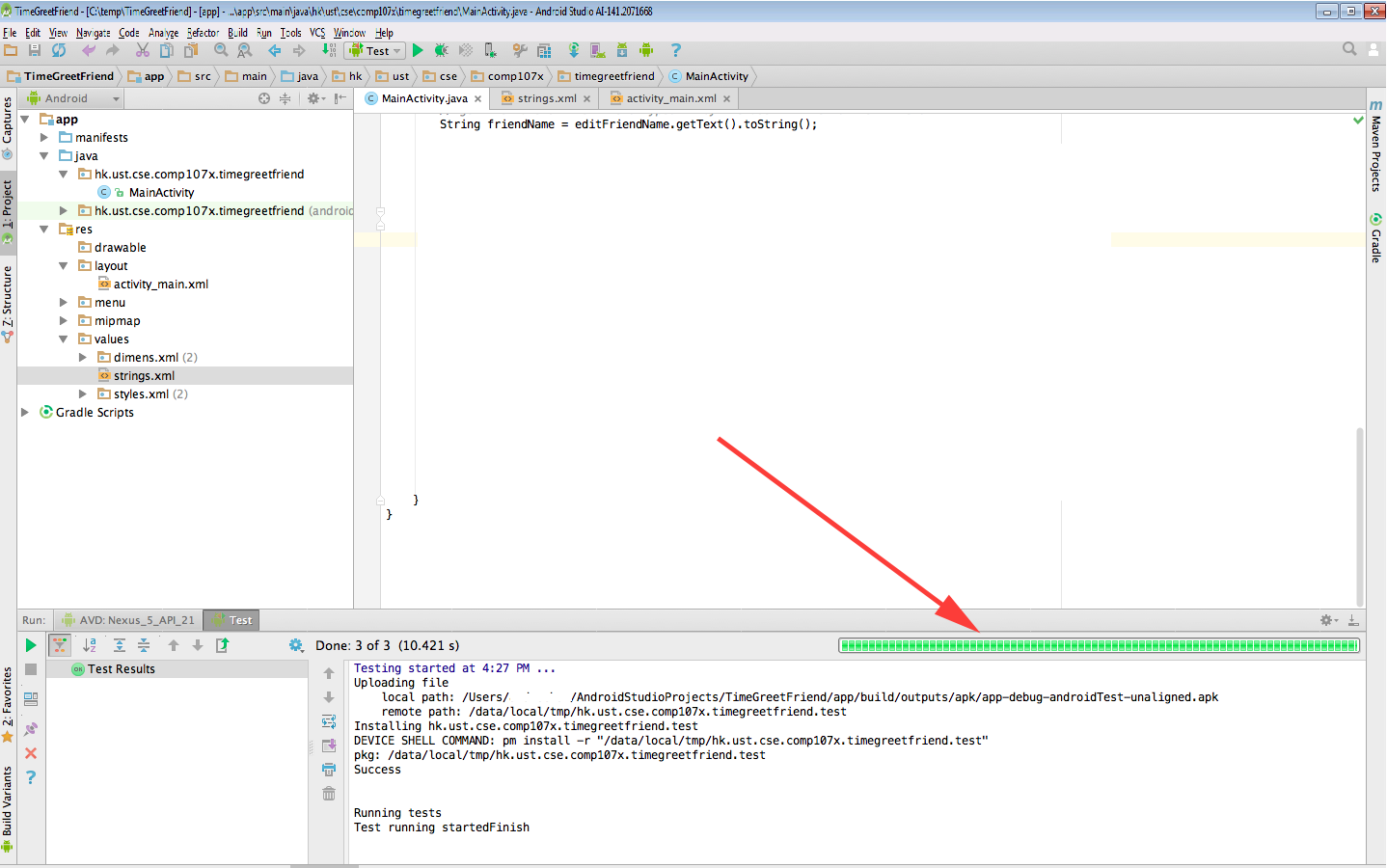<br/>\n", | |
"\n", | |
"7. If some of the tests failed, then you will see a screen with a *red* bar as shown below.\n", | |
" \n", | |
" 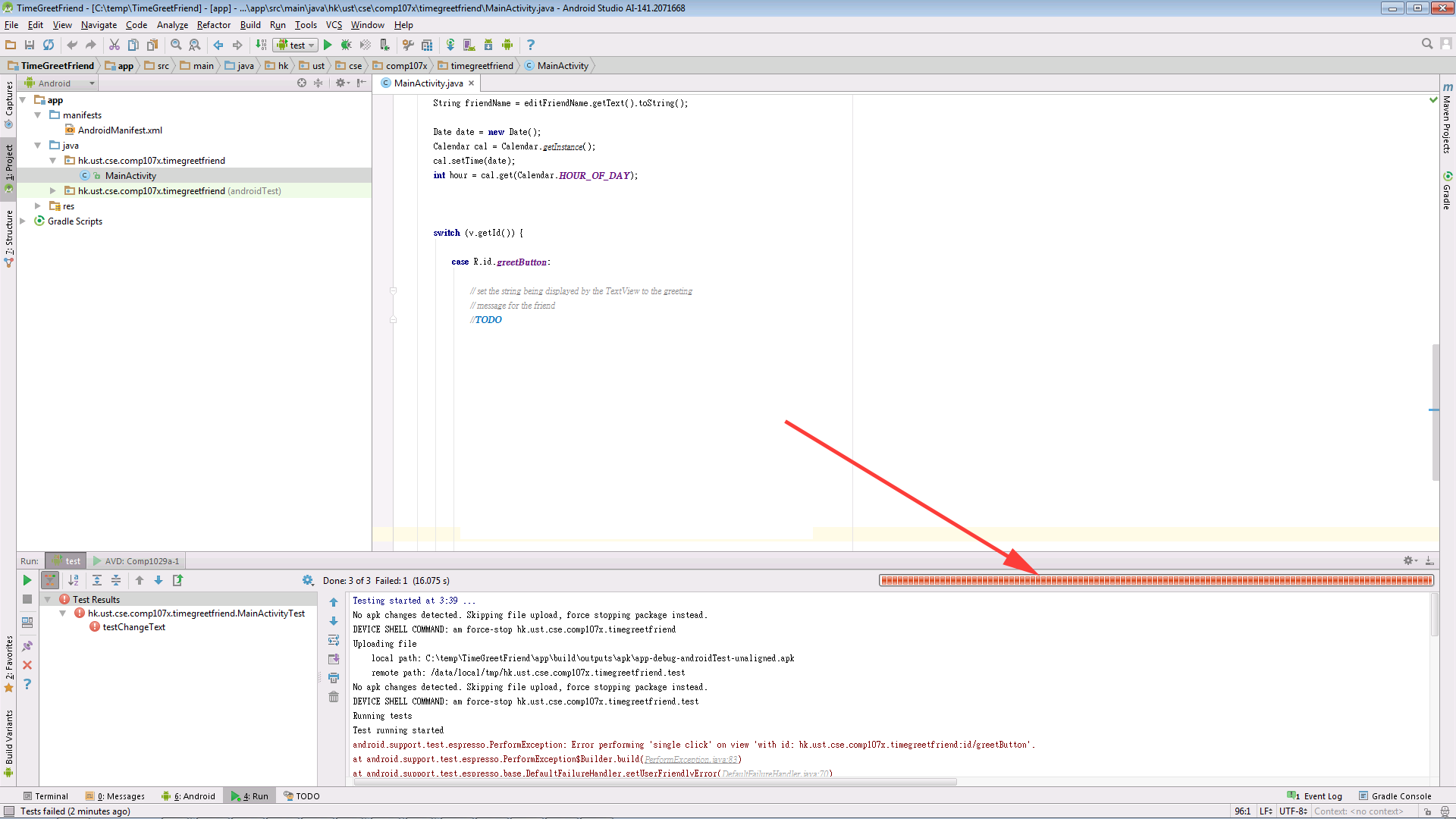<br/>\n", | |
"\n", | |
"8. Capture the result of the test in a screenshot. Save the screenshot as **jpg/png** format, this will be the image that you will need to submit in the assignment response. \n", | |
" \n", | |
" 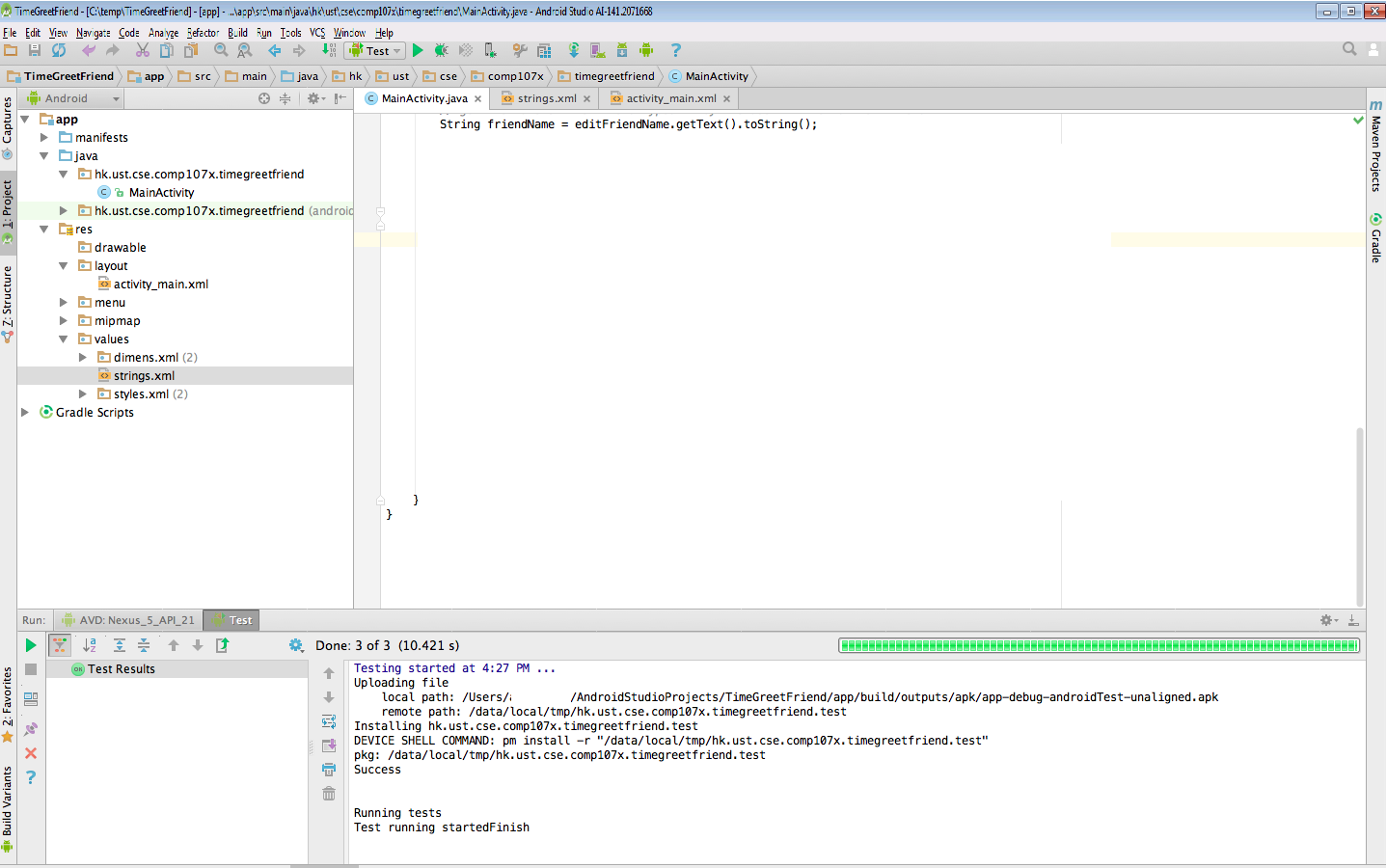<br/>\n" | |
] | |
} | |
], | |
"metadata": { | |
"kernelspec": { | |
"display_name": "Python 2", | |
"language": "python", | |
"name": "python2" | |
}, | |
"language_info": { | |
"codemirror_mode": { | |
"name": "ipython", | |
"version": 2 | |
}, | |
"file_extension": ".py", | |
"mimetype": "text/x-python", | |
"name": "python", | |
"nbconvert_exporter": "python", | |
"pygments_lexer": "ipython2", | |
"version": "2.7.6" | |
} | |
}, | |
"nbformat": 4, | |
"nbformat_minor": 0 | |
} |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment