Revision 5 http://webapps.stackexchange.com/revisions/89566/5
#Short answer
The link points to a Q&A that has two answers but non-of them looks to me to be appropiate. I.E. the code of one of answers is incomplete and both of them send emails, so the adoption is too complex to be used as a starting point.
Below is a script that is easier to adopt.
#Instructions
-
Create a test form and note the form ID (between ../d/
and /edit
in the url).
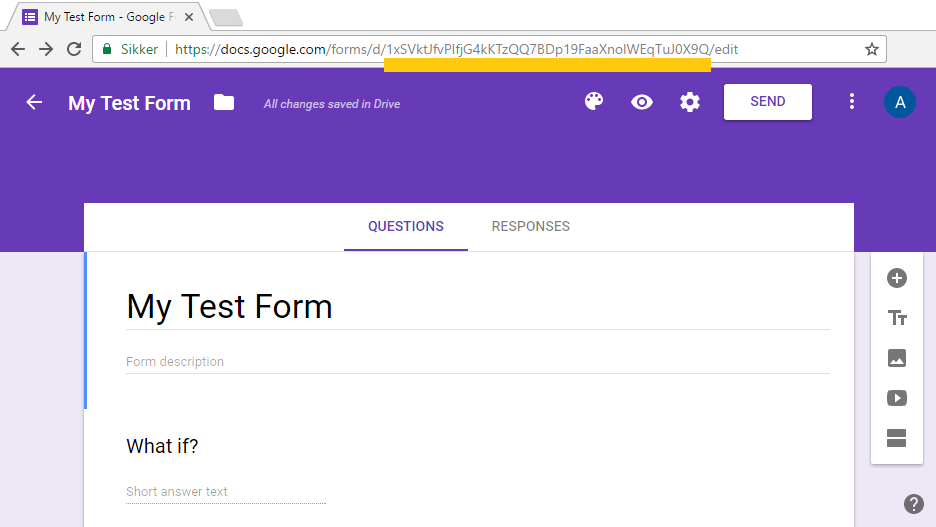
-
Set the form to send responses to a new spreadsheet.
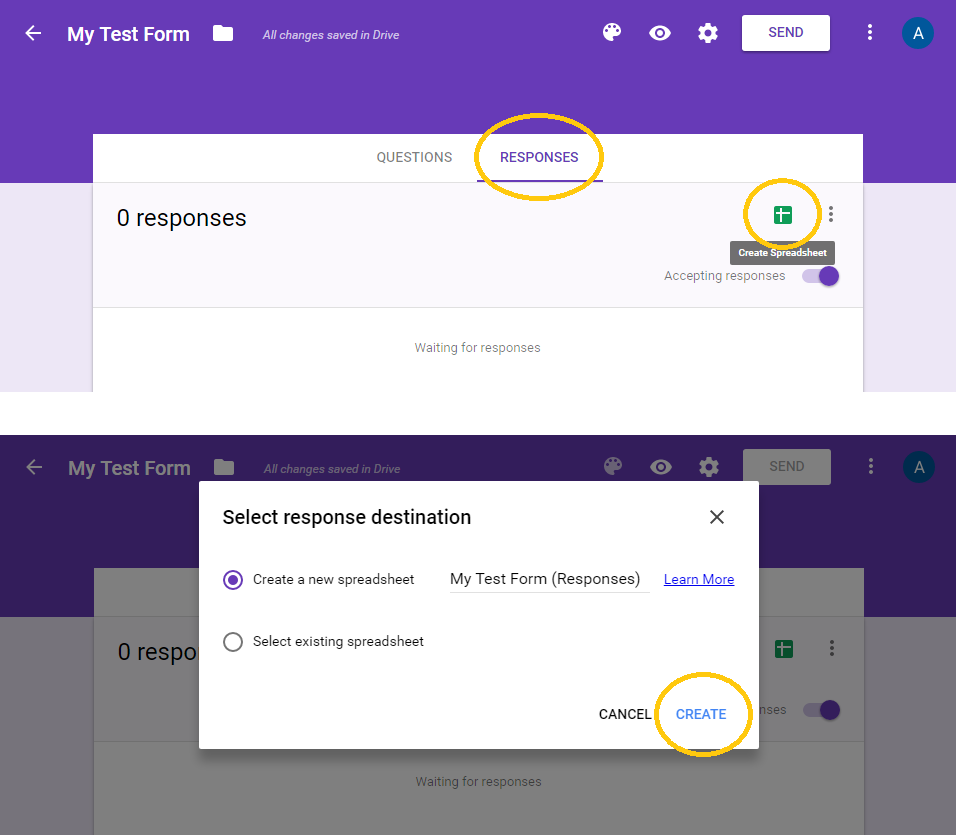
-
You'll be redirected to the new spreadsheet. Note the active sheet name (rename it if you want) and add a header to the column to be used to hold the response edit URLs, i.e. Edit Url
. NOTE: Capitalization is very important, so be very careful on how do you write it.
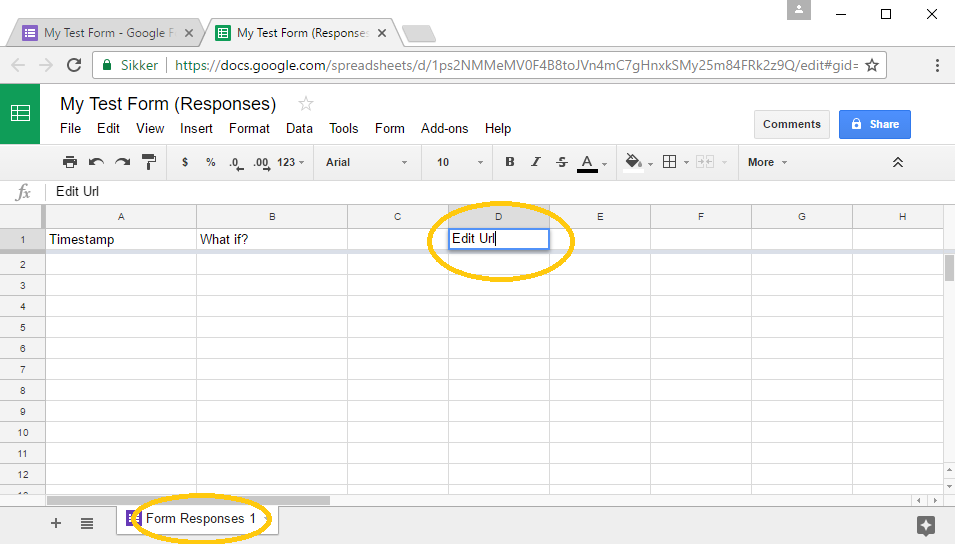
-
Go to Tools > Script editor
to add a Google Apps Script project bounded to the spreadsheet.
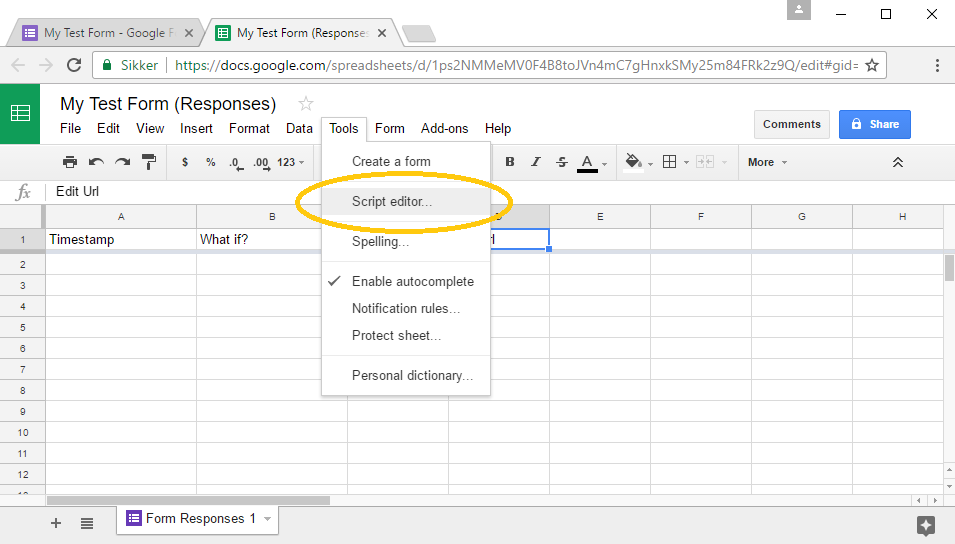
-
Replace the default code with the code below (remember to edit the global variables according to your case).
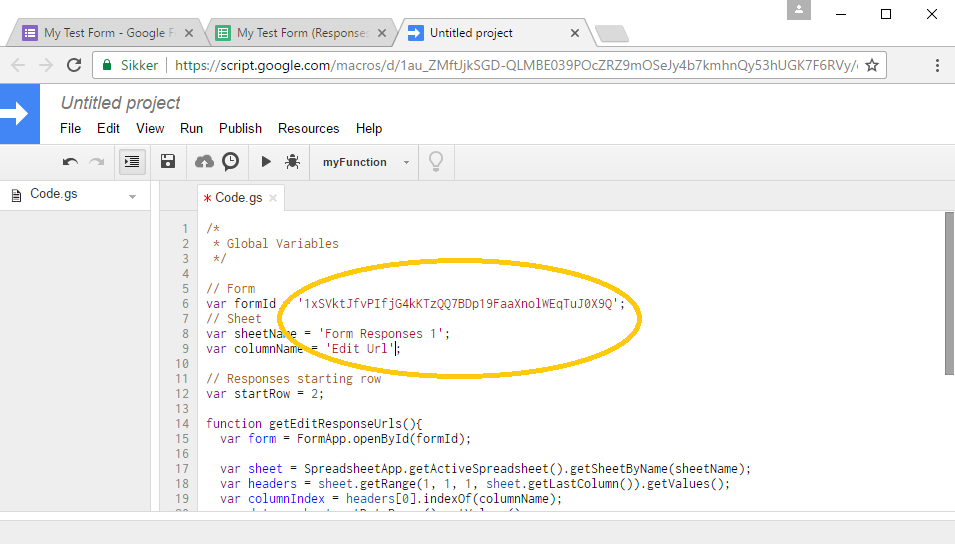
-
Save the project, and then add an installable form summit trigger (if you get an "Authorization required" dialog, click "Review Permissions" and then "Allow").
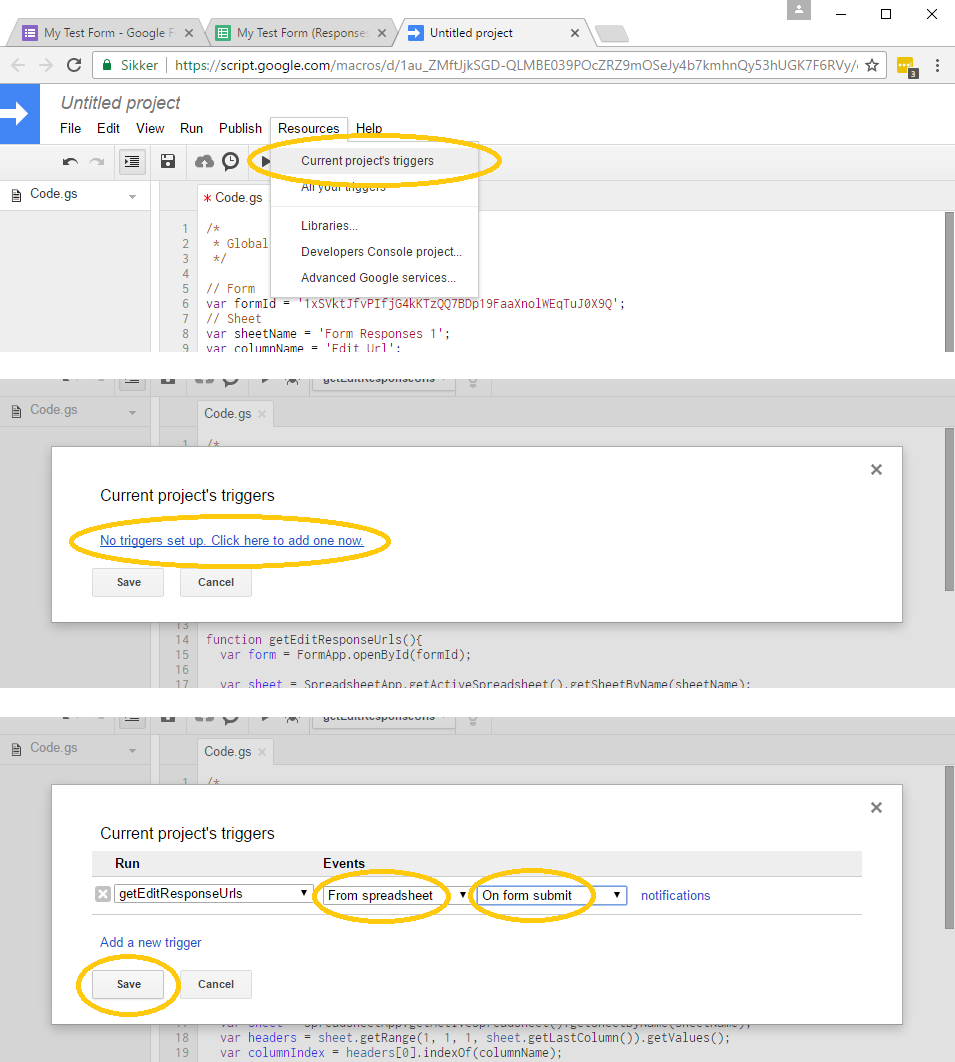
-
Submit some sample responses to test the solution.
#Code
/*
* Global Variables
*/
// Form URL
var formURL = 'https://docs.google.com/forms/d/form-id/viewform';
// Sheet name used as destination of the form responses
var sheetName = 'Form Responses 1';
/*
* Name of the column to be used to hold the response edit URLs
* It should match exactly the header of the related column,
* otherwise it will do nothing.
*/
var columnName = 'Edit Url' ;
// Responses starting row
var startRow = 2;
function getEditResponseUrls(){
var sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(sheetName);
var headers = sheet.getRange(1, 1, 1, sheet.getLastColumn()).getValues();
var columnIndex = headers[0].indexOf(columnName);
var data = sheet.getDataRange().getValues();
var form = FormApp.openByUrl(formURL);
for(var i = startRow-1; i < data.length; i++) {
if(data[i][0] != '' && data[i][columnIndex] == '') {
var timestamp = data[i][0];
var formSubmitted = form.getResponses(timestamp);
if(formSubmitted.length < 1) continue;
var editResponseUrl = formSubmitted[0].getEditResponseUrl();
sheet.getRange(i+1, columnIndex+1).setValue(editResponseUrl);
}
}
}
Alternative code (only small changes):
FormApp.openById()
instead ofFormApp.openByUrl()
, in case Google's URL format changes in the future.data[][]
), in case the value in an empty cell is evernull
orundefined
instead of an empty string._