-
-
Save lancethomps/a5ac103f334b171f70ce2ff983220b4f to your computer and use it in GitHub Desktop.
function run(input, parameters) { | |
const appNames = []; | |
const skipAppNames = []; | |
const verbose = true; | |
const scriptName = "close_notifications_applescript"; | |
const CLEAR_ALL_ACTION = "Clear All"; | |
const CLEAR_ALL_ACTION_TOP = "Clear"; | |
const CLOSE_ACTION = "Close"; | |
const notNull = (val) => { | |
return val !== null && val !== undefined; | |
}; | |
const isNull = (val) => { | |
return !notNull(val); | |
}; | |
const notNullOrEmpty = (val) => { | |
return notNull(val) && val.length > 0; | |
}; | |
const isNullOrEmpty = (val) => { | |
return !notNullOrEmpty(val); | |
}; | |
const isError = (maybeErr) => { | |
return notNull(maybeErr) && (maybeErr instanceof Error || maybeErr.message); | |
}; | |
const systemVersion = () => { | |
return Application("Finder").version().split(".").map(val => parseInt(val)); | |
}; | |
const systemVersionGreaterThanOrEqualTo = (vers) => { | |
return systemVersion()[0] >= vers; | |
}; | |
const isBigSurOrGreater = () => { | |
return systemVersionGreaterThanOrEqualTo(11); | |
}; | |
const V11_OR_GREATER = isBigSurOrGreater(); | |
const V12 = systemVersion()[0] === 12; | |
const APP_NAME_MATCHER_ROLE = V11_OR_GREATER ? "AXStaticText" : "AXImage"; | |
const hasAppNames = notNullOrEmpty(appNames); | |
const hasSkipAppNames = notNullOrEmpty(skipAppNames); | |
const hasAppNameFilters = hasAppNames || hasSkipAppNames; | |
const appNameForLog = hasAppNames ? ` [${appNames.join(",")}]` : ""; | |
const logs = []; | |
const log = (message, ...optionalParams) => { | |
let message_with_prefix = `${new Date().toISOString().replace("Z", "").replace("T", " ")} [${scriptName}]${appNameForLog} ${message}`; | |
console.log(message_with_prefix, optionalParams); | |
logs.push(message_with_prefix); | |
}; | |
const logError = (message, ...optionalParams) => { | |
if (isError(message)) { | |
let err = message; | |
message = `${err}${err.stack ? (" " + err.stack) : ""}`; | |
} | |
log(`ERROR ${message}`, optionalParams); | |
}; | |
const logErrorVerbose = (message, ...optionalParams) => { | |
if (verbose) { | |
logError(message, optionalParams); | |
} | |
}; | |
const logVerbose = (message) => { | |
if (verbose) { | |
log(message); | |
} | |
}; | |
const getLogLines = () => { | |
return logs.join("\n"); | |
}; | |
const getSystemEvents = () => { | |
let systemEvents = Application("System Events"); | |
systemEvents.includeStandardAdditions = true; | |
return systemEvents; | |
}; | |
const getNotificationCenter = () => { | |
try { | |
return getSystemEvents().processes.byName("NotificationCenter"); | |
} catch (err) { | |
logError("Could not get NotificationCenter"); | |
throw err; | |
} | |
}; | |
const getNotificationCenterGroups = (retryOnError = false) => { | |
try { | |
let notificationCenter = getNotificationCenter(); | |
if (notificationCenter.windows.length <= 0) { | |
return []; | |
} | |
if (!V11_OR_GREATER) { | |
return notificationCenter.windows(); | |
} | |
if (V12) { | |
return notificationCenter.windows[0].uiElements[0].uiElements[0].uiElements(); | |
} | |
return notificationCenter.windows[0].uiElements[0].uiElements[0].uiElements[0].uiElements(); | |
} catch (err) { | |
logError("Could not get NotificationCenter groups"); | |
if (retryOnError) { | |
logError(err); | |
log("Retrying getNotificationCenterGroups..."); | |
return getNotificationCenterGroups(false); | |
} else { | |
throw err; | |
} | |
} | |
}; | |
const isClearButton = (description, name) => { | |
return description === "button" && name === CLEAR_ALL_ACTION_TOP; | |
}; | |
const matchesAnyAppNames = (value, checkValues) => { | |
if (isNullOrEmpty(checkValues)) { | |
return false; | |
} | |
let lowerAppName = value.toLowerCase(); | |
for (let checkValue of checkValues) { | |
if (lowerAppName === checkValue.toLowerCase()) { | |
return true; | |
} | |
} | |
return false; | |
}; | |
const matchesAppName = (role, value) => { | |
if (role !== APP_NAME_MATCHER_ROLE) { | |
return false; | |
} | |
if (hasAppNames) { | |
return matchesAnyAppNames(value, appNames); | |
} | |
return !matchesAnyAppNames(value, skipAppNames); | |
}; | |
const notificationGroupMatches = (group) => { | |
try { | |
let description = group.description(); | |
if (V11_OR_GREATER && isClearButton(description, group.name())) { | |
return true; | |
} | |
if (V11_OR_GREATER && description !== "group") { | |
return false; | |
} | |
if (!V11_OR_GREATER) { | |
let matchedAppName = !hasAppNameFilters; | |
if (!matchedAppName) { | |
for (let elem of group.uiElements()) { | |
if (matchesAppName(elem.role(), elem.description())) { | |
matchedAppName = true; | |
break; | |
} | |
} | |
} | |
if (matchedAppName) { | |
return notNull(findCloseActionV10(group, -1)); | |
} | |
return false; | |
} | |
if (!hasAppNameFilters) { | |
return true; | |
} | |
let checkElem = group.uiElements[0]; | |
if (checkElem.value().toLowerCase() === "time sensitive") { | |
checkElem = group.uiElements[1]; | |
} | |
return matchesAppName(checkElem.role(), checkElem.value()); | |
} catch (err) { | |
logErrorVerbose(`Caught error while checking window, window is probably closed: ${err}`); | |
logErrorVerbose(err); | |
} | |
return false; | |
}; | |
const findCloseActionV10 = (group, closedCount) => { | |
try { | |
for (let elem of group.uiElements()) { | |
if (elem.role() === "AXButton" && elem.title() === CLOSE_ACTION) { | |
return elem.actions["AXPress"]; | |
} | |
} | |
} catch (err) { | |
logErrorVerbose(`(group_${closedCount}) Caught error while searching for close action, window is probably closed: ${err}`); | |
logErrorVerbose(err); | |
return null; | |
} | |
log("No close action found for notification"); | |
return null; | |
}; | |
const findCloseAction = (group, closedCount) => { | |
try { | |
if (!V11_OR_GREATER) { | |
return findCloseActionV10(group, closedCount); | |
} | |
let checkForPress = isClearButton(group.description(), group.name()); | |
let clearAllAction; | |
let closeAction; | |
for (let action of group.actions()) { | |
let description = action.description(); | |
if (description === CLEAR_ALL_ACTION) { | |
clearAllAction = action; | |
break; | |
} else if (description === CLOSE_ACTION) { | |
closeAction = action; | |
} else if (checkForPress && description === "press") { | |
clearAllAction = action; | |
break; | |
} | |
} | |
if (notNull(clearAllAction)) { | |
return clearAllAction; | |
} else if (notNull(closeAction)) { | |
return closeAction; | |
} | |
} catch (err) { | |
logErrorVerbose(`(group_${closedCount}) Caught error while searching for close action, window is probably closed: ${err}`); | |
logErrorVerbose(err); | |
return null; | |
} | |
log("No close action found for notification"); | |
return null; | |
}; | |
const closeNextGroup = (groups, closedCount) => { | |
try { | |
for (let group of groups) { | |
if (notificationGroupMatches(group)) { | |
let closeAction = findCloseAction(group, closedCount); | |
if (notNull(closeAction)) { | |
try { | |
closeAction.perform(); | |
return [true, 1]; | |
} catch (err) { | |
logErrorVerbose(`(group_${closedCount}) Caught error while performing close action, window is probably closed: ${err}`); | |
logErrorVerbose(err); | |
} | |
} | |
return [true, 0]; | |
} | |
} | |
return false; | |
} catch (err) { | |
logError("Could not run closeNextGroup"); | |
throw err; | |
} | |
}; | |
try { | |
let groupsCount = getNotificationCenterGroups(true).filter(group => notificationGroupMatches(group)).length; | |
if (groupsCount > 0) { | |
logVerbose(`Closing ${groupsCount}${appNameForLog} notification group${(groupsCount > 1 ? "s" : "")}`); | |
let startTime = new Date().getTime(); | |
let closedCount = 0; | |
let maybeMore = true; | |
let maxAttempts = 2; | |
let attempts = 1; | |
while (maybeMore && ((new Date().getTime() - startTime) <= (1000 * 30))) { | |
try { | |
let closeResult = closeNextGroup(getNotificationCenterGroups(), closedCount); | |
maybeMore = closeResult[0]; | |
if (maybeMore) { | |
closedCount = closedCount + closeResult[1]; | |
} | |
} catch (innerErr) { | |
if (maybeMore && closedCount === 0 && attempts < maxAttempts) { | |
log(`Caught an error before anything closed, trying ${maxAttempts - attempts} more time(s).`) | |
attempts++; | |
} else { | |
throw innerErr; | |
} | |
} | |
} | |
} else { | |
throw Error(`No${appNameForLog} notifications found...`); | |
} | |
} catch (err) { | |
logError(err); | |
logError(err.message); | |
getLogLines(); | |
throw err; | |
} | |
return getLogLines(); | |
} |
@lancethomps Appreciate the response! No worries or priority on getting back to this, real job comes first hah.
For what it's worth even with the widgets removed, the error still persists on 11.3.1. I'm updating to 11.4 now and will report back if it changes. Screenshot attached for reference if it even matters hah.
Edit: Just realized this script pertains to actually closing incoming notifications. My mistake - I was looking for a way to clear all notifications at once in NC and mistook this for that!
Look below.
I just realized that you can also use this to clear notifications inside of the widgets or notification center if you quickly press the widgets button RIGHT after pressing your clear notifications shortcut. Just a quick tip. But it does not work if you are already in notification center or the widgets menu.
This is awesome! Is it possible to modify this to "Mark as Read" a single Mail or Message alert?
@PhilMac I recently created some LaunchBar actions (all based on Applescripts) that allow that. https://github.com/Ptujec/LaunchBar/tree/master/Notifications#readme
It's weird that 'Clear All' isn't an action when notifications are viewed as a list even though there is the clickable 'Clear All' X
on the screen (the 'Clear All' action is only when viewing the single stack, meaning this snippet has to slowly loop through to close them all when viewing the list).
Thank you! I've assigned this to 4 finger FORCE click so I can hit my laptop in rage whenever the "Disk not ejected" alert comes up.
I created an Alfred Workflow based on your script. Works perfectly, tested with macOS Monterey 12.6: https://github.com/bpetrynski/alfred-notification-dismisser
Thank you so much! It works.
@lancethomps seems that it no longer works in macOS Ventura.
[[Run Script](alfredpreferences:workflows%3Eworkflow%3Euser.workflow.45837E05-E293-47B3-AFAA-8873305104C7%3E6D6E67D1-1F17-4129-A457-0D9A41C42E95)] 2022-10-26 10:31:24.806 [close_notifications_applescript] ERROR Error: No notifications found... run@ 2022-10-26 10:31:24.809 [close_notifications_applescript] ERROR No notifications found... /Users/bartosz/Library/Caches/com.runningwithcrayons.Alfred/Workflow Scripts/319418A9-C310-4A9C-ABF4-F54D5188C4DC: execution error: Error: Error: No notifications found... (-2700)
@lancethomps seems that it no longer works in macOS Ventura.
[[Run Script](alfredpreferences:workflows%3Eworkflow%3Euser.workflow.45837E05-E293-47B3-AFAA-8873305104C7%3E6D6E67D1-1F17-4129-A457-0D9A41C42E95)] 2022-10-26 10:31:24.806 [close_notifications_applescript] ERROR Error: No notifications found... run@ 2022-10-26 10:31:24.809 [close_notifications_applescript] ERROR No notifications found... /Users/bartosz/Library/Caches/com.runningwithcrayons.Alfred/Workflow Scripts/319418A9-C310-4A9C-ABF4-F54D5188C4DC: execution error: Error: Error: No notifications found... (-2700)
Same issue after upgrading to Ventura 😭
@lancethomps seems that it no longer works in macOS Ventura.
[[Run Script](alfredpreferences:workflows%3Eworkflow%3Euser.workflow.45837E05-E293-47B3-AFAA-8873305104C7%3E6D6E67D1-1F17-4129-A457-0D9A41C42E95)] 2022-10-26 10:31:24.806 [close_notifications_applescript] ERROR Error: No notifications found... run@ 2022-10-26 10:31:24.809 [close_notifications_applescript] ERROR No notifications found... /Users/bartosz/Library/Caches/com.runningwithcrayons.Alfred/Workflow Scripts/319418A9-C310-4A9C-ABF4-F54D5188C4DC: execution error: Error: Error: No notifications found... (-2700)
Same issue after upgrading to Ventura 😭
I have a similar script for LaunchBar. I updated it to work on Ventura a few days ago. The applescript does not depend on LaunchBar so you can use it in Alfred, Shortcuts or on its own. https://github.com/Ptujec/LaunchBar/blob/master/Notifications/Dismiss%20all%20notifications.lbaction/Contents/Scripts/default.applescript
@Ptujec it works well, however it has limited support for os languages.
@Ptujec it works well, however it has limited support for os languages.
Correct. Unfortunately I haven't found a way to support all possible languages programatically.
If you want to add your language you can use this script to find out the exact wording for your UI language. It will copy all possible action names to your clipboard. Obviously there needs to be a notification for that to work. Than just add the relevant names like "close" and "clear all" in your language in line 10 of the script I shared before.
tell application "System Events"
try
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
set _descriptions to {}
repeat with _group in _groups
set _actions to actions of _group
repeat with _action in _actions
set end of _descriptions to description of _action & ", "
end repeat
end repeat
end try
set the clipboard to _descriptions as text
end tell
Here is a script you can use to create test notifications:
display notification "Notification" with title "Title"
How could one get the notification title here?
I only want to dismiss notifications with specific title
Sorry for the tardiness in replies to the comments about this not working on Ventura - I hadn't upgraded until last week and didn't have a good environment to test Ventura support on. It should be fixed now.
How could one get the notification title here? I only want to dismiss notifications with specific title
@rosenpin update the appNames
parameter on line 3. I haven't been using that lately so I'm not 100% sure it still works on Ventura, but let me know if it doesn't work. If you're looking to do something other than close all notifications for a specific application, that isn't supported at the moment
How could one get the notification title here?
I only want to dismiss notifications with specific title
Not sure how it would look like in JXA. But this should work in standard applescript:
tell application "System Events"
-- Replace the text in quotations with the expected title you want to close
set _close_text to "YOUR CLOSE TEXT"
set _result to {}
try
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
repeat with _group in _groups
-- Convert static text of group to a string
set _notification_title to value of item 1 of static text of _group
-- Compare close text with the title … if they match close
if _notification_title is _close_text then
set _actions to actions of _group
repeat with _action in _actions
if description of _action is in {"Schließen", "Alle entfernen", "Close", "Clear All"} then
perform _action
end if
end repeat
end if
end repeat
end try
end tell
Is anyone using this on macOS Ventura 13.3? I tried it but it didn't work.
I slightly modified the above script to work on macOS Ventura 13.3, Unfortunately this won't clear all notification just one at a time.
tell application "System Events"
try
click menu bar item 1 of menu bar 1 of application process "Control Center"
end try
try
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
repeat with _group in _groups
set _actions to actions of _group
repeat with _action in _actions
if description of _action is in {"Schlie§en", "Alle entfernen", "Close", "Clear All"} then
perform _action
end if
end repeat
end repeat
end try
key code 53 # Escape Key
end tell
The script is working in Ventura, if running from automator. Oddly, running from finder gives an error, but running from other apps (like chrome) works just fine. Is there some way to see the logs when running the action from services and not in automator directly?
Has anyone been able to get this to work within BetterTouchTool (BTT) and if so, what did you do? I am having trouble getting it to fire and unsure if I'm tripping up on something due to BTT or something else... thanks in advance!
Sorry for the tardiness in replies to the comments about this not working on Ventura - I hadn't upgraded until last week and didn't have a good environment to test Ventura support on. It should be fixed now.
Thank you Lance! The latest code works well for Ventura.
I managed to close all notification for a single group with one action.
I'm using macOS Ventura 13.4 and upgraded code from @alwinsamson.
- I deleted first try block because it always raise error in my case.
- if not working, follow the guide in comment please.
tell application "System Events"
try
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
repeat with _group in _groups
set _actions to actions of _group
repeat with _action in _actions
-- NOTE: Other actions are also usable
-- like "View", "Close" (only one notification)
-- If not working, find the correct
-- description field value 'Response' tab in Script Editor
if description of _action is in {"Close All", "모두 지우기"} then
perform _action
end if
end repeat
-- If there's only one notification for the top group,
-- "Close All" would not exist
repeat with _action in _actions
if description of _action is in {"Close", "닫기"} then
perform _action
end if
end repeat
end repeat
end try
key code 53 # Escape Key
end tell
Thank you @roeniss! Working [mostly] on my M1 Pro Ventura 13.4.1 (22F82)
It reliably removes the notifications from my screen, but only dismisses some of the notifications from the Notifications Center.
--- UPDATE 2023-09-14 ---
@dtyuan saved the day! "Clear" and "Clear All" worked for me! Thanks! (though I have to run it multiple times and seems less reliable if I have the notification center already displayed)
Thank you @roeniss.
Changing from "Close All"
to "Clear All"
seems to work for me. (Ventura 13.5.1)
The last version, published by @roeniss, sometimes doesn't work when I have multiple notification groups, each one having multiple notifications. Only some notification groups are closed but the rest remain open and I have to run the script multiple times to get rid of all of them. I have many applications with notifications configured in Alert mode (not Banner) so this situation happens quiet frequently for me.
I think (but can't prove it) that closing a notification group somehow interferes with iteration over remaining groups and therefore this script finishes early. So I tried to modify it so that iteration on groups restarts every time notification is closed.
tell application "System Events"
try
repeat
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
set numGroups to number of _groups
if numGroups = 0 then
exit repeat
end if
repeat with _group in _groups
set _actions to actions of _group
set actionPerformed to false
repeat with _action in _actions
if description of _action is in {"Clear All", "Close"} then
perform _action
set actionPerformed to true
exit repeat
end if
end repeat
if actionPerformed then
exit repeat
end if
end repeat
end repeat
end try
end tell
This version works much better for me. Try it if you experience similar problems.
also works great 👍 Thanks!
Just FYI, to me, separating and doing "Cleare All" first then "Close" works better.
tell application "System Events"
try
repeat
set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter"
set numGroups to number of _groups
if numGroups = 0 then
exit repeat
end if
repeat with _group in _groups
set _actions to actions of _group
set actionPerformed to false
repeat with _action in _actions
if description of _action is in {"Clear All"} then
perform _action
set actionPerformed to true
exit repeat
end if
end repeat
repeat with _action in _actions
if description of _action is in {"Close"} then
perform _action
set actionPerformed to true
exit repeat
end if
end repeat
if actionPerformed then
exit repeat
end if
end repeat
end repeat
end try
end tell
Just FYI, to me, separating and doing "Cleare All" first then "Close" works better.
That's strange, semantically there shouldn't be any difference - once you act "Clear All"/Close on group or notification, it will be dismissed immediately and there's no point in running the other action on it....
Anyway, I do anticipate weird behaviour because of bugs in all this applescript/accessibility feature of MacOS - and I'm sure there are many. After several more days of usage I do see that even my solution doesn't clear all the notifications if there are many (>5) notifications/groups present. I'm currently experimenting with putting delays between loop iterations in various places, may be that will help.
I wish I could understand what's going on here. I'm on Monterey but not a single script on this page works. Most do absolutely nothing at all.
The OP's gist, saved as a javascript AS application and granted the accessibility permissions, is incredibly slow, waits a long time before very slowly closing a small fraction of my notifications, not all of them, and then simply quits. I've run it three times now and I still haven't gotten rid of all my notifications.
The rest of the code samples on this page do nothing at all on my machine, except for @Ptujec's, which aborts with error "The variable _descriptions is not defined." number -2753 from "_descriptions"
even though I do have notifications showing. The rest of them, I run them, they run, and I still have notifications showing, nothing at all happened.
I wish I could understand what's going on here. I'm on Monterey but not a single script on this page works. Most do absolutely nothing at all.
The OP's gist, saved as a javascript AS application and granted the accessibility permissions, is incredibly slow, waits a long time before very slowly closing a small fraction of my notifications, not all of them, and then simply quits. I've run it three times now and I still haven't gotten rid of all my notifications.
The rest of the code samples on this page do nothing at all on my machine, except for @Ptujec's, which aborts with
error "The variable _descriptions is not defined." number -2753 from "_descriptions"
even though I do have notifications showing. The rest of them, I run them, they run, and I still have notifications showing, nothing at all happened.
Scripts that involve GUI scripting are prone to break with new OS versions. Not sure to which version of my script you are referring but this one should work under Monterey
Has anyone been able to get this to work within BetterTouchTool (BTT) and if so, what did you do? I am having trouble getting it to fire and unsure if I'm tripping up on something due to BTT or something else... thanks in advance!
FYI: BetterTouchTool offers this feature built-in: Close All Notification Alerts
, for those who would prefer a paid solution: https://community.folivora.ai/t/clear-notifications-with-a-keyboard-shortcut/30335
(I already use BetterTouchTool for dozens of other use cases, so I already had it.)
(and if you're wondering how I came across this thread: I didn't realize BTT already offered this feature until today...)
Does this script still work with Sonoma?
I've been trying this script out as an Alfred workflow (courtesy of https://github.com/bpetrynski/alfred-notification-dismisser), and it doesn't seem like the script is doing anything.
I created an Alfred Workflow based on your script. Works perfectly, tested with macOS Monterey 12.6: https://github.com/bpetrynski/alfred-notification-dismisser
OMG Thank YOU!!!! Been needing this for a long time. :-)
Just FYI, to me, separating and doing "Cleare All" first then "Close" works better.
tell application "System Events" try repeat set _groups to groups of UI element 1 of scroll area 1 of group 1 of window "Notification Center" of application process "NotificationCenter" set numGroups to number of _groups if numGroups = 0 then exit repeat end if repeat with _group in _groups set _actions to actions of _group set actionPerformed to false repeat with _action in _actions if description of _action is in {"Clear All"} then perform _action set actionPerformed to true exit repeat end if end repeat repeat with _action in _actions if description of _action is in {"Close"} then perform _action set actionPerformed to true exit repeat end if end repeat if actionPerformed then exit repeat end if end repeat end repeat end try end tell
I'm getting the following error on macOS Sonoma 14.3.
I have resolved the issue. If someone is having this notification, this is because this has to be a javascript bundled application file, not an applescript bundled application. You have to add this as javascript for some strange reason.
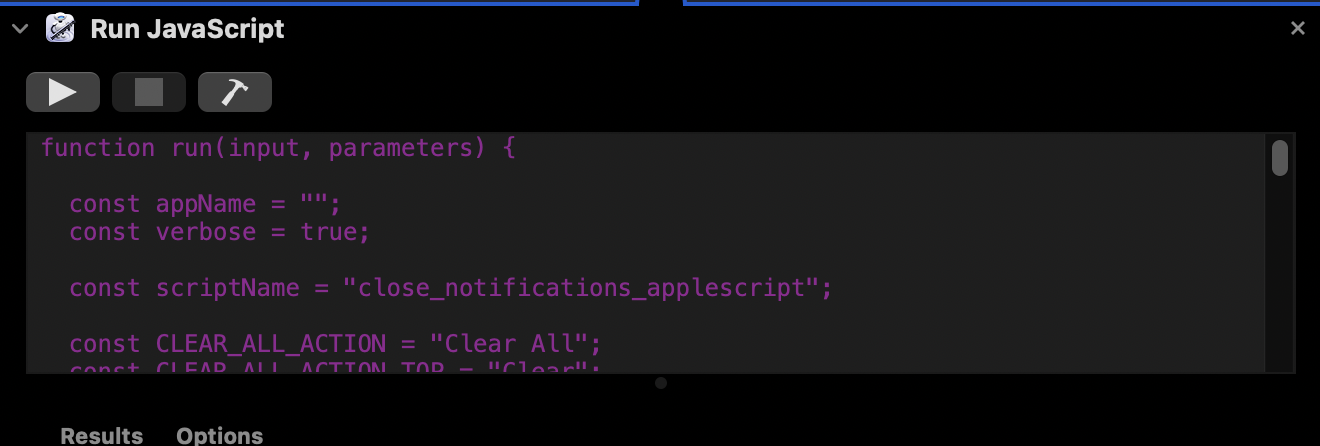
Also, I think that the creator should create a formal page for how to use this instead of leaving it like this.